Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial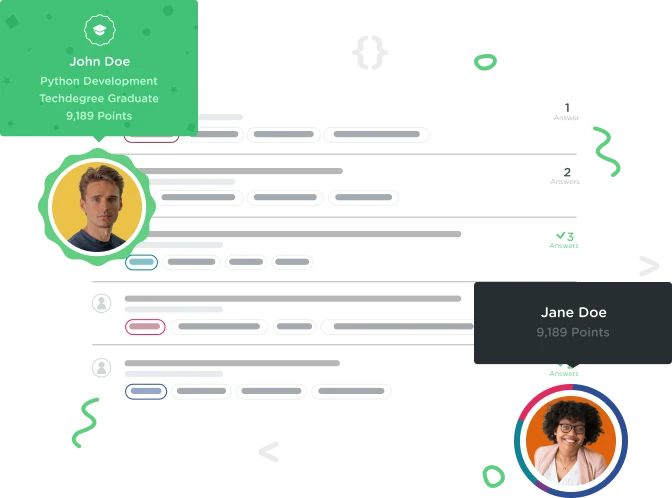

nicholassnider
2,568 PointsMy code is not compiling even though there is no compiler error and is working in Xcode. Any ideas?
There's no compiler error in treehouse and is doing what the challenge asks for in Xcode. Help!
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: String) {
self.title = title
self.author = author
self.tag = Tag(name: tag)
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "My First Post", author: "Nick", tag: "new")
let postDescription = firstPost.description()
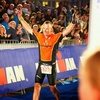
Steve Hunter
57,712 PointsThat's because it isn't passing the tests. It is syntactically correct but incomplete as far as the challenge is concerned.
1 Answer
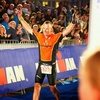
Steve Hunter
57,712 PointsHi Nicolas,
Which bit isn't working? At first glance, I can see one error; you've not passed a Tag
into the init
method when you've created firstPost
. That should look something like:
let firstPost = Post(title: "Title", author: "Me", tag: Tag(name: "aTag"))
Your init
method is expecting a tag: String
but the struct
has a tag: Tag
.
Try correcting that - I'll keep working through the challenge and comparing my code with yours too.
Steve.
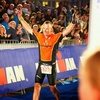
Steve Hunter
57,712 PointsRight ...
You can't use tag.name
in your returned string as you haven't created a Tag
instance; you're calling .name
on a String
. Two things to change; bin the init
method. Then, when you create firstPost
actually create a Tag
and pass that in, like I did above.
Then, your description method should be OK as it can call name
on a viable Tag
instance.
Make sense?
Steve.
My code:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "Title", author: "Me", tag: Tag(name: "Tag"))
let postDescription = firstPost.description()
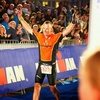
Steve Hunter
57,712 PointsThat's not quite correct; apologies.
The challenge tests are expecting to send a Tag
object into the firstPost
creation - I don't know why that doesn't get tested in the first part of the challenge, I'll be honest. There must be some semantic test that means your eloquent workaround isn't quite passing the tests as set. There's probably a good reason for that - I just can't see it right now!

nicholassnider
2,568 PointsThanks for your help Steve, I got it figured out. I got rid of the init method and called the Tag instance in my firstPost constant. I guess I over-thought it a little.
nicholassnider
2,568 Pointsnicholassnider
2,568 PointsIt simply says "Bummer! Your code could not be compiled. Please click on "Preview" to view the compiler errors." Yet there is no compiler error