Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial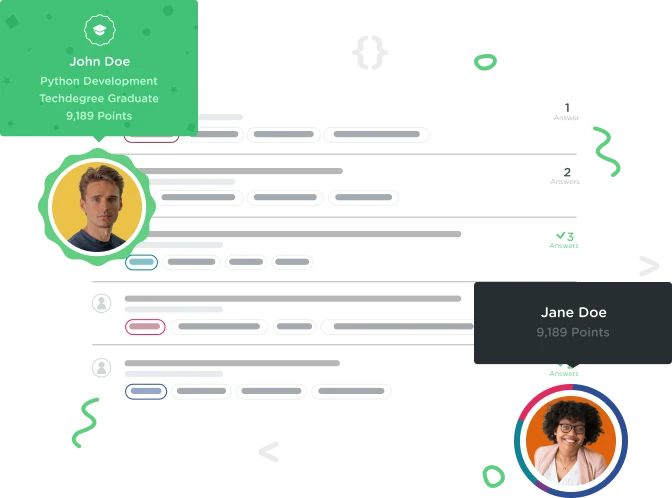

adam åslund
Courses Plus Student 12,184 PointsMy solution for the student record search challenge.
This is how I solved the additional challenges that Dave provided.
var message = '';
var search;
var student;
var noStudent = false; //Use false here so that user can keep searching even after no student was found
function print(message) {
var div = document.getElementById('output');
div.innerHTML = message;
};
function getStudentReport (student) {
var report = '<h2> Student: ' + student.name + '</h2>';
report += '<p> Track: ' + student.track + '</p>';
report += '<p> Achievements: ' + student.achievements + '</p>';
report += '<p> points: ' + student.points + '</p>';
return report;
};
while(true){
search = prompt('Search for students. Type "list" to show all students and "quit" to exit the search.');
if (search === null || search.toLowerCase() === 'quit') {
break;
};
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search.toLowerCase() === student.name.toLowerCase()) {
message += getStudentReport(student); // adding += adds the second getStudentReport after the first instead of overwriting it.
print(message);
noStudent = true;
}
};
if (!noStudent) { // This if statment is to solve the problem if a student dosen't exist.
print('<p> No student named ' + search + '</p>');
};
message =''; // comment this out if you want searches to stay
noStudent = false; //Resets noStudent = false again and the user can keep on searching.
}
Is there any obvious mistakes or improvements to DRY I more than thankful for any input.
2 Answers

Ira Bradley
12,976 PointsVery minor syntax stuff. You don't need to end functions with a semi-colon. Also i += 1 can be represented as i++.

adam åslund
Courses Plus Student 12,184 PointsThanks! I'm not sure why I add the semi-colons, seems to be a habit to avoid forgetting one. I normally use i++ since it comes automatic when you expand a for loop in sublime. But since Dave used it in this course I didn't go back and change it.

Ira Bradley
12,976 PointsYou also can read up on array.reduce() array.filter() and array.map(). I would consider using array.reduce() instead of your for loop. This might help. Let me know if you can't figure it out and I'll post a code example. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/Reduce
Nicholas Woods
6,260 PointsNicholas Woods
6,260 PointsI had trouble outputting 'No student by such name' working in my loop. I know it is more to do with me still coming to grips with the syntax, but it was occasionally throwing up the 'No student' alert for students in the array. So I used a function. I would be interested in what the experts think is a better practice. It is good seeing what other people do.