Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial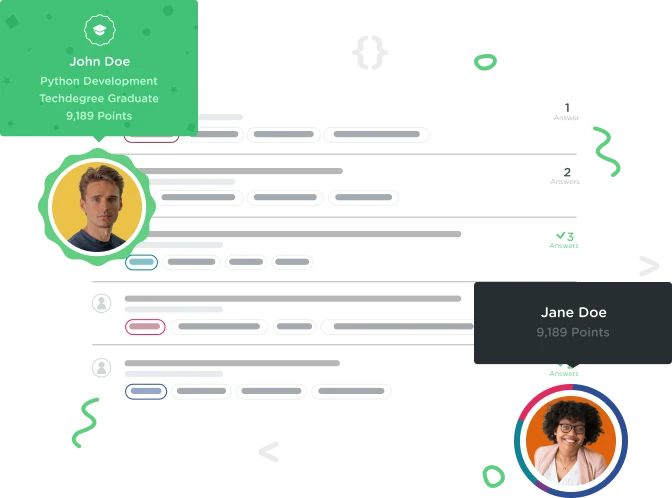

Dmitri Weissman
4,933 Pointsmost_courses is failing to pass regardless of the output
The code bellow is working perfectly fine if executed on my computer and in workspaces. Currently, when submitting to the system, all variants of the most_courses function get the "Bummer! Try again!" message. Functions return the full name of the teacher with the most courses. I tried to:
- return only the first name of the teacher
- return str(name)
another weird problem is that with the first commented version of the most_courses function, the system was complaining that I'm trying to use len() on int - line 27. So I had to assign the argument dic to local var doc - line 25. Again, the code worked in workspaces and on my computer without this reassignment.
Any help/explanation would be appreciated.
Thanks, Dmitri.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dic):
return len(dic)
def num_courses(dic):
l = 0
for t in dic:
l += len(dic[t])
return l
def courses(dic):
t = list()
for t in dic:
t.extend(dic[t])
return t
'''
def most_courses(dic):
doc = dic
for t in doc:
doc[t] = len(doc[t])
print(doc)
return (list(doc.keys())[list(doc.values()).index(sorted(list(doc.values()))[-1])])
def most_courses(dic):
doc=dic
for k in doc:
doc[k] = len(doc[k])
s = sorted(list(doc.values()))
print(s)
idx = s[-1]
for name, courses in doc.items():
if courses == idx:
return list(name.split())[0]
'''
def most_courses(dic):
m = 0
teacher = ''
for name, courses in dic.items():
if len(courses) > m:
m = len(courses)
teacher = name
return teacher
4 Answers

Manish Giri
16,266 PointsYou're right! There was a bug in the code, it worked because in the given data, the last key's value had the maximum length.
Here's the cleaned up code -
def num_courses(tdict):
return sum(len(v) for k,v in tdict.items())
def most_courses(tdict):
max_count = 0
name = ''
for k,v in tdict.items():
if(len(v) > max_count):
max_count = len(v)
name = k
return name
Also, I cleaned up the num_courses
method to use len(v)
instead of len(tdict[k])
.
In fact, I just realized there's a much better way to write the most_courses()
method using max()
-
def most_courses(tdict):
return max(tdict, key = lambda k: len(tdict[k]))
Isn't Python wonderful!

Dmitri Weissman
4,933 Pointsooh .... Manish Giri had a "bug" in the initial post. it worked because the test dictionary had the most courses in the last dictionary value.
your code seems to be completely fine.

Manish Giri
16,266 PointsThis is what worked for me -
def most_courses(tdict):
max_count = 0
name = ''
for k,v in tdict.items():
if(len(v) > max_count):
name = k
return name
Code till task 4/5 -
def num_teachers(tdict):
return len(tdict)
def num_courses(tdict):
return sum(len(tdict[k]) for k,v in tdict.items())
def courses(tdict):
clist = []
for i in tdict.values():
clist.extend(i)
return clist
def most_courses(tdict):
max_count = 0
name = ''
for k,v in tdict.items():
if(len(v) > max_count):
name = k
return name

Dmitri Weissman
4,933 PointsThat's interesting. I liked your version of the num_courses() very much :) Though I can't understand how your version of most_courses works since the max_count is always 0. So it supposed to return the last checked name from the dictionary. What do I miss ?
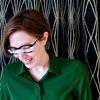
Vanessa Van Gilder
7,034 PointsI'm with Dmitri. Manish Giri--could you explain that? I ended up doing:
def most_courses(teach_dict):
most = 0
for teacher,courses in teach_dict.items():
if len(courses) > most:
most = len(courses)
name = teacher
return name

Dmitri Weissman
4,933 PointsHi Vanessa, we discussed few things here. Which part you want to be explained? If it's about some of the correct functions not working, there are a lot of bugs I've encountered. If your code works on your computer, simply restart the task and retype everything. The retyping should be done at the end of the file as sometimes the validation program fails to merge your code into test code. If it's about beautiful
def most_courses(tdict):
return max(tdict, key = lambda k: len(tdict[k]))
Just read about python max() and lambda. Later is just a way to do a nameless function in line. In short, max will return the largest value from an iterable (our list tdict). key = allows to use a function.
lambda k: len(tdict[k])
will do exactly the same as:
def whatever_function_name (k):
return len(tdict[k])
but the later can not be used withing max().
If it's anything else, just post it.
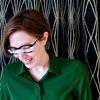
Vanessa Van Gilder
7,034 PointsSorry, I meant your question: "That's interesting. I liked your version of the num_courses() very much :) Though I can't understand how your version of most_courses works since the max_count is always 0. So it supposed to return the last checked name from the dictionary. What do I miss ?" I put
most = len(courses)
to update my maxnumber but Manish didn't...I was wondering how that could work without it.
Dmitri Weissman
4,933 PointsDmitri Weissman
4,933 PointsWow, that last version with max is beautiful and unfortunately beyond my skill for now :) There are more bugs from what I see. for example my version of courses()
while passing on it's own, will prevent any variant (including yours) of the most_courses() to pass. And yet surprising is that validation system still complains on len() with int in my version of the most_courses()
Thank you very much for your help :) now I can continue