Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial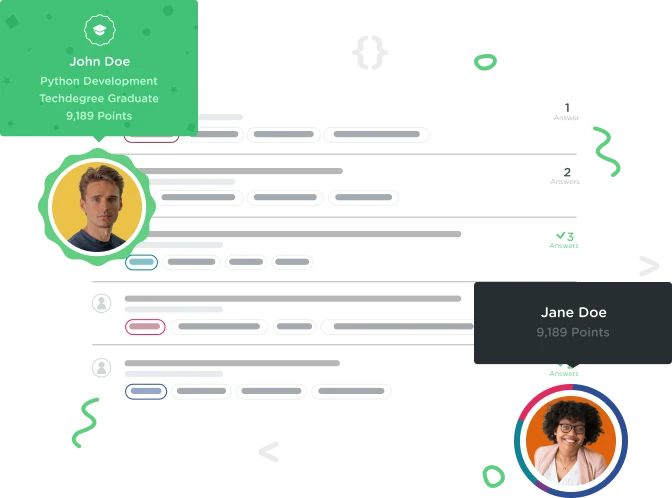

nicole godoy
945 PointsMake sure you're not using the memberwise initializer provided by default?
I'm getting this error even though I assigned initial values to each stored property. I'm not sure why It is still using memberwise initializer by default.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init( red: Double, green: Double, blue: Double, alpha: Double, description: String) {
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
self.description = ("red: \(self.red), green: \(self.green), blue: \(self.blue), alpha: \(self.alpha)")
}
}
3 Answers
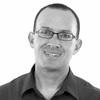
David Papandrew
8,386 PointsHi Nicole,
You want to assign the custom init parameters to the struct's stored properties
Also, "description" should not be one of the custom initializer parameters.
Here's the corrected code:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(self.red), green: \(self.green), blue: \(self.blue), alpha: \(self.alpha)"
}
}
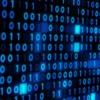
Alexander Davison
65,469 PointsDon't worry about getting this one wrong; I in fact got a little confused in this challenge earlier today.
The only problems are that:
- You should take in a description as an argument to the
init
method. - You should set
self.red
to the argumentred
,self.blue
to the argumentblue
, etc. Don't set them to a fixed value! - I'm not sure if this is causing an error, but if still aren't passing, you should try to remove parentheses around
description
if you still aren't passing.
I hope this helps. ~Alex

Jon Barnett
2,004 PointsI think the course compiler is throwing errors here where there may be none. The task says this "Using the initializer assign values to the first four properties." Ok so there is an example of this in the Apple docs as follows- ---------------------------------------------quote begins The example below defines a new structure called Fahrenheit to store temperatures expressed in the Fahrenheit scale. The Fahrenheit structure has one stored property, temperature, which is of type Double:
struct Fahrenheit {
var temperature: Double
init() {
temperature = 32.0
}
}
var f = Fahrenheit()
print("The default temperature is \(f.temperature)° Fahrenheit")
// Prints "The default temperature is 32.0° Fahrenheit"
The structure defines a single initializer, init, with no parameters, which initializes the stored temperature with a value of 32.0 (the freezing point of water in degrees Fahrenheit).
Default Property Values You can set the initial value of a stored property from within an initializer, as shown above. Alternatively, specify a default property value as part of the property’s declaration. You specify a default property value by assigning an initial value to the property when it is defined. ----------------------------------------------quote ends
All pretty clear. So here is the solution
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init() {
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
self.description = "red: \(self.red), green: \(self.green), blue: \(self.blue), alpha: \(self.alpha)"
}
}
Note the empty brackets as part of the init declaration, following the Apple example. My Solution throws an error in the Treehouse task- but not in the preview section. Also if you test it in Xcode with the following
let test = RGBColor()
test.red
test.description
You can go on to test all the values. All as they should be. No errors in Xcode.
ie create an instance called test, then check that the required default values have been set using dot syntax, Xcode then displays the correct and required values on the right.
Ok happy to be corrected, but I have googled the crap out of this and I think this is it. Further- the code is not really displaying correctly here for some Treehousey reason. Pasan- great teacher and communicator, please check this.
nicole godoy
945 Pointsnicole godoy
945 PointsThank you so much!