Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial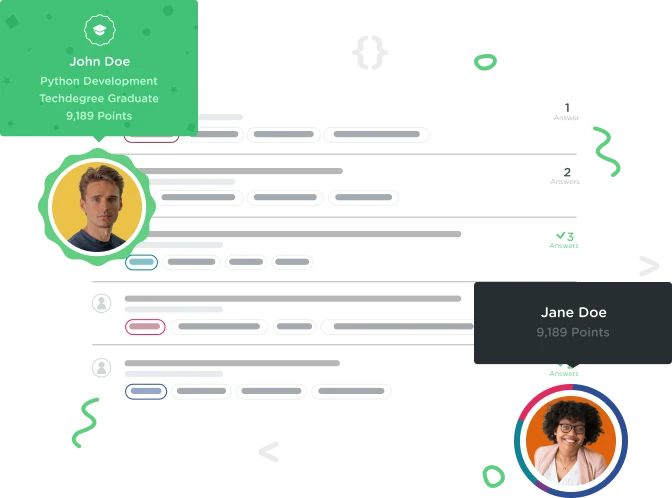
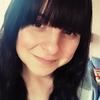
Jess Taylor
5,160 PointsMy solution, pretty pleased with myself! :)
function print(message) {
document.write(message);
};
function searchAgain() {
var choice = prompt('Do you want to search again?');
choice = choice.toLowerCase();
if ( choice === 'yes' || choice === 'y' ) {
return true;
} else {
return false;
};
}
function searchStudents(name) {
for ( i = 0; i < student.length; i++ ) {
if ( name === student[i].name.toLowerCase() ) {
print( displayStudent(i) )
};
}
}
function listStudents() {
for ( i = 0; i < student.length; i++ ) {
print( displayStudent(i) );
}
}
function displayStudent(i) {
var message = "";
message += '<h2>Student: ' + student[i].name + '</h2>';
message += '<p>Track: ' + student[i].track + '</p>';
message += '<p>Achievements: ' + student[i].achievements + '</p>';
message += '<p>Points: ' + student[i].points + '</p>';
return message;
}
var student = [
{ name: 'Neil', track: 'Web Design', achievements: 5, points: 536 },
{ name: 'Jess', track: 'Python', achievements: 15, points: 650 },
{ name: 'Charmain', track: 'iOS', achievements: 7, points: 480 },
{ name: 'Jamie', track: 'Javascript', achievements: 8, points: 585 },
{ name: 'Mary-Jane', track: 'Drawing', achievements: 20, points: 784 },
];
do {
var input = prompt("Search for a name to see if we hold a record, or 'quit' to ummm quit");
if ( input.toLowerCase() === 'quit' ) {
break;
} else {
searchStudents( input.toLowerCase() );
}
} while ( searchAgain() );
Pretty damn happy with myself after this challenge, actually feel like I know what i'm doing :)
Thanks Dave!
3 Answers
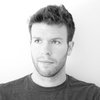
Matthew Rigdon
8,223 PointsThis looks good to me, very organized too :)
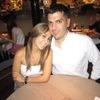
Antonio Jaramillo
15,604 PointsNice stuff. I noticed that if you type anything but 'yes' or 'y', like 'Charmain' for example, in response to your searchAgain() function, your searchAgain() function will return false. Also, instead of using document.write, document.getElementById('output').innerHTML = message would probably work better. It not only makes use of the #output in your HTML, but it also resets what is printed to your HTML upon each individual search.
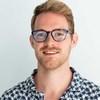
Ryan McCutcheon
10,033 Points//-----------------VARIABLES-----------------
//Nothing seemed to need scope outside the loop especially given that it is the entire program. In fact I wanted variables to reset each loop so limited their scope.
//-----------------FUNCTIONS-----------------
//Used to print items to the output HTML ID in index.html
function print(message) {
document.getElementById('output').innerHTML = message;
}
//used to break the continuous loop by testing user response against 'quit' or 'null'
function stopLoop(response) {
if (response === "null" || response.toLowerCase() === "quit") {
return true;
}
}
//used to determine whether or not the student number exists in the data set
function search(response) {
for ( var i = 0; i < students.length; i++ ) {
if (students[i].number === parseInt(response)) {
return true;
}
}
}
//used to build up HTML message based on student data
function getInfo(response) {
var student = students[parseInt(response)];
for (var prop in student) {
message += "<h3><strong>" + prop + ":</strong> " + student[prop] + "</h3>"
}
return message
}
//-----------------LOOP TO RUN PROGRAM----------------
do {
//Prompt to collect user input with response reseting each pass due to its scope
var response = prompt("Search student records by student number or type 'quit' to exit.");
//message variable scope set to within loop so that it clears each pass
var message = '';
//logical test to match response with student, quit loop or provide feedback on number range
if (search(response) === true) {
print(getInfo(response, students));
} else if (stopLoop(response) === true) {
break;
} else {
alert("There is no student with that number. Press 'ok' and try a number between 1 and " + students.length + ".")
}
} while (true);
Hey Jess, Just wanted to let you know that I really found your solution inspiring so I sat down for an evening and worked at building my own search engine. By borrowing some ideas I think it really made my end product stronger. I went the route of a student number to eliminate issues with duplication and built a more lean HTML compiler (I think haha) for the message. If anyone has feedback on my code I would love to hear it!
And as always thanks Dave, your teaching is awesome and the courseware is top notch!
Ryan