Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial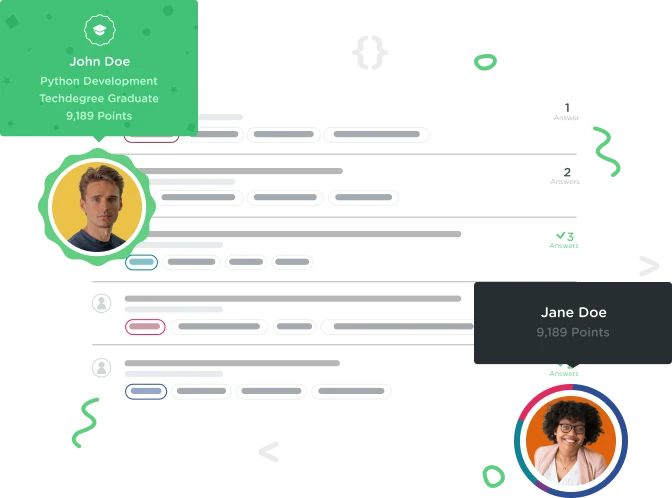

Hanley Chan
27,771 PointsMy dungeon game solution
Hi, I just finished my dungeon game and would like some feedback.
import random
def print_map():
print("MAP (P = player, ? = unvisited room, x = visited room):")
for y in range(0,rows):
for x in range(0,cols):
if (x,y) == player_loc:
print("P",end= " ")
#elif (x,y) == monster_loc: # uncomment to show monster location on map
# print("M",end = " ")
#elif (x,y) == door_loc: # uncomment to show door location on map
# print("D",end = " ")
else:
if (x,y) in movement_history:
print("x",end = " ")
else:
print("?",end = " ")
print("\n")
def move_player(move):
if move in getValidMoves():
x,y = player_loc
if move == "UP":
return (x,y-1)
elif move == "DOWN":
return (x,y+1)
elif move == "LEFT":
return (x-1,y)
else:
return (x+1,y)
else:
print("\nThat is not a legal move")
return player_loc
def getValidMoves():
x,y = player_loc
if x == 0 and y == 0:
return (tuple(("DOWN","RIGHT")))
elif x == cols-1 and y == rows-1:
return (tuple(("UP","LEFT")))
elif x == 0 and y == rows-1:
return (tuple(("UP","RIGHT")))
elif x == cols-1 and y == 0:
return (tuple(("DOWN","LEFT")))
elif x == 0 and y < rows-1:
return (tuple(("UP","DOWN","RIGHT")))
elif x < cols-1 and y == 0:
return (tuple(("DOWN", "LEFT","RIGHT")))
elif x < cols-1 and y == rows-1:
return (tuple(("UP", "LEFT", "RIGHT")))
elif x == cols-1 and y < rows-1:
return (tuple(("UP", "DOWN", "LEFT")))
else:
return (tuple(("UP","DOWN","LEFT","RIGHT")))
# generate game map
rows = 4
cols = 5
GAME_GRID = list()
movement_history = list() # contains all moves that the player has made
for x in range(0,cols):
for y in range(0,rows):
GAME_GRID.append(tuple((x,y)))
# generate player location
player_loc = random.choice(GAME_GRID)
movement_history.append(player_loc)
# generate monster location. Make sure it is not the same as player location
while True:
monster_loc = random.choice(GAME_GRID)
if monster_loc == player_loc:
continue
else:
break
# generate door location. Make sure it is not the same as player or monster location
while True:
door_loc = random.choice(GAME_GRID)
if door_loc == player_loc or door_loc == monster_loc:
continue
else:
break
while True:
print_map()
print("\nYou're currently in room {}".format(player_loc))
print("Enter a valid move:")
validMoves = getValidMoves()
for _, validMove in enumerate(validMoves):
print(validMove)
print("\nEnter QUIT to quit\n")
move = input ("> ")
move = move.upper()
old_player_loc = player_loc
if move == "QUIT":
break
elif move == "UP":
player_loc = move_player("UP")
elif move == "DOWN":
player_loc = move_player("DOWN")
elif move == "LEFT":
player_loc = move_player("LEFT")
elif move == "RIGHT":
player_loc = move_player("RIGHT")
else:
print("That is not a valid command.\n")
continue
if old_player_loc != player_loc: # add to move history if player_loc changed
movement_history.append(player_loc)
if player_loc == monster_loc:
print("GAME OVER! You have been eaten by the monster")
print("You made {} moves\n".format(len(movement_history)-1))
break
if player_loc == door_loc:
print("YOU WIN! You have found the exit to the dungeon")
print("You made {} moves\n".format(len(movement_history)-1))
break
1 Answer

shezazr
8,275 Pointsthe only problem I found is that the room location is not consistent.. i.e. it says you are in room 4,2 but the player marker is actually in room 5,3..
Also having to type in Right/Left etc is annoying.. shortcuts like D, L, etc would be better
Hanley Chan
27,771 PointsHanley Chan
27,771 PointsHi shez azr, the reason the room numbers aren't correct is because my room numbers starts at (0,0). So should be adding 1 to all of the room the numbers. I probably should have started counting at room (1,1) so it's less confusing.
I agree about having shortcuts for input so I don't have to type as much input everytime. I will keep in mind for future assignments