Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial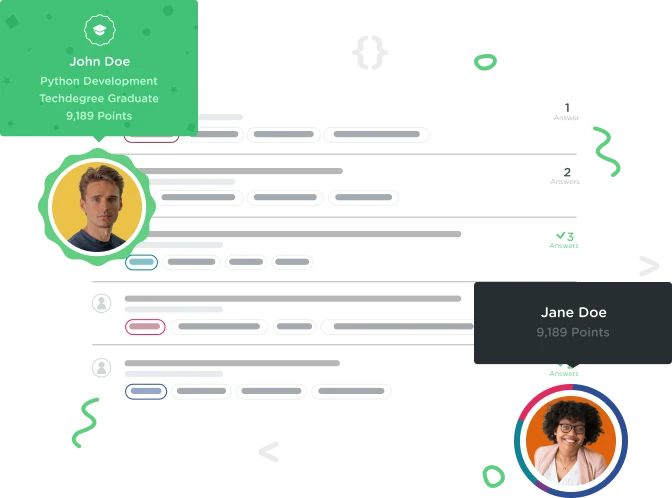
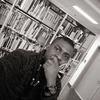
luckychukwuladimasi
Courses Plus Student 2,282 Pointslooping through Arrays.
I am finding it difficult making progress... Any one knows why this lines of code wouldn't pass this challenge?
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i <8; i+=1) {
console.log( tempeatures [0]);
console.log( tempeatures [1]);
console.log( tempeatures [2]);
console.log( tempeatures [3]);
console.log( tempeatures [4]);
console.log( tempeatures [5]);
console.log( tempeatures [6]);
console.log( tempeatures [7]);
}
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i <8; i+=1) {
console.log( tempeatures [0]);
console.log( tempeatures [1]);
console.log( tempeatures [2]);
console.log( tempeatures [3]);
console.log( tempeatures [4]);
console.log( tempeatures [5]);
console.log( tempeatures [6]);
console.log( tempeatures [7]);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
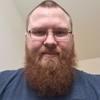
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsUsing loops allows you to type less line of code. Try something like this:
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i+=1) {
console.log( tempeatures [i]);
// [i] will first time in loop be [0] then [1] then [2] ....
// this should save you from typing all those lines
}

Brandon Benefield
7,739 PointsYour code is logging the ENTIRE array to the console for EACH iteration. What you need to do is reference your var i = 0
variable (or iterator in this case).
var temperatures = [100, 90, 99, 80, 70, 65, 30, 10];
for (var i = 0; i < temperatures.length; i++) {
console.log(temperatures[i]);
}
So in var i = 0
in this case, i
is going to be your indexer for the array. If you recall, we can access any item inside of the array with a number i.e. console.log(temperatures[0])
will log 100
to your console. So on and so forth. So as i
increases in number (thats what the i++
is doing after each iteration through the for
loop) i
using its value (starting at 0) and acting as a number.
Now we use temperature.length
for the most part to iterate through arrays, because for one, it's easier than counting our arrays (imagine if it were 1000 indexes long) and sometimes we do not even know what is inside of the array. It could be one item, it could be a million.
I hope this helps.

Ricardo Aguirre
6,344 PointsYou are declaring an array in var temperatures and you are calling tempeatures.