Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial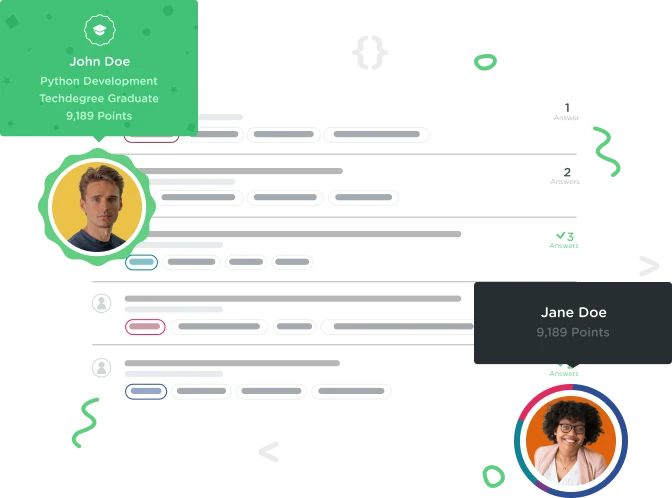

Michael Moore
7,121 PointsMy function works but is not accepted. Probably better ways to go about it, but it works. Please tell me why,
def word_count(string):
dictionary = {}
splits = string.split(" ")
for word in splits:
word = word.lower()
dictionary[word] = 0
for word in splits:
word = word.lower()
for key in dictionary.keys():
if word == key:
dictionary[word] = dictionary[word] + 1
print(dictionary)
return dictionary
word_count("I i I HaVE HAVE have A a A A a A Rea REA rea real b b B BAD stu STu STUT STUTtering stuttering Pro PRO pRo Problem")
word_count("I do not like it Sam I Am")
results in:
{'real': 1, 'b': 3, 'stuttering': 2, 'bad': 1, 'have': 3, 'stut': 1, 'a': 6, 'i': 3, 'rea': 3, 'st
u': 2, 'problem': 1, 'pro': 3}
{'not': 1, 'do': 1, 'i': 2, 'it': 1, 'sam': 1, 'am': 1, 'like': 1}
Please tell me why it is not being accepted. Did I misunderstand what it wanted? Seems pretty clear in the instructions it just wanted a word count by key: value.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
dictionary = {}
splits = string.split(" ")
for word in splits:
word = word.lower()
dictionary[word] = 0
for word in splits:
word = word.lower()
for key in dictionary.keys():
if word == key:
dictionary[word] = dictionary[word] + 1
print(dictionary)
return dictionary
word_count("I do not like it Sam I Am")
2 Answers

Michael Moore
7,121 PointsJust to let you know, it just didn't like the way my code was phrased, but it had the exact same effect.
you can look at my code above, versus the code below. I think the main thing it didn't like was me naming a dictionary
dictionary = {}
versus
dictionary = dict()
here was the code that works:
def word_count(string):
dictionary = dict()
splits = string.lower().split()
print(splits)
for word in splits:
dictionary[word] = 0
for word in splits:
for key in dictionary.keys():
if word == key:
dictionary[word] +=1
print(dictionary)
return dictionary
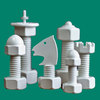
Steven Parker
231,236 PointsThe error message contains a hint: "Bummer! Hmm, didn't get the expected output. Be sure you're lowercasing the string and splitting on all whitespace!"
To make it split on all whitespace, the argument to "split" should be empty. Providing a space makes it split only on a space.
And you only need to define the function, you shouldn't call it yourself.

Michael Moore
7,121 PointsI actually did remove the (" ") and changed it to () in the split, and it gave the same result. I am lowercasing it, as shown above with the stuttering string. I saw the split(" ") vs split() and tried them both with the same outcome both times. Using their own sentence I am getting the exact same outcome they seem to expect.
And its not telling me I didn't get the expected outcome. Just saying, bummer, try again! Which I assume means the same thing, but according to their own sentence, it seems to be exactly what they wanted.

Michael Moore
7,121 PointsI wasn't calling it i my submission treehouse. That was taken from my workshop. I was just showing what I was passing in and what I was receiving back so those who viewed this could see what I was fully doing and the results it was receiving. The problem literally was either the way I had the .lower(), or the dict(). Those are the only 2 things that changed in my submission. It may well have not liked variable = variable.lower(), or it may have been the dict(), because those are the only 2 things that changed.
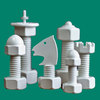
Steven Parker
231,236 PointsI did test my answer. After pasting in the code, removing the invocation and the argument to "split", it passed.
Steven Parker
231,236 PointsSteven Parker
231,236 PointsIn most of these challenges, they want you to just define a function. Calling it yourself will at best be ignored, but sometimes it will confuse the validator.
Also, in most cases, they only want it to return a value. Printing things in the function can also confuse the validator.