Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial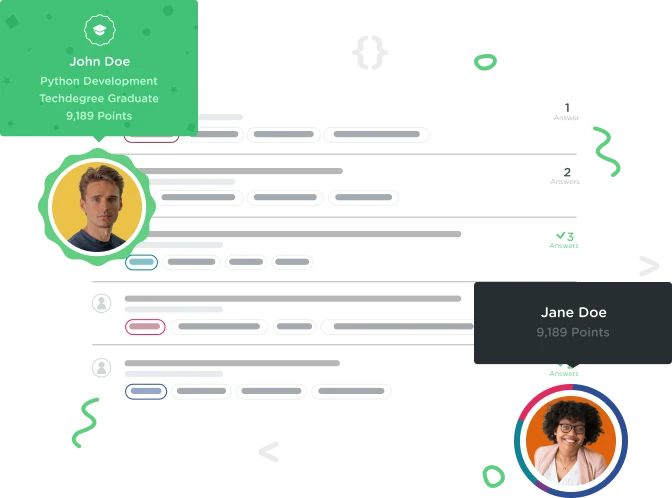

Andrew McPheron
14,290 PointsMy solution is rather different- can someone review it for quality or tips?
I had two major goals while designing this. One was to get better with multidimensional arrays, so I stored a lot of related data in one array. The other was to embrace DRY principles, so I perverted a perfectly good print method into something that didn't make me rewrite code for both the right and wrong categories. I'd say 39 lines and only a few variables is something worth being proud of at least. Any feedback to make it better is appreciated though!
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML += message;
}
//Questions, Answer, Response, Boolean
var quizContent = [
["What is 2 + 2?", "4"],
["How many fingers on two hands?", "10"],
["How many quarts in a gallon?", "4"]
];
var score = 0; //questions correct
for (var i = 0; i < quizContent.length; i++){
quizContent[i].push(prompt(quizContent[i][0])); //Collect repsonses
if (quizContent[i][2] === quizContent[i][1]){ // Interpret responses as booleans
quizContent[i].push(true);
score++;
}
else {
quizContent[i].push(false);
}
}
function quizResults(correct){ //Condensed display to change right or wrong, trying to DRY.
if (correct === true){
var status = 'right!'
}
else if (correct === false){
var status = 'wrong.'
score = 3 - score;
}
print('<br><br> You got ' + score + ' question/s ' + status + '<br><ol>'); //"You got 2 question/s right!"
for (var i = 0; i < quizContent.length; i++){
if (quizContent[i][3] === correct){
print('<li>' + quizContent[i][0] + ' ' + quizContent[i][2] + '</li>'); //Question Answer
}
print('</ol>')
}
}
quizResults(true);
quizResults(false);
2 Answers

Damien Watson
27,419 PointsHey Andrew,
I understand the multi-dimensional arrays thing, so I won't mention object structure over arrays.
Looks pretty clean. Only thing I would do is add a variable for each array position so you don't have to remember what the numbers mean (see my example). There may be more ways to simplify, but I've run out of time. :)
Also, if you're going for smaller code, you can use inline equations (can't remember their real name). It's like an if statement in one line, if you only need to set one item. The break down is something like:
var abc = 10;
var result = (abc < 10)?true:false;
// or
alert ("The result is "+(abc<10?"true":"false"));
function print(message) {
document.getElementById('output').innerHTML += message;
}
//Questions, Answer, Response, Boolean
var QUESTION = 0, ANSWER = 1, RESPONSE = 2, CORRECT = 3;
var quizContent = [
["What is 2 + 2?", "4"],
["How many fingers on two hands?", "8"],
["How many quarts in a gallon?", "4"]
];
var score = 0; //questions correct
for (var i = 0; i < quizContent.length; i++){
quizContent[i].push(prompt(quizContent[i][QUESTION])); //Collect repsonses
quizContent[i].push(quizContent[i][RESPONSE] === quizContent[i][ANSWER]); // Set it here
if (quizContent[i][CORRECT]) score++; // Check it here
}
function quizResults(showCorrect){ //Condensed display to change right or wrong, trying to DRY.
print('<br><br> You got ' + (showCorrect?score:3-score) + ' question/s ' + (showCorrect?'right!':'wrong.') + '<br><ol>'); //"You got 2 question/s right!"
for (var i = 0; i < quizContent.length; i++){
if (quizContent[i][CORRECT] === showCorrect){
print('<li>' + quizContent[i][QUESTION] + ' ' + quizContent[i][RESPONSE] + '</li>'); //Question Answer
}
print('</ol>');
}
}
quizResults(true);
quizResults(false);
But overall, pretty good. :)
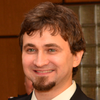
Miroslav Kovac
11,454 PointsHi Andrew, I did not check the functionality, but here are some tips....
*** If you not need the variable - do not create it. Better for memory and performance (of course noi important in some small code like this, but for future...)
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML += message;
}
replace with:
function print(message) {
document.getElementById('output').innerHTML += message;
}
*** You should put functions definitions 'print' and 'quizResults' in one place together, on start or end of file. Is better for code readability, if you do not mix executable code and definitions.
*** Variable 'status'
if (correct === true){
var status = 'right!'
}
else if (correct === false){
var status = 'wrong.'
score = 3 - score;
}
Is better practice to declare variable only once as:
var status;
if (correct === true){
status = 'right!'
}
else if (correct === false){
status = 'wrong.'
score = 3 - score;
}
*** If/else if - If you use boolean - is better to use only if/else and shortened if check
if (correct) {
status = 'right!'
}
else {
status = 'wrong.'
score = 3 - score;
}
*** When you use for-loop on array - maybe is better to user Array.forEach function (But this is more advanced) *** Will be better to use Objects to solve your quiz like an multidimensional array - but seems that you want to do it so, for some training *** Do not forget on semicolons 'var status = 'wrong.' should be 'var status = 'wrong.';'
This is only tips. In any case good job!

Andrew McPheron
14,290 PointsWow, really good eye. The print function is actually code copied from the exercise so I didn't think particularly hard about it. What you have looks right though, so I'll keep that in mind. Declaring outside the conditional statement is something I should have known, glad you caught that and told me.
As for objects, that's the next lesson. Haven't had any exposure to them yet, just wanted to push my knowledge of arrays a bit further.
Thanks for the feedback!
Damien Watson
27,419 PointsDamien Watson
27,419 PointsOh, also. There are only 8 fingers on two hands ( plus two thumbs ;)
Andrew McPheron
14,290 PointsAndrew McPheron
14,290 PointsI like the idea of using variables for the index positions. I was juggling those facts mentally, I don't know why I didn't think to just assign them like that. Thanks for reading over my stuff!