Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial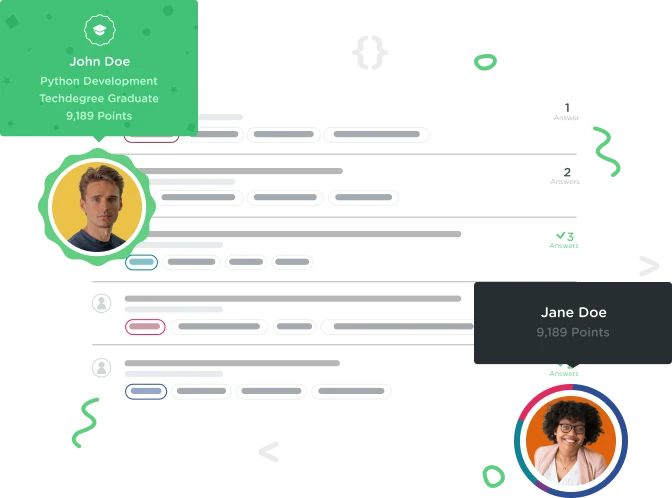

codymerrill
3,260 PointsMy answer (including extended questions at the end)
This way might be more convoluted than it needs to be, but this was how things made sense in my head.
// Lil Printer
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// Make an array to easily access student names w/ indices
// They are pushed as ".toUpperCase()" to make
// searches case insensitive
var studentNames = [];
for (var i = 0; i < students.length; i += 1) {
studentNames.push(students[i].name.toUpperCase());
}
// This makes our report and then prints it via Lil Printer
// It will loop through the every entry of "recordsMatching" and use
// each value as the index in "students"
function getReport() {
var report = '';
for (var i = 0; i < recordsMatching.length; i += 1) {
var recordIndex = recordsMatching[i];
var student = students[recordIndex];
report += '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
print(report);
}
}
// The Rest...
while(1) {
var search = prompt('Enter the name of a student');
// When and how to make the madness stop
if (search === null || search.toLocaleUpperCase() === 'QUIT') {
break;
}
// Make our searches case insensitive
search = search.toUpperCase()
// Look for a match to our search and store the index
var idx = studentNames.indexOf(search);
// If there is a match to our search
if (idx > -1) {
// Make an array to hold the indexes of our matches records
var recordsMatching = [];
// Push the index of each matching record to our new array
while (idx != -1) {
recordsMatching.push(idx);
idx = studentNames.indexOf(search, idx+1);
}
getReport();
}
// If there isn't a match to our search
else {
print('<h2>That person isn\'t real\&hellip\; Sorry.');
}
}