Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial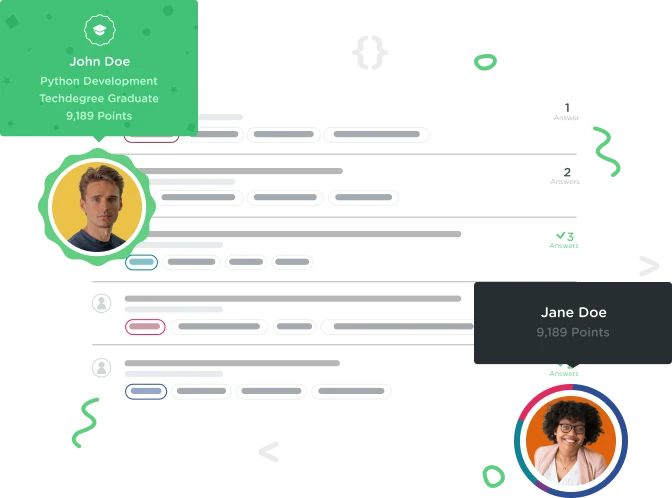

Trevor Byrom
720 PointsMake a while loop that runs until start is falsey.
What am I doing wrong in my code? Everything is fine until I submit step 3 when it says task 1 fails. Pointers please?
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start = True:
num = random.randint(1, 99)
if even_odd != 0:
print("{} is odd".format(num))
else:
print("{} is even".format(num))
start -= 1
2 Answers
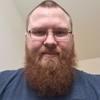
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsThere is a couple of mistakes:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start: # by saying 'start' python knows it means 'as long as start == True'
ran_num = random.randint(1, 99)
if even_odd(ran_num): # even_odd is a function
print("{} is even".format(ran_num))
else:
print("{} is odd".format(ran_num))
start -= 1
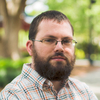
Kenneth Love
Treehouse Guest TeacherWow, took me awhile to find the bug in the challenge. Turns out I was correctly testing the output...but only the last line. So if your last line was correct, the challenge would pass (this is why the if even_odd != 0:
was passing sometimes).
Trevor Byrom
720 PointsTrevor Byrom
720 PointsPerfect. Thank you so much Henrik!
Steven Parker
231,248 PointsSteven Parker
231,248 PointsGood catch! I didn't notice the function error because the other code actually passed the challenge.
Clearly it should not have. You might want to report it as a bug to Support.
And while your example shows that you can check if start is "truthy" just by naming it, since it is a number it will never actually be equal to True.
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsHenrik Christensen
Python Web Development Techdegree Student 38,322 Pointssince it is a number it will never actually be equal to True. - I've always thought that is was True as long it was > 0? :-p
EDIT: Just sent a mail to support about this bug.
Moderator edit: The problem is that you can pass the challenge by saying:
if even_odd != 0: rest of the code here
but that shouldn't be possible since even_odd is a function.
Edited to include information from help ticket JP
Steven Parker
231,248 PointsSteven Parker
231,248 PointsThere's a difference between True and "truthy". Try this:
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsHenrik Christensen
Python Web Development Techdegree Student 38,322 PointsNice example to explain difference between True and truthy :-)