Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial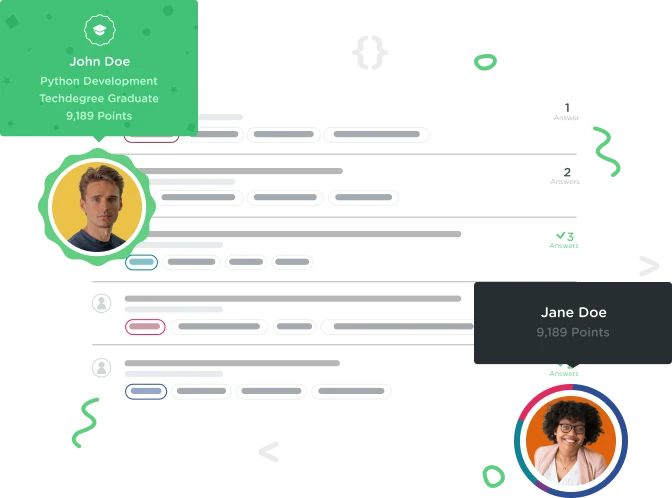

Hiren Mistry
1,138 PointsMy attempt before the video: the output is strange - need some help!
The problem is that when I type:
airplane, the output is : rpln (which is correct)
airplan: the output is: irpln
aeroplane: rpln (Which is correct)
air: ir
The try clause gets skipped if the vowel never appeared in the word in the first place.
I thought of trying to put except: (NameError, ValueError)
...but it did nothing
Here is the code:
print("Give me a word and I'll devowel it.")
user_input = input("> ")
new_list = list(user_input)
for vowel in new_list: try: new_list.remove('a') new_list.remove('e') new_list.remove('i') new_list.remove('o') new_list.remove('u') except ValueError: continue
devoweled = "".join(new_list) print("{} has been devoweled to {}.".format(user_input,devoweled))
2 Answers
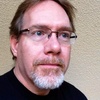
Chris Freeman
Treehouse Moderator 68,441 PointsYour code loops for each character in new_list. On each loop, the try
statement tries to remove the first instance of each vowel, but stops processing the first time a vowel is not longer present.
Given "airplane", the first pass removes one "a", one "e", one "i", then has an exception because there is no "o". The second pass removed next "a", then has an exception because there is no more "e". All subsequent passes hit the exception because there is not longer an "a'. Result: "rpln". OK.
Given "air", the first pass removes one "a", then has an exception because there is no "e". All subsequent passes hit the exception because there is not longer an "a'. Result: "ir".
The first correction: It is strongly recommended to not operate on the collection used in a for
statement. In this case, you are directlly changing the list of characters new_list
. While it might work in some situations, changing collection length or changing indexes has bad side effects. Better to copy list or collect the results in another list.
Ignoring the above correction, for the moment, you could fix your code by separating your try
statement into multiple statements:
for vowel in new_list:
try:
new_list.remove('a')
except ValueError:
pass
try:
new_list.remove('e')
except ValueError:
pass
try:
new_list.remove('i')
except ValueError:
pass
try:
new_list.remove('o')
except ValueError:
pass
try:
new_list.remove('u')
except ValueError:
pass
# continue # <-- continue not needed if last incode block
While this works, your code is now thrashing trying to remove vowels that are no longer there. You could check if the character under test is actually a vowel before removing:
for vowel in new_list:
if vowel in "aeiou":
new_list.remove(vowel)
However, this now fails, on "aire" because it still is operating on the for
statement collection new_list'. This can be corrected by taking advantage of the
for ... inautomatically treating a string as a collection. Replacing
new_listwith
user_input` gives you:
print("Give me a word and I'll devowel it.")
user_input = input("> ")
new_list = list(user_input)
for vowel in user_input: # <-- changed collection to input string
if vowel in "aeiou":
new_list.remove(vowel) # <-- operating on created list
devoweled = "".join(new_list)
print("{} has been devoweled to {}.".format(user_input,devoweled))
Finally, an alternative would be to simply collect the non-vowels:
print("Give me a word and I'll devowel it.")
user_input = input("> ")
new_list = [] # <-- intialized to empty list
for character in user_input: # <-- using input string
if not character in "aeiou":
new_list.append(character) # <-- operating on created list
devoweled = "".join(new_list)
print("{} has been devoweled to {}.".format(user_input,devoweled))

Hiren Mistry
1,138 PointsThank you! Your help is very clear!
Riley Gelwicks
6,214 PointsRiley Gelwicks
6,214 PointsVery clear thanks for the help!