Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial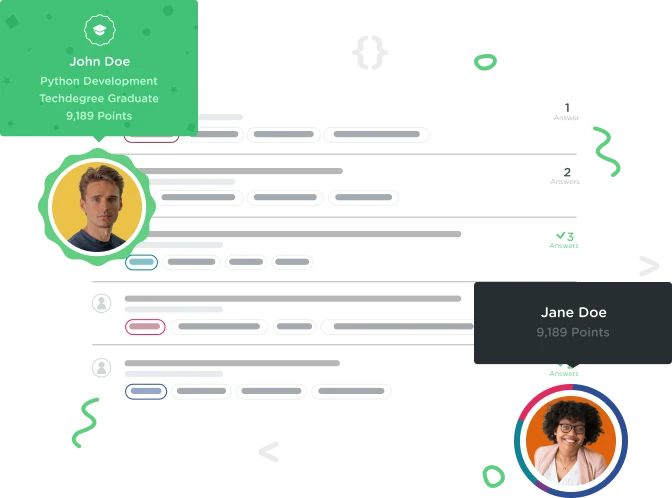

Suraj Shah
1,055 PointsLoop Challenge
I need to loop through items.
If item begins with 'a' then skip else print the item
Not sure why my code is not passing the challenge. Any help would be greatly appreciated!
Thanks
def loopy(items):
for item in items:
try:
if item.index('a') == 0:
except[ValueError]:
continue
else:
print(item)
2 Answers
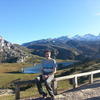
Nick Osborne
4,612 PointsI think the problem is that your if will only return True or False rather than a ValueError. Something like this is more simple:
def loopy(items):
for item in items:
if item[0]=="a":
continue
else:
print(item)

Andy Hughes
10,049 PointsI've just done this challenge myself and as usual, over complicated it. This first part is straight forward i.e. print the items. So we have our function:
def loopy(items):
and we need the for statement to print "items"
def loopy(items):
for item in items:
print(items)
This completes the first part of the challenge. The next part is then to add in the 'break' based on the condition 'STOP' being in the 'items' list. If 'STOP' is not present, we need to then print(items). So the first part needs the 'if' statement to catch 'STOP'.
def loopy(items):
for item in items:
if items == "STOP":
break
This gives us our requirement for the 'Break' in the challenge. The last part is to continue with the 'for loop' if 'STOP' is not present and print the items in the list.
def loopy(items):
# Code goes here
for item in items:
if items == "STOP":
break
else:
print(item)
I spent a good few hours trying various combinations of 'if ==' and 'if !=' and started getting way too complicated. In the end I rewatched the videos, looked up the code syntax example in python manual and then stripped it back.
Hope it helps anyone else stuck here. :)