Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial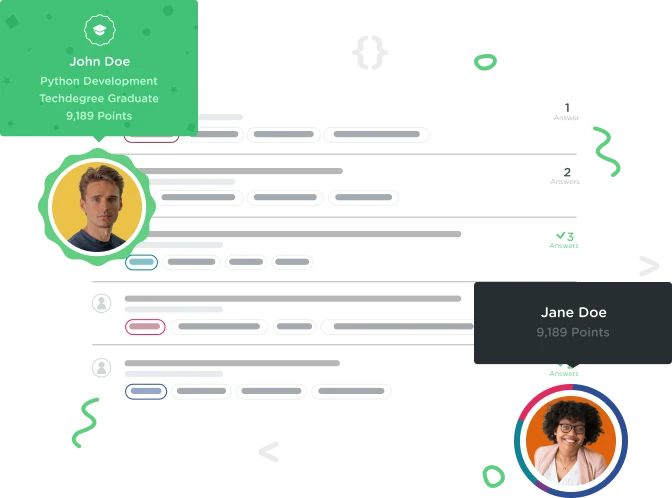
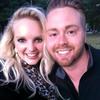
Jesse Fister
11,968 Pointsmy code is not correct?
I'm not sure what is wrong with my code. The challenge asks to create a while loop and have the user guess the password until they reach the answer of "sesame" Thanks.
var secret = prompt("What is the secret password?");
var guess;
while (guess !== "sesame") {
secret = "sesame";
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
2 Answers
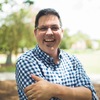
Dave McFarland
Treehouse TeacherHi Jesse Fister
Inside the while
loop you need to use the prompt()
method again, so that the user gets to try again...and again...and again, until the secret word is provdied. Your program just sets the variable to the secret word in the loop.

Jessica Barnett
8,028 PointsOkay, I see a few problems here. First your initial variables...
var secret = prompt("What is the secret password?");
// This will ask the user for their input only 1 time, which isn't what we want.
var guess;
// this variable is never getting set to anything, so it will always equal 'undefined'
Then the while loop. This is the part of the code that will repeat, so you definitely want to move your prompt to the user in here somewhere.
In your loop, you're comparing guess (which equals undefined) to "sesame". Neither of these values is ever getting changed, so your condition will always return true!
// This loop will never end because "undefined" and "sesame" don't change
while (guess !== "sesame") {
secret = "sesame";
}
I'd refactor the code to be more like this. The three main things I did were:
Switch the variables around. I would guess from the variable names that
secret
is meant to hold a secret password, like "sesame", and thatguess
is meant to hold the user's guess.I moved the prompt into the while loop, so the user will be asked for their guess multiple times.
I changed the conditions in the while loop so the loop will end when the user's guess from the prompt (stored in
guess
) matches the secret password "sesame" (stored insecret
)
var secret = "sesame"; // this sets your secret password.
var guess; // this will hold the user's guesses
//this loop will ask the user for input until their input matches secret
while (guess !== secret) {
guess = prompt("What is the secret password?"); // here you're just re-setting the guess variable with the user's input
}
//we've now exited the loop, which means the user gave us the right input (the secret password)
document.write("You know the secret password. Welcome.");
Hope that helps, and isn't too confusing...
Best of luck!