Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial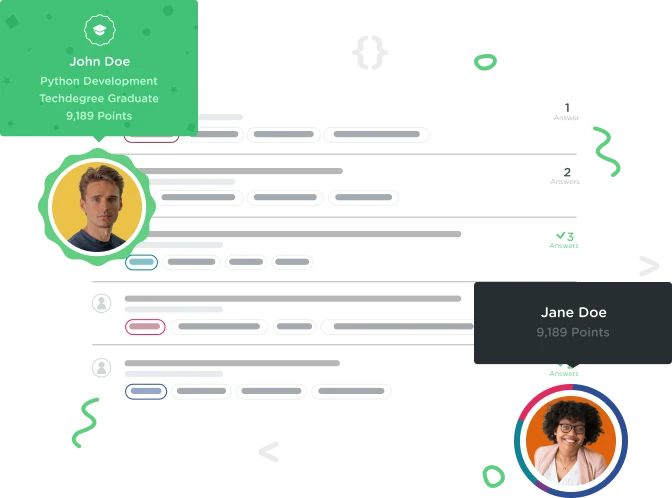
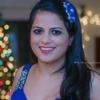
Mona Jalal
4,302 Pointsmost_classes() returns the wrong teacher
I passed it first but in the new challenge the method most_classes() is not passing now:
def num_teachers(dict): return len(dict)
def most_classes(dict): for item in dict: count = 0 max = 0 teacher = None for course in dict[item]: count += 1 if count > max: max = count teacher = item return teacher
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def num_teachers(dict):
return len(dict)
def most_classes(dict):
for item in dict:
count = 0
max = 0
teacher = None
for course in dict[item]:
count += 1
if count > max:
max = count
teacher = item
return teacher
7 Answers
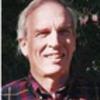
jcorum
71,830 PointsMona, some folk think we shouldn't provide real answers, but only hints, but I'm of the opinion that you learn better if you don't get too frustrated, and can see the answer once you've tried and it hasn't worked. At least that's been my experience.
If you are of a different persuasion about this then don't read on! In other words, spoiler alert.
def most_classes(teacher_dict):
max_count = 0
teacher = ''
for key in teacher_dict:
value = teacher_dict[key]
if len(value) > max_count:
max_count = len(value)
teacher = key
return teacher
def num_teachers(teacher_dict):
teacher_count = 0
for key in teacher_dict:
teacher_count += 1
return teacher_count
def stats(teacher_dict):
teacher_count_list = []
for key, value in teacher_dict.items():
newItem = [key, int(len(value))]
teacher_count_list.append(newItem)
return teacher_count_list
def courses(teacher_dict):
course_list = []
for key, value in teacher_dict.items():
new_list = value;
for newItem in new_list:
course_list.append(newItem)
return course_list
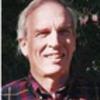
jcorum
71,830 PointsYou enter a blank line, then you hit the accent grave key 3 times ``` and the type of code, so you'd put python, then the code on the next line(s), followed by a line with 3 more accent graves ``` and then a blank line.
def most_classes(dict):
max=0
teacher=None
for key in dict:
if len(dict[key]) > max:
max=len(dict[key])
teacher=key
return teacher
There are more instructions in the Markdown Cheatsheet below, but that's the main part for including code.
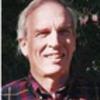
jcorum
71,830 PointsThere's a question on StackOverflow about whether Python has generics like Java, and here's part of the answer:
"No. Python is not a statically typed language, so there is no need for them. Java generics only provide compile time type safety; they don't do anything at runtime. Python doesn't have any compile time type safety, so it wouldn't make sense to add generics to beef up the compile time type checks Python doesn't have.
A list, for example, is an untyped collection. There is no equivalent of distinguishing between List<Integer> and List<String> because a Python list can store any type of object."
http://stackoverflow.com/questions/20449398/does-python-have-generic-methods
But that's not quite the right answer. See: https://www.python.org/dev/peps/pep-0484/#generics
However, that's way beyond the Python track.
Here are some examples of how to create and work with lists:
a_list = list(range(10))
a_list = a_list + [10, 11, 12]
a_list.append([13, 14, 15])
//a_list will be [1...9, 10, 11, 12, [13, 14, 15])
list_1 = [1,2,3]
list_2 = [4,5,6]
list_3 = list_1 + list_2
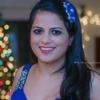
Mona Jalal
4,302 Pointsfor num_teachers you could only use def num_teachers(dict): return len(dict) jcorum
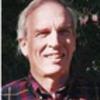
jcorum
71,830 PointsHey, that's right. Someone else asked how to do it in a loop (for practice) and I forgot I'd changed it. Good eye.
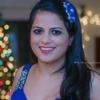
Mona Jalal
4,302 PointsAlso I solved it this way too: How should I select something as code? jcorum
python
def most_classes(dict):
max=0
teacher=None
for key in dict:
if len(dict[key]) > max:
max=len(dict[key])
teacher=key
return teacher
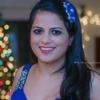
Mona Jalal
4,302 Pointsok thank you so much. I don't know how to create a separate thread so I ask it here as googling didn't help. How can I create a list of list in Python and assign a value to it? in Java: jcorum
List<List<Integer>> list = new ArrayList<>();
list.get(0).add(1);