Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial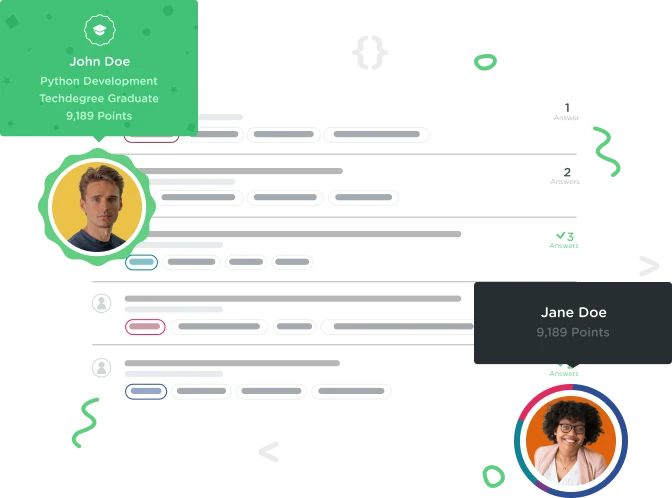
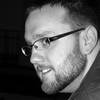
Norbert Stadler
4,015 PointsMy solution
So I faced some problems when I was writing the code:
1) What if the first given number is greater than the second? For this, I wrote an "if" statement (see below). 2) When I tried with two adjacent integers, I always got the greater number. I tried with 54 and 55 and always got 55 because of the floor and +1 method. I came up with this:
var numberX = prompt('Random number between x and y. Give the x number:'); numberX = parseInt(numberX); var numberY = prompt('Give the y number:'); numberY = parseInt(numberY);
if (numberX > numberY) { numberZ = numberX numberX = numberY numberY = numberZ }
alert(Math.round(Math.random() * (numberY - numberX) + numberX));
What do you think?
1 Answer

Andrei Fecioru
15,059 PointsHi,
You can also do something like this:
var a = Math.min(numberX, numberY);
var b = Math.max(numberX, numberY);
alert(Math.round(Math.random() * (b - a) + a));
But then again I would view this as a scenario where the input data is invalid. If I were to write a function which generates a random integer between two input integers I would do something like:
function generateRandInt(start, end) {
start = parseInt(start);
end = parseInt(end);
// check the input values.
if (isNaN(start) || // the start value is invalid
isNaN(end) || // the end value is invalid
(start >= end)) // the start value is not greater than the end value
{
// abort the computation
throw new Error("Invalid input arguments");
}
// do the processing
return Math.round(Math.random() * (end - start) + end);
}
That would be my take on this.
Mark VonGyer
21,239 PointsMark VonGyer
21,239 Pointsit's quite inefficient to create these additional numbers and move the storage around.
What might be better is if you wrote the function line twice and changed it.
if(numberX > numberY){
function 1;
}else {
function 2(which is the reverse of function 1); }
It might mean more code but it definitely makes processes faster for the client.