Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial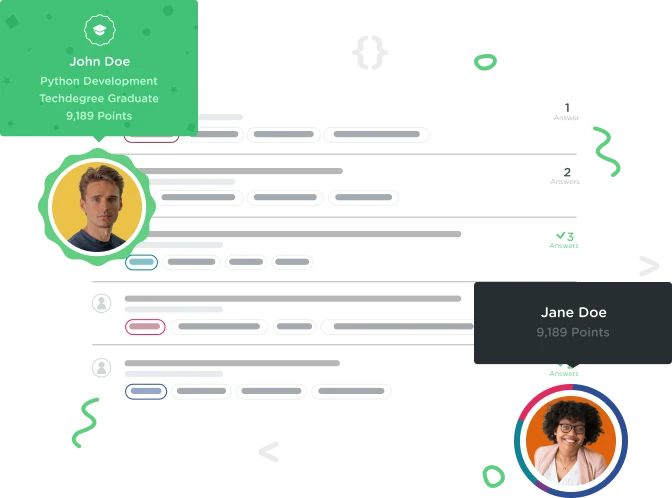

Ezekiel dela Peña
6,231 PointsMy Solution (Feedback is appreciated)
var questions = ["What is the sum of 39 + 46?",
"Who is the first president of the Philippines? (answer should be: FN LN)",
"Is a dog a herbivore, carnivore, or omnivore?",
"What is the answer to the riddle: 'What goes on four feet in the morning, two feet at noon, and three feet in the evening?'",
"What is the name of this website?"];
var answers = [];
var score = 0;
for(var i = 0; i < questions.length; i++) {
// set in a precedence on how they were used.
var answer = prompt(questions[i]);
var valid = false;
var question_number = i;
/*
considering validity of the answers.
*/
do {
if(answer != "" && i != 0) { //if not empty. (questions[1-4]).
answers.push(answer.toLowerCase());
valid = true;
} else if(isNaN(answer) === false && i == 0) { //if a number. (questions[0]).
answers.push(parseInt(answer));
valid = true;
} else {
alert("Invalid answer! Please answer it again!");
answer = prompt(questions[i]);
valid = false;
}
} while(!valid); //until valid.
// counting scores
switch(question_number) {
case 0:
if( answers[i] === 85 ) { score++; } break;
case 1:
if( answers[i] === "emilio aguinaldo") { score++; } break;
case 2:
if( answers[i] === "omnivore") { score++; } break;
case 3:
if( answers[i] === "man") { score++ } break;
case 4:
if( answers[i] === "treehouse") { score++ } break;
}
}
// Awarding Cermony ;)
if( score === questions.length) {
alert("GOLD MEDAL!");
} else if( score === 4 || score === 3) {
alert("SILVER MEDAL!");
} else if( score === 2 || score === 1) {
alert("BRONZE MEDAL!");
} else {
alert("You didn't get anything, Sorry!");
}
2 Answers
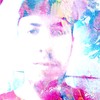
Stoyan Peshev
6,683 PointsWhile the code works, it’s too much code for such a simple task, too many operations. Here is a simple solution:
var questions = ["What is the sum of 39 + 46?",
"Who is the first president of the Philippines? (answer should be: FN LN)",
"Is a dog a herbivore, carnivore, or omnivore?",
"What is the answer to the riddle: 'What goes on four feet in the morning, two feet at noon, and three feet in the evening?'",
"What is the name of this website?"];
var answers = ["85",
"emilio aguinaldo",
"omnivore",
"man",
"treehouse"];
var score = 0;
for (var i = 0, len = questions.length; i < len; i++) {
var answer = prompt(questions[i]).toLowerCase();
if (answer === answers[i]) {
score++;
}
}
// Awarding Cermony ;)
if( score === questions.length) {
alert("GOLD MEDAL!");
} else if( score === 4 || score === 3) {
alert("SILVER MEDAL!");
} else if( score === 2 || score === 1) {
alert("BRONZE MEDAL!");
} else {
alert("You didn't get anything, Sorry!");
}
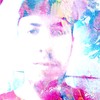
Stoyan Peshev
6,683 PointsWell, there is nothing to validate from the user :) Prompting returns a string - it's either empty or with something in it. Your answers array is also containing strings (yes, even numbers are converted to strings). So you just check if one equals other. :)
For more complex validation you can trim strings, remove excess space etc., but for a simple case like this it's not needed. :)
Ezekiel dela Peña
6,231 PointsEzekiel dela Peña
6,231 PointsThanks for pointing out that I can put the answers in an array totally forgot about that (makes the code more shorter that way).
Though better if still have the validation for a firm response from the user.
Thanks again! :)