Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial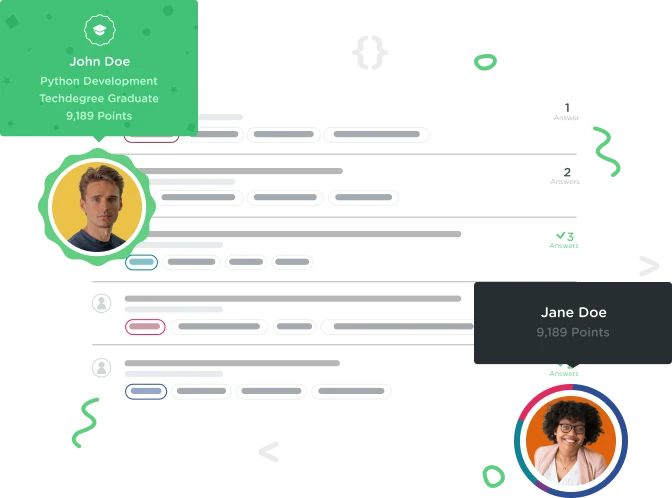

Matthew Kaiser
2,597 PointsMy code is working in an IDE... TT won't accept it... The "hint" is that my function isn't working... What do I do??
function max(randoNum1, randoNum2) { var randoNum1 = Math.floor( Math.random() * 0 ) + 1; console.log(randoNum1); var randoNum2 = Math.floor(Math.random() * 300 ); console.log(randoNum2); if (randoNum2 > randoNum1) { return randoNum2; } else { return randoNum1; } }
max();
Here you see my randoNum1 variable will always produce 1 and will always be less than randoNum2.
In my IDE the output shows as always being the larger number. Even if randoNum1 wasn't purposefully set to always be 1, the function would output the larger number.
So why can this code not be accepted by Team Treehouse? I'm frustrated / excited lol. Thanks for your time and help!!
function max(randoNum1, randoNum2) {
var randoNum1 = Math.floor( Math.random() * 0 ) + 1;
console.log(randoNum1);
var randoNum2 = Math.floor(Math.random() * 300 );
console.log(randoNum2);
if (randoNum2 > randoNum1) {
return randoNum2;
} else {
return randoNum1;
}
}
max();
1 Answer
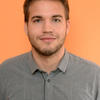
Adam Beer
11,314 PointsYou overcomplicated the solution. That's it. But this isn't problem. Just focus on the question. Happy coding!
function max(cucumber, apple){
if ( cucumber < apple ) {
return apple;
} else {
return cucumber;
}
}
alert(max(5, 9));
Antti Lylander
9,686 PointsAntti Lylander
9,686 Pointsyeah, NEVER do anything else that is instructed. :D BTW, you can do this without using
else
at all. Can you figure out how?Matthew Kaiser
2,597 PointsMatthew Kaiser
2,597 PointsThis code worked for me:
function max(x, y) { if (x > y) { return x; } else { return y; } }
max(12, 4);
I appreciate your help.
Antti, I tried to accept your challenge and do it without the 'else' but it gave me an error message saying 'max' wouldn't return a value. I think I have to write basically exactly what they want me to write, because it worked in my IDE without else.
Antti Lylander
9,686 PointsAntti Lylander
9,686 PointsThis is how it can be done without
else
. If the first condition is met, return will exit the function. If b is greater (or equal) than a, b will be returned. Also passes this challenge.