Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial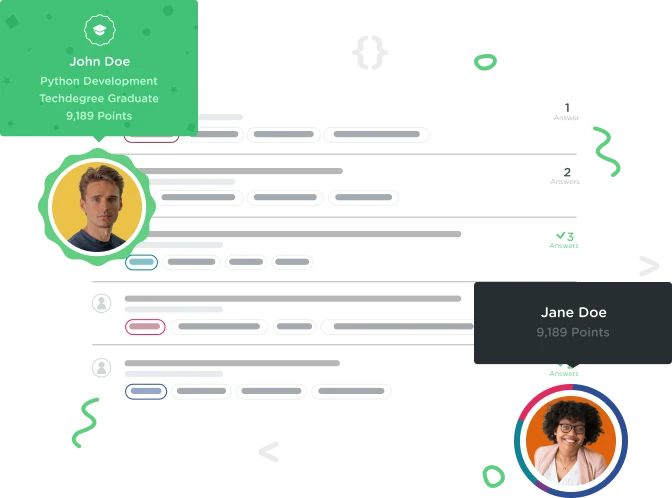

Mikey Neilson
Front End Web Development Techdegree Student 12,642 PointsMy score variables do not increment when I run my code??
Hi Guys could someone please tell me were I'm going wrong with this code. I want either the players_score or the cpu_score to increment by one whenever they win a round.. Any help would be appreciated Many Thanks :)
choice = ["rock", "paper", "Scissors"]
players_score = 0
cpu_score = 0
def win_lose(a,b,c,d)
if a == "rock" && b == "scissors"
c+=1
puts "YOU WIN!!"
elsif a == "scissors" && b == "rock"
d+=1
puts "YOU LOSE!!"
elsif a =="paper" && b == "rock"
c+=1
puts "YOU WIN!!"
elsif a =="rock" && b == "paper"
d+=1
puts "YOU LOSE!!"
elsif a == "scissors" && b == "paper"
c+=1
puts "YOU WIN!!"
elsif a == "paper" && b == "scissors"
d+=1
puts "YOU LOSE!!"
else a == b
puts "Its a Draw this time!!"
end
end
while players_score < 2 && cpu_score < 2
print "Lets play. Plese choose rock, paper or scissors: "
players = gets.chomp.downcase
puts "You have #{players}"
cpu = choice.sample.downcase
puts "Computer has #{cpu}"
win_lose(players, cpu, players_score, cpu_score)
puts "scores are player #{players_score} , cpu #{cpu_score}"
end
2 Answers

Greg Kaleka
39,021 PointsHi Mikey,
What you've run into is the fact that in Ruby, assignment operators are non-mutating. Essentially, what's happening is that you're passing in the values of players_score
and cpu_score
, changing those values locally within the scope of your function, and exiting the function. Meanwhile the original variables players_score
and cpu_score
are unchanged. Here's how I would change your code:
choice = ["rock", "paper", "Scissors"]
players_score = 0
cpu_score = 0
def win_lose(a,b,c,d)
if a == "rock" && b == "scissors"
c+=1
puts "YOU WIN!!"
elsif a == "scissors" && b == "rock"
d+=1
puts "YOU LOSE!!"
elsif a =="paper" && b == "rock"
c+=1
puts "YOU WIN!!"
elsif a =="rock" && b == "paper"
d+=1
puts "YOU LOSE!!"
elsif a == "scissors" && b == "paper"
c+=1
puts "YOU WIN!!"
elsif a == "paper" && b == "scissors"
d+=1
puts "YOU LOSE!!"
else a == b
puts "Its a Draw this time!!"
end
return [c,d] # new line
end
while players_score < 2 && cpu_score < 2
print "Lets play. Plese choose rock, paper or scissors: "
players = gets.chomp.downcase
puts "You have #{players}"
cpu = choice.sample.downcase
puts "Computer has #{cpu}"
result = win_lose(players, cpu, players_score, cpu_score) # storing the return value
players_score = result[0] # new line
cpu_score = result[1] # new line
puts "scores are player #{players_score} , cpu #{cpu_score}"
end
You can see I've only added three lines of code and one tweaked one.The function now returns the new player and cpu scores. We store the return value and then assign the new score values to players_score
and cpu_score
.
Hopefully this makes sense!
Cheers
-Greg

Mikey Neilson
Front End Web Development Techdegree Student 12,642 PointsHi Greg many thanks for help! I would just like to make sure I have a grasp on what you have done here first of all you have returned the value of c and d and stored it in a array called results,and then passing index 0 of the array to players_score var and then passing index 1 of the array to cpu_score.. Is that correct ??

Greg Kaleka
39,021 PointsYep - you got it! Note we're returning an array, and storing it in the variable result
. That's pretty much what you said, but just clarifying slightly

Mikey Neilson
Front End Web Development Techdegree Student 12,642 PointsOk Big thanks for your help Greg :)