Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial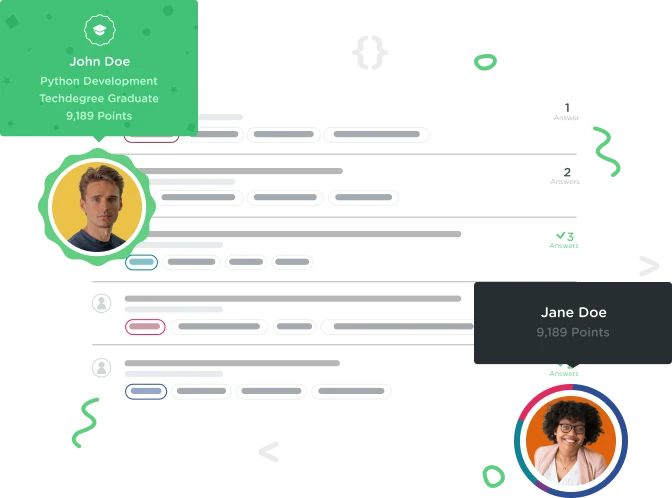

Crystal Shelton
Courses Plus Student 1,520 PointsMy code works in the workspace but not in the challenge area?
My dictionary defined only has 5 courses in it so why is the challenge telling me that I should have 18?
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
dictionary = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(dictionary):
max_count = 0
teacher_name = ""
for key in dictionary:
classes = 0
for value in dictionary[key]:
classes += 1
if classes > max_count:
max_count = classes
teacher_name = key
else:
continue
continue
return teacher_name
most_classes(dictionary)
def num_teachers(dictionary):
count = 0
for key in dictionary:
count += 1
return count
num_teachers(dictionary)
def stats(dictionary):
my_list = []
for key in dictionary:
count = 0
for value in dictionary[key]:
count += 1
my_list.append([key, count])
return my_list
stats(dictionary)
def courses(dictionary):
my_list = []
for key,value in dictionary.items():
my_list.append([value])
return my_list
courses(dictionary)
4 Answers
Graham Mackenzie
2,747 PointsGlad my first response helped!
I think you're reading the challenge correctly. What's happening is that your courses()
function is currently only returning the first course each teacher is offering. In order for it to add every course, you'd need to use nested for
loops like you did for stats()
, except instead of increasing the count, append every value
to the list that gets returned. Let me know if that does the trick! And, again, please designate my answer as the best one, if so.
Thanks! and Be Well, Graham
Graham Mackenzie
2,747 PointsHey Crystal!
So, this is something that I've seen trip up a lot of people on here, but one of the things about these Treehouse Challenges is that - often without saying so explicitly - what they are doing as part of the challenge is running your code and sending it their own values.
In other words, they are automatically sending your code a pre-made dictionary with 18 classes in it, which is why the challenge is throwing an error when they only get back the 5 that your coding has overridden it with. You can tell that this is what they are doing by their inclusion of the phrase "The dictionary will be something like:" (italics mine) in the comments above the code you write. They didn't actually want you to copy and paste the code in that comment, they were just letting you know what the dictionary they were sending looked like so you'd know how to handle it.
The reason it worked in Workspace, by comparison, is because there is no pre-made dictionary being sent to your function there, in which case the one you coded sufficed.
The key is to remove dictionary = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
from your code. Additionally, you can remove the following function calls as well: most_classes(dictionary)
, most_classes(dictionary)
, stats(dictionary)
, and courses(dictionary)
; the challenge environment already runs those functions as a means of testing your code, so all you have to do is define them and click the pink "Check Work" button.
I hope this helps! Please select this as Best Answer if you found it most helpful.
Be Well, Graham

Crystal Shelton
Courses Plus Student 1,520 PointsThanks Graham for your response. I tried removing the dictionary definition and removing the function calls and it stills says "Bummer! You returned 5 courses and you should have returned 18." Here is my code: def courses(dictionary): my_list = []
for key,value in dictionary.items(): my_list.append([value]) continue
return my_list
I am wondering if the challenge is meaning something different than the way I am reading it. The challenge says to "Write a function called courses() that takes the dictionary of teachers. It should return a list of all the courses offered by the teachers." I read this to say that I should return just a list of all of the classes available. Am I reading the challenge wrong?

Crystal Shelton
Courses Plus Student 1,520 PointsThanks again for your help Graham. It still did not work when I made the change that you suggested. It had trouble with the brackets around the value variable in the append statement. Once I removed the brackets it passed!