Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial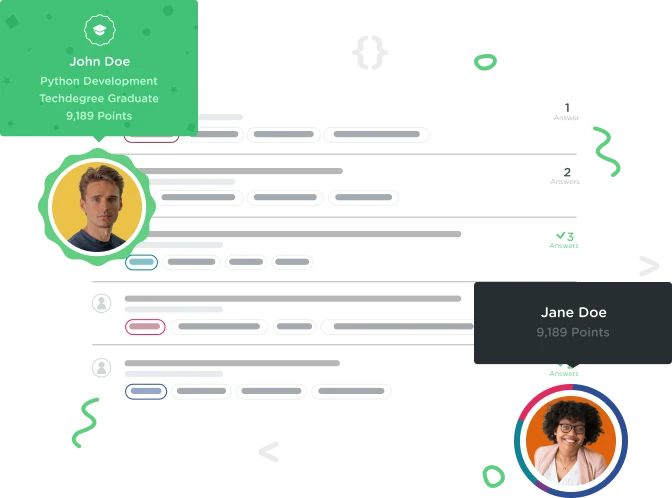

Tony Brackins
28,766 PointsLost
I got lost here. Creating random numbers between a users input. Should I keep going to conditional statements?
1 Answer

Nega Lulessa
15,669 PointsTony,
If you can, kindly also post a snippet of the code you are having the issue with so others can take a look and may be suggest a possible solution. That said, I am going to take a stab in the dark here and hope the following would be of any help to you.
Let's assume you want to generate a random number between 0 and a number the user has supplied. You can do this by using JavaScript's Math object, more specifically, the Math object's function or method called random(). This method generates a random number between 0 and 1. Here is one way to go about it:
var userNumber; //Say you have stored the user's number in this variable
var randomNumber; //Declare a variable to hold the random number you seek
//call the Math.random() method multiplying it by the value stored in userNumber-1 as follows:
randomNumber = Math.ceil(Math.random() * (userNumber -1));
Here is what is happenning here:
Since Math.random() only returns a number between 0 and 1 (say, 0.092456), multiplying it by the userNumber -1 returns a value between 0 and one less than the user's number with some decimals in it. When you call the Math.ceil() method on that result, you will get a number rounded up to the nearest integer. (Of course, you could also use Math.round(), or Math.floor() with the necessary tweaks). This returned number is then stored in the randomNumber variable. When you inspect randomNumber, you will find that it is an integer anywhere between 0 and the userNumber without the decimals.
Below, i have constructed a simple function named randomNumber that uses this very same code. It takes a single argument (in this case, the user's number or whatever is captured in the variable userNumber). When called with the proper argument supplied, the function dynamically spits out a random number between 0 and the user's number. Have a look:
var userNumber; //The same as above, grab the user's number and store it in here.
//Declare a function called randomNumber that takes a single argument:
var randomNumber = function(userNumber) {
//Do the computations, as above, and return the generated random number:
return Math.ceil(Math.random() * (userNumber-1)); //The same code from above
}
Suppose the number the user supplied was 98. You would then call this function like so:
randomNumber(98);
15 // This is what i got on my console. Expect a different result on your side...
Now, if you wish, you can also store the return value of this function into another variable and do whatever you want with it. Storing the return of the function call into a variable could take the following form:
var anotherRandNum = randomNumber(76); //Tired of this 98...
//Now you can deploy anotherRandNum to some good use:
console.log(anotherRandNum + " bottles of beer on the wall, take one down and...");
Hope this has been helpful.
Do shout back with corrections, beers etc etc....
Cheers!
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherTony;
Where are you lost? Let's see if we can help you out.
Ken