Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial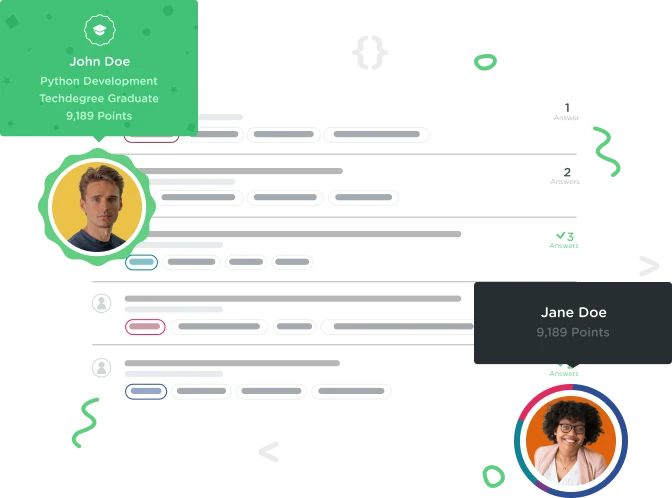

nagamani palamuthi
6,160 PointsMy photos are not displaying... pls help
SyntaxError: Unexpected token <
it shows the above error in the console. my code below:

nagamani palamuthi
6,160 Pointsthanks for your time... will fix and try again!!
2 Answers

Adam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsYou really should indent your code as it makes it easier to read and thus easier to debug. While JavaScript doesn't really care about spaces (or semicolons for that matter), you want to get in the habit of writing clean code. Writing code should be like writing an essay; not like sending a text message.
Also, be consistent with your quotation mark usage (except when avoiding using escapes in strings).
Here is what your code should look like:
$(document).ready(function() {
$("button").click(function() {
$("button").removeClass("selected");
$(this).addClass("selected");
//the ajax part
var flickerAPI = "http://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?" ;
var animal = $(this).text();
var flickrOptions = {
tags: animal,
format: "json"
};
var displayPhotos = function(data) {
var photoHtml = "<ul>";
$.each(data.items,function(i, photo) {
photoHTML += '<li class="grid-25 tablet-grid-50">';
photoHTML += '<a href="' + photo.link + '" class="image">';
photoHTML += '<img src="' + photo.media.m + '"></a></li>';
}); //end each
photoHTML += '</ul>';
$("#photos").html(photoHTML);
}; //end function
$.getJSON(flickerAPI, flickrOptions, displayPhotos);
});
});
Here is mine using ES6 syntax:
$(document).ready( () => {
// Button click handler
$('button').click( (event) => {
// Change appearance of buttons on click
$('button').removeClass('selected');
$(event.currentTarget).addClass('selected');
// AJAX call to Flickr API on click
const flickrAPI = `http://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?`;
const animal = $(event.currentTarget).text();
const flickrOptions = {
tags: animal,
format: 'json'
};
// Loop through Flickr response and append response to DOM
const displayPhotos = (data) => {
let photoHTML = `<ul>`;
$.each(data.items, (i, photo) => {
photoHTML += `<li class="grid-25 tablet-grid-50">`;
photoHTML += `<a href="${photo.link}" class="image">`;
photoHTML += `<img src="${photo.media.m}"></a></li>`;
});
photoHTML += `</ul>`;
$('#photos').html(photoHTML);
};
// GET JSON data from Flickr
$.getJSON(flickrAPI, flickrOptions, displayPhotos);
});
});

Derrick Johnson
4,347 PointsWouldn't it be easier to do this instead of doing a +=
3 separate times?
(images) => {
let html = `<ul>`;
$.each(images.items,(i,image) => {
html += `
<li class="grid-25 tablet-grid-50">
<a href="${image.link}" class="image">
<img src="${image.media.m}" />
</a>
</li>
`;
});
html += `</ul>`;
}

Adam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsDerrick Johnson you are correct; not sure why I didn't do a multi-line template literal, but that's how I've been doing them.
Jonathon Waunch
9,368 PointsJonathon Waunch
9,368 PointsCouple things:
If you fix those items, the photos should load.