Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial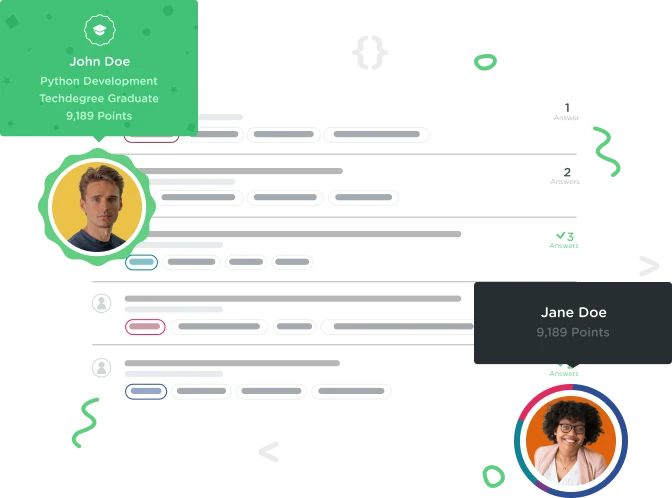
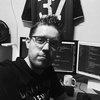
Alexander Khant
Full Stack JavaScript Techdegree Student 17,051 PointsMy solution for two additional problems.
Hi. Here's my solution to a couple of problems that Dave McFarland mentioned in the video.
- Display a message when a student's name isn't found.
- Display all students whose names match. If you're already familiar with Dave's solution for this challenge, it might take just a few lines of code to fix those issues. Just edit the last line of code in the print function like this: outputDiv.innerHTML += message; If necessary, you might want to replace it with document.write(); As for the solution with printing out all students whose names match, within the main for loop add a flag (true) to the main if statement which searches and prints out the student data. Next, outside of the for loop check, if this condition is false, then print out the alert message. Anyway, here's my code:
var nameData = [];
var message = '';
var student;
var search;
var found = false;
function print(message) {
var outputDiv = document.getElementById('output');
// '+= message' fix for printing out students with same names
outputDiv.innerHTML += message;
}
// function to capitalize the first character in a string
String.prototype.capitalize = function() {
return this.charAt(0).toUpperCase() + this.slice(1);
}
// function to print out the search results
function getStudentReport (data) {
var report = '<h2>Student: ' + data.name + '</h2>';
report += '<p>Track: ' + data.track + '</p>';
report += '<p>Achievements: ' + data.achievements + '</p>';
report += '<p>Points: ' + data.points + '</p>';
return report;
}
while (true) {
// .toLowerCase() in case of user inputs capitalized characters
search = prompt("Enter a student's name to display information, or 'quit' to exit.").toLowerCase();
if(search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i+=1) {
// converting all name info in lower case and storing it in a separate array
nameData[i] = students[i].name.toLowerCase();
if (search === null || nameData[i] === search) {
alert('Name: \'' + search.capitalize() + '\' was found.');
message = getStudentReport(students[i]);
print(message);
// setting the flag to check if a name exists in our database.
found = true;
}
}
// if the name is not found, display an alert message
if (!found) {
alert('Name: ' + search.capitalize() + ' was not found.');
}
}
Would appreciate your feedback.

Kuladej Lertkachonsuk
Full Stack JavaScript Techdegree Student 3,175 PointsHello Alexander,
Could you please explain more on editing the "print" function. The one you changed from '=message' into '+=message'.
Why does this solve the problem of the same name?
Thank you very much
1 Answer
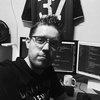
Alexander Khant
Full Stack JavaScript Techdegree Student 17,051 PointsHi, Andre! Thanks for your commentary. You're absolutely right. The "search === null" with the pipe in the second IF statement could be avoided! In fact, my decision was so rushed that I missed a few problems in the code. Perhaps, I was experimenting with something else and forgot to take it out. The main point was to show that I solved it. HOWEVER, even if we throw away the "search === null" and the pipe, then when pressing the escape button, we'll get an error message: "Uncaught TypeError: Cannot read property 'toLowerCase' of null." The solution for this is to move the "toLowerCase()" from here: search = prompt("Enter a student's name to display information, or 'quit' to exit.").toLowerCase(); to the second IF statement like this: if (nameData[i] === search.toLowerCase()) It should work. I hope it helps. Let me know if you have some questions.
Raul Astete
7,805 PointsRaul Astete
7,805 PointsHello ! I was reading all the answers and I found yours. Everything looks good, but I have a question. Why in your second condition with the double pipe "||" Check if "search === null"? I have not found a reason, because supposedly you already did this check line up to make the break. Thanks for your answer!