Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial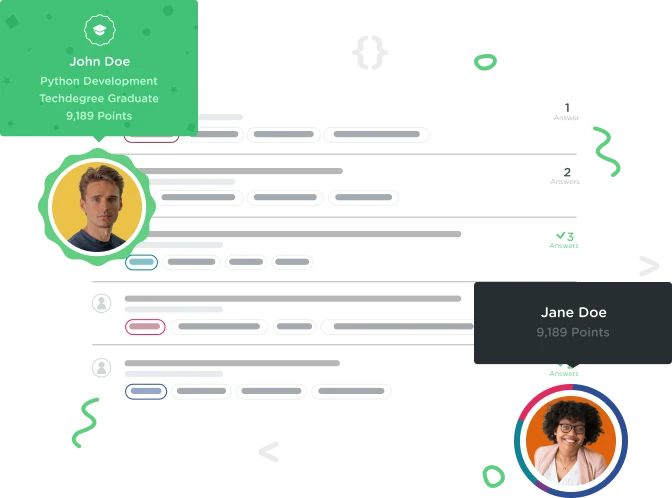

james oliver
Courses Plus Student 684 PointsMy answer with console.log without functions and pretty simple. maybe I should use a function but here's my solution.
// Loop prompt
while (true){
var answer = prompt('insert the student');
if (answer == 'quit'){
break;
}
//looping the array of object
for (var i=0; i < students.length; i++){
if(students[i].name == answer){
//console the students if match
for (prop in students[i]){
console.log(prop +": "+ students[i][prop]);
}
var checkNo = ''; //to check if doesn't exist and avoid repetition if one student is not in the array
}
}
//if is no blank there is not student. kind of return true when finish.
if (checkNo != ''){
console.log('No students with this name!');
}
}
1 Answer
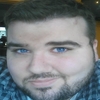
Marcus Parsons
15,719 PointsHey James,
When you're programming, try to think about what the user is going to see with your program. If someone didn't know JavaScript and ran your program as is, they would have no idea how to quit the program. I'm sure they would eventually type in quit to the box, but you need more information if you're just going to use "quit" as the breaking point of the student search.
Personally, I would use the natural cancel button that is on the prompt to exit out of the loop. All you have to do is check for any falsey value because when you click cancel on a prompt it will return a falsey value such as null or "":
//if answer is any falsey value
if (!answer) {
//break out of the loop
break;
}
I also noticed that checkNo
isn't actually used. Your if statement will never execute because checkNo
will always be an empty string with your current code. The easiest way to use checkNo
would be set to as a boolean instead of an empty string and set your variables at the beginning. Set it to true/false depending on how you wanted to do it. I would set checkNo
to be false at first and only true if a student isn't found.
james oliver
Courses Plus Student 684 Pointsjames oliver
Courses Plus Student 684 PointsHi Marcus,
Nice feedback, very appreciate it. I just tried to post the logic of the problem because I wanted to know the opinions. You're right I should do something for the user. But now that you make me think a bit further I would like to ask you why you think that checkNo is not used. I mean is there because without this variable I don't know how to know from outside the for loop that everything is finish and printed to the console. otherwise it will console.log() many times whether or not the student is found. You gave me a good hint about use Boolean in this case but I would like to know whats the difference in this case? I mean... I know that is a better practice use Boolean rather than empties arrays.. I'm sure that I'm missing something here!!! I look forward for you second feedback! thank you very much!