Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial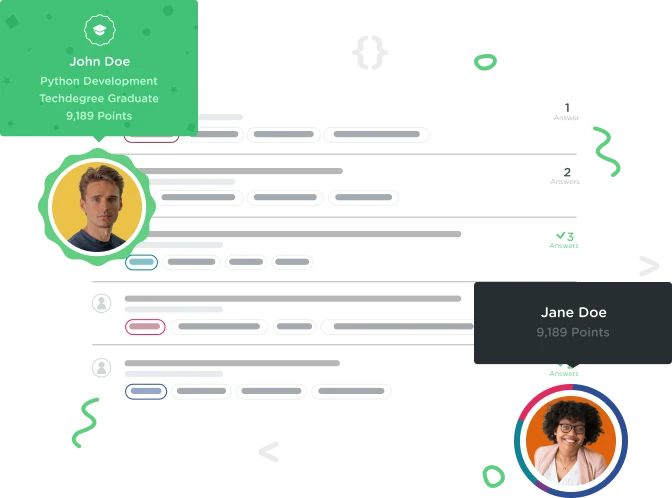

Colin Sygiel
5,249 PointsMy code isn't running the prompts. What gives?
/* Quiz: Ask 5 questions, keep track of the number of questions answered correctly, provide results with how many questions they got right and give player a ranking */
/*5 Star Wars questions, stored in separate variables */ var questionOne = prompt ("What is the name of Han Solo\'s furry friend?"); var questionTwo = prompt ("What is the last name of Luke?"); var questionThree = prompt ("What is the name of the dark side\'s secret weapon?"); var questionFour = prompt ("What is the name of Luke\'s father?"); var questionFive = prompt ("What is the first name of the mysterious man Han solo and everyone else visits?");
/Contain correct answers and count each correct answer as a point/
If (questionOne === 'Chewbacca') { var answerOne = 1 }
If (questionTwo === 'Skywalker') { var answerTwo = 1 }
If (questionThree === 'Death Star') { var answerThree = 1 }
If (questionFour === 'Darth Vader') { var answerFour = 1 }
If (questionFive === 'Lando') { var answerFive = 1 }
/Compare points earned and give a score/
If (answerOne + answerTwo + answerThree + answerFour + answerFive = 5) { document.write ('Congrats, you have earned the Gold Star!') }
If (answerOne + answerTwo + answerThree + answerFour + answerFive = 2 || answerOne + answerTwo + answerThree + answerFour + answerFive = 3 || answerOne + answerTwo + answerThree + answerFour + answerFive = 4) { document.write ('Congrats, you have earned the Silver Star!') }
If (answerOne + answerTwo + answerThree + answerFour + answerFive = 1) { document.write ('You have earned the Bronze Star!') }
2 Answers
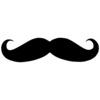
hum4n01d
25,493 PointsThere are a few issues with your code.
- You need to remove the spaces before calling prompt
- You should have a space between your if statement and the condition
- You are using single equal signs when you should be using double or triple equal signs to check for equality
Your code should look like this:
/* Quiz: Ask 5 questions, keep track of the number of questions answered correctly, provide results with how many questions they got right and give player a ranking */
/*5 Star Wars questions, stored in separate variables */
var questionOne = prompt("What is the name of Han Solo\'s furry friend?");
var questionTwo = prompt("What is the last name of Luke?");
var questionThree = prompt("What is the name of the dark side\'s secret weapon?");
var questionFour = prompt("What is the name of Luke\'s father?");
var questionFive = prompt("What is the first name of the mysterious man Han solo and everyone else visits?");
//Contain correct answers and count each correct answer as a point/
if (questionOne === 'Chewbacca') {
var answerOne = 1;
}
if (questionTwo === 'Skywalker') {
var answerTwo = 1;
}
if (questionThree === 'Death Star') {
var answerThree = 1;
}
if (questionFour === 'Darth Vader') {
var answerFour = 1;
}
if (questionFive === 'Lando') {
var answerFive = 1;
}
//Compare points earned and give a score/
if (answerOne + answerTwo + answerThree + answerFour + answerFive == 5) {
document.write('Congrats, you have earned the Gold Star!')
}
if (answerOne + answerTwo + answerThree + answerFour + answerFive == 2 || answerOne + answerTwo + answerThree + answerFour + answerFive == 3 || answerOne + answerTwo + answerThree + answerFour + answerFive == 4) {
document.write('Congrats, you have earned the Silver Star!')
}
if (answerOne + answerTwo + answerThree + answerFour + answerFive == 1) {
document.write('You have earned the Bronze Star!')
}

Colin Sygiel
5,249 PointsThanks for the link, that is very helpful. The prompts work now, but the console.log display of score is not showing for me. Is it for you?
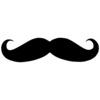
hum4n01d
25,493 PointsI think your conditions arenβt allowing the message to be printed :)
Could you mark my answer as correct please?
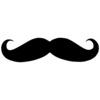
hum4n01d
25,493 PointsThanks ;)
hum4n01d
25,493 Pointshum4n01d
25,493 PointsIβm working on it, there are a few more problems than I realized
Colin Sygiel
5,249 PointsColin Sygiel
5,249 PointsI see that now - thanks so much :)
hum4n01d
25,493 Pointshum4n01d
25,493 PointsDoes that work for you? I edited the post
hum4n01d
25,493 Pointshum4n01d
25,493 PointsCould you mark my answer as correct please?
hum4n01d
25,493 Pointshum4n01d
25,493 PointsThis may be useful to you: https://jsfiddle.net/f035ytz4/4/