Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial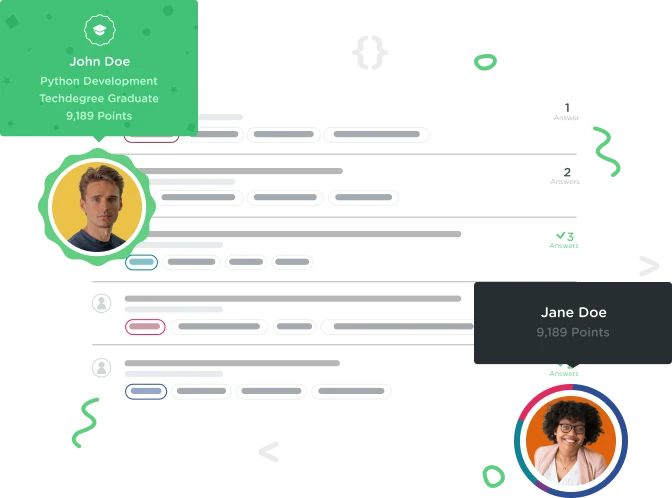
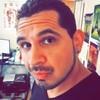
Daniel LittleThunder
6,975 PointsMy Example List: What I've Learned So Far
I just wanted to share my code for what I've learned in JS thus far. It takes the concepts in this course (a lot of code copying) and combines it with other techniques from this track. This code accepts user input and uses that to build an array, then prints the contents of that array to the screen. Below is the code. I hope this helps other users out there in some way. I'm going to keep working on this code. I think that I want to collect quantities and output the quantity of each item along with this code. Eventually, I would like to collect this information using an HTML structured and CSS styled form. I just have to figure out how to do it.
JavaScript :
//creates empty array named shoppingList
let shoppingList = [];
//creates a function to print the contents of an ordered list to the screen
function printList(orderedList) {
document.write(orderedList);
}
//builds array to use for list through user input
function buildList() {
//set varialbes and array
let isDone = false;
let addItem;
//construct loop break on boolean condition of 'if is false' or 'while var isDone is not true'
while (!isDone) {
//checks initial length of array and prompts user for input if array is empty
//if not empty, prompts user to add more items
if(shoppingList.length < 1) {
addItem = prompt("Welcome to your shopping list. Add an item to get things started. When you're finished, simply type 'done'.");
} else {
addItem = prompt("Great! Keep adding items to your list. Type 'done' when you are finished.");
}
//if input is numeric or null prompts user for non-numeric or non-null input
while (!isNaN(addItem) && addItem != null) {
addItem = prompt("Sorry. List items can contain numbers, but cannot contain only numbers.");
}
//adds input to array as long as input isn't 'done'
if (addItem.toUpperCase() != 'DONE') {
shoppingList.push(addItem);
}
//terminates program if input is 'done' or null
if (addItem.toUpperCase() === 'DONE' || addItem === null) {
isDone = true;
return shoppingList;
break
}
}
}
//builds ordered list using a for loop to iterate through the contents of the array then calls the print function
function createList(array) {
let list = '<h1>My Shopping List</h1>'
list += '<ol>';
for (i = 0; i < array.length; i++) {
list += '<li>' + array[i] + '</li>';
}
list += '</ol>';
printList(list);
}
//calls the build list function so user can create array through input
buildList();
//calls the create list function and prints results to the screen
createList(shoppingList);