Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial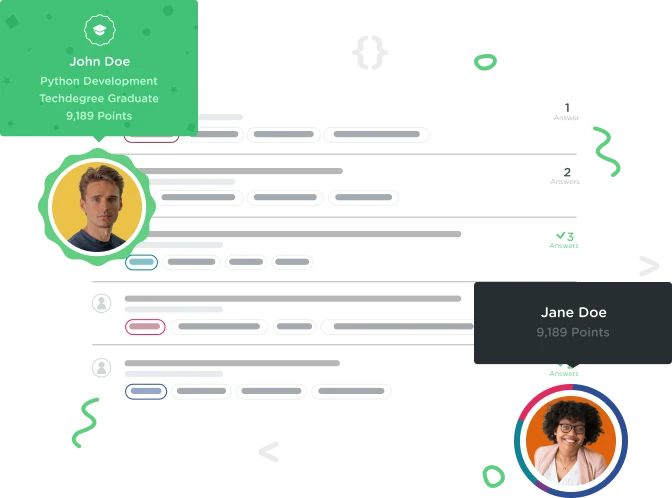
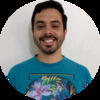
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsMove_player() Function
Before seeing how Kenneth coded the function, mine was like this:
def move_player(player, move):
if move == "LEFT":
for index, position in enumerate(CELLS):
if position == player:
player = CELLS[index - 1]
if move == "RIGHT":
for index, position in enumerate(CELLS):
if position == player:
player = CELLS[index + 1]
if move == "UP":
for index, position in enumerate(CELLS):
if position == player:
player = CELLS[index - 5]
if move == "DOWN":
for index, position in enumerate(CELLS):
if position == player:
player = CELLS[index + 5]
return player
When I ran the game, it works during the first or second loop, but I always keep getting this error:
Traceback (most recent call last): player = CELLS[index + 1] IndexError: list index out of range
I can't figure it out why it is out of the list range. For all the valid moves, the increment to CELLS[index] should not be out of range. If someone can explain me that I would really appreciate it.
2 Answers
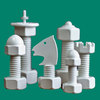
Steven Parker
241,811 PointsIt looks like the loop to find the player continues after the movement is applied. So if the player is moving right, the loop will keep updating the position until he is at the end of the cells list, and then the next iteration will cause the error.
You just need a "break" statement to be sure to end the loop when the player is found and the move has been made:
if move == "RIGHT":
for index, position in enumerate(CELLS):
if position == player:
player = CELLS[index + 1]
break # don't keep moving
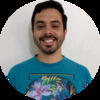
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsOh, man... Thank you so much! I spent a good chunk of my evening trying to figure out what I was missing. I get it now! Really appreciate it!
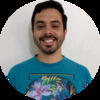
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsHey, Noob Developer!
Take a look at the list CELLS. Say you are in position (0, 2) and you want to move vertically up to position (0, 1). If you notice, when you go from the tuple (0, 2) to the tuple (0, 1), you skip 5 index positions, meaning you go from the index related to the (0, 2) tuple to a new index with is the previous index minus 5. The same goes if you want to move down, from (0, 1) to (0, 2) for example. The only diference is that you go from a certain index to another one wich is the the same as the previous plus 5. If you want to move right in the CELLS list, you only have to take your current index position and sum 1 to it.
To sum it up, you just have to look the CELLS list and see how many indexes you skip to go from a position to another for each move you make.
<noob />
17,063 Points<noob />
17,063 PointsHi i have a question about your code, what this line does? and the rest
player = CELLS[index + 5]