Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial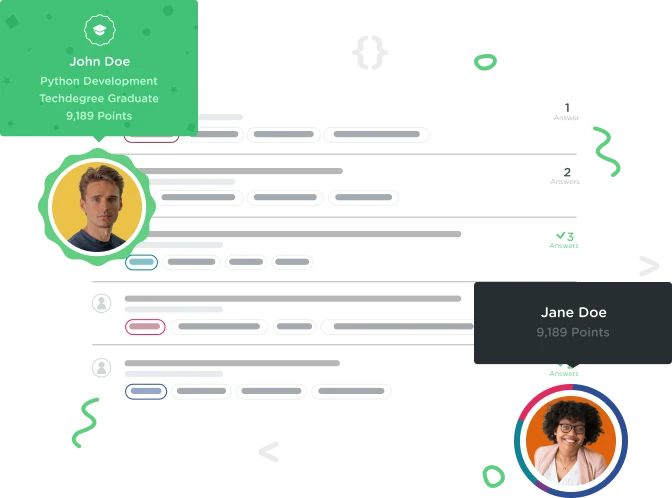

Binu David
Front End Web Development Techdegree Student 11,990 Pointsmost_courses - code not completing the challenge as expected. However, in testing it did produce what I was expecting..
def most_courses(dict): max_count = 0 teacher_list = []
Running through each teacher and course in the dict
for teacher in dict:
print("This is the object type for teacher: ")
print(type(dict[teacher]))
Verifying the course(s) are in a list
if isinstance(dict[teacher], list):
print("This is how many classes the teacher has: ")
print(len(dict[teacher]))
# Checking current highest count against each item
if max_count < (len(dict[teacher])):
max_count = len(dict[teacher])
teacher_list = []
teacher_list.extend(teacher.split(','))
print(teacher_list)
elif (max_count == len(dict[teacher])):
teacher_list.extend(teacher.split(','))
print(teacher_list)
Verifying the course(s) may be a string
elif isinstance(dict[teacher], str):
print(1)
if max_count < 1:
max_count = 1
teacher_list.extend(teacher.split(','))
#print("Total count is:", max_count)
#print ("The completed teacher's list shows: ", teacher_list)
return teacher_list
most_courses(teachers)
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dict):
return (len(dict.keys()))
def num_courses(dict):
count = 0
for courses in dict:
# print(type(dict[courses]))
if isinstance(dict[courses], list):
# print(len(dict[courses]))
count += len(dict[courses])
elif isinstance(dict[courses], str):
# print(1)
count += 1
# print("Total count is:", count)
return count
def courses(dict):
course_list = []
for course in dict:
if isinstance(dict[course], list):
course_list.extend(dict[course])
# print (course_list)
elif isinstance(dict[course], str):
course_list.extend(dict[course].split(","))
# print(course_list)
return course_list
def most_courses(dict):
max_count = 0
teacher_list = []
# Running through each teacher and course in the dict
for teacher in dict:
print("This is the object type for teacher: ")
print(type(dict[teacher]))
# Verifying the course(s) are in a list
if isinstance(dict[teacher], list):
print("This is how many classes the teacher has: ")
print(len(dict[teacher]))
# Checking current highest count against each item
if max_count < (len(dict[teacher])):
max_count = len(dict[teacher])
teacher_list = []
teacher_list.extend(teacher.split(','))
print(teacher_list)
elif (max_count == len(dict[teacher])):
teacher_list.extend(teacher.split(','))
print(teacher_list)
# Verifying the course(s) may be a string
elif isinstance(dict[teacher], str):
print(1)
if max_count < 1:
max_count = 1
teacher_list.extend(teacher.split(','))
#print("Total count is:", max_count)
#print ("The completed teacher's list shows: ", teacher_list)
return teacher_list
1 Answer
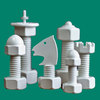
Steven Parker
241,957 PointsYou may have misunderstood the objective, which is always a risk when testing outside of the challenge.
This code builds up and returns a list, but the instructions say that the function "should return the name of the teacher with the most courses." So the return should be a single string containing the name of just one teacher.
Also, for the challenge, your code should not "print" anything.