Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial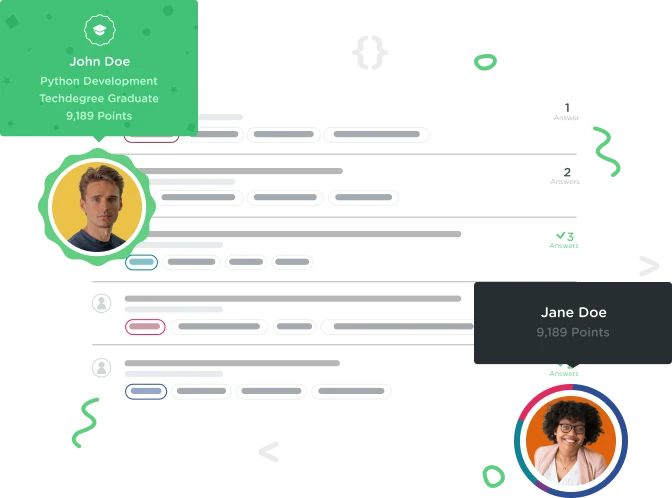

Gus Feliciano
Front End Web Development Techdegree Student 5,089 PointsMethod Confusion in Ruby
I am confused on a certain part of my code while doing the Ruby Track on Building a Address Book.
Why is it that after I define a method for "last_first" for instance I need to type last_first after I end the if statement? Just don't seem to understand it.
Thanks!
class Contact
attr_writer :first_name, :middle_name, :last_name
def first_name
@first_name
end
def middle_name
@middle_name
end
def last_name
@last_name
end
def first_last
first_name + " " + last_name
end
def last_first
last_first = last_name
last_first += ", "
last_first += first_name
if !@middle_name.nil?
last_first += " "
last_first += middle_name.slice(0, 1)
last_first += "."
end
last_first
end
def full_name
full_name = first_name
if !@middle_name.nil?
full_name += " "
full_name += middle_name
end
full_name += ' '
full_name += last_name
full_name
end
def to_s(format = 'full_name')
case format
when 'full_name'
full_name
when 'last_first'
last_first
when 'first'
first_name
when 'last'
last_name
else
first_last
end
end
end
jason = Contact.new
jason.first_name = "Jason"
jason.last_name = "Seifer"
puts jason.to_s
puts jason.to_s('full_name')
puts jason.to_s('last_first')
nick = Contact.new
nick.first_name = "Nick"
nick.middle_name = "A"
nick.last_name = "Pettit"
puts nick.to_s('first_last')
1 Answer
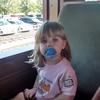
Patrick Hager
15,539 PointsAs I understand it, the reason you put "last_first" (and "full_name") is to explicitly return the contents of the variable when the method is called, in this case in your to_s method.
Hope that helps to clear it up a bit.