Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial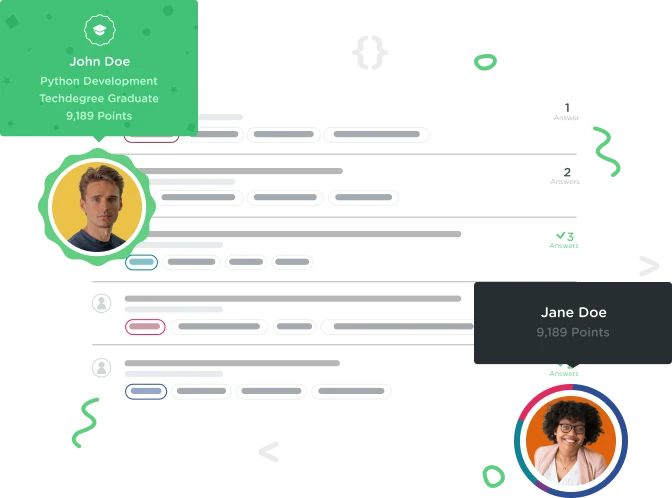
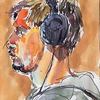
Sean Maden
3,504 PointsMy code returns {'am' : 2, 'i' : 2, 'that' : 1} but it throws the error: "Some of the words seem to be missing."
I am starting with the string "I am that I am," but my code doesn't use all of the recommended commands. However, my output seems to be correct nonetheless.
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
my_str = "I am that I am"
my_str = my_str.lower()
x = my_str.split()
def word_count(my_str):
x = list(my_str)
position = 0
d={}
d[x[position]]=0
while position < len(x):
if x[position] not in d:
d[x[position]]=1
position+=1
continue
else:
d[x[position]]+=1
position+=1
continue
print(d)
return d
word_count(x)
2 Answers
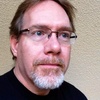
Chris Freeman
Treehouse Moderator 68,426 PointsHi Sean, It seems you have implemented a character counter instead of a word counter. When I run your code I get:
word_count(my_str)
{'a': 3, ' ': 4, 'i': 2, 'h': 1, 'm': 2, 't': 2} # <-- from print(d)
Out[104]: {' ': 4, 'a': 3, 'h': 1, 'i': 2, 'm': 2, 't': 2}
It looks like you need to first split it incoming string into words first using:
word_list = my_str.split()
Then loop over the items in word_list
and count each word.
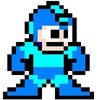
Robert Richey
Courses Plus Student 16,352 PointsHi Sean Maden,
That's a great attempt! This is a really tricky challenge, but we can get through it. Let's try and walk through a pseudo-code solution. When you find yourself stuck like this, try writing pseudo code that describes what you'd like to do, and then figure out what code you need to do it.
Also, we only need to write the function. The challenge will pass in it's own string.
# 1. Create a function named word_count() that takes a string.
# 2. Return a dictionary [...]
def word_count(my_str):
d = {}
# so far so good. at this point, we'll need a list holding the words from my_str - lowercased
# for each word in the list:
# if this word is already in d:
# d[word] += 1
# else:
# d[word] = 1
return d;
Try this out and please let me know if this helps or not.
Cheers!
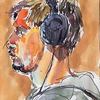
Sean Maden
3,504 PointsI think this approach is helpful in general, and I definitely started the challenge on this line of thinking. I got hung up for awhile trying to force and answer using something like "for word in dict:" as a loop, so ultimately I settled on taking advantage of ordering and position in the word list.
Sean Maden
3,504 PointsSean Maden
3,504 PointsGood call, I may have accidentally pasted an old version of my code.
Out of curiosity, what was the string you used when you ran it at first?
Chris Freeman
Treehouse Moderator 68,426 PointsChris Freeman
Treehouse Moderator 68,426 PointsI ran using your
my_str
. Now that you asked, I looked back and see you setx = my_str.split()
. When I runword_list(x)
I get:Same as you.
The challenge likely didn't pass because it was expecting the split inside the function.
Sean Maden
3,504 PointsSean Maden
3,504 PointsEDIT: just saw your comment above, and I concur - I think the challenge was looking for code that takes commands in a certain order or uses them within the definition (even though I come up with a satisfactory answer here)
I realized something that probably is throwing you off (it took me a while to catch it!). Basically, it is not a character counter in this instance because I predefine x as a word list and then pass x to the function. What is confusing is I also used a new variable "x" that makes a list out of whatever argument is passed to the function (in this case the argument happens to be "x"). So it actually works out as a word counter with the variables I have defined, even though the function itself doesn't use a split command in its definition. Hopefully that clears this up a bit :P