Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial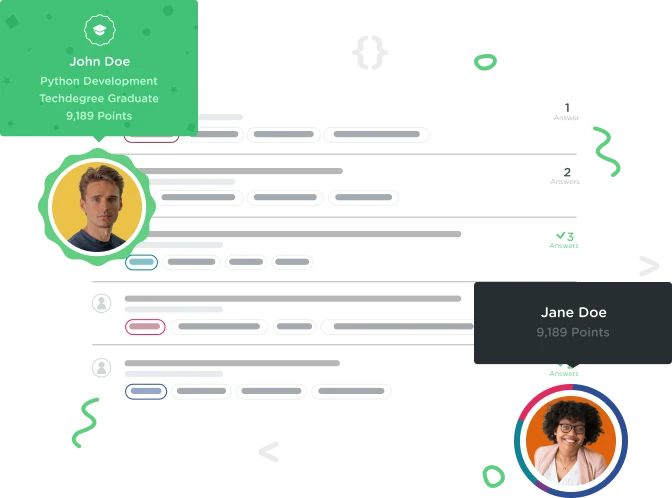
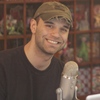
John Yzaguirre
22,025 PointsMy solution. The final run is simplified using functions and logic in a while loop. I made a lot of notes too!
//handy functions
function print(message) {
var targetId = document.getElementById('output');
targetId.innerHTML = message;}
function resetToStart(){
searchResults = [];
resultsFound = false;
}
//-----------------------------
//Place any global variables below
var searchKey;
var searchResults = [];
var resultsFound = false;
//-----------------------------
/* What are we trying to do?
User will 'lookup' the name of a student in the database.
The print out will reflect the results of comparing the User's input with the data or a particular command.
Scenarios will be 'record found' in which 'record presenter' will print a concatenated group of strings and pieces of data.
'quit' will be when the the word "quit" is typed in the prompt, or the User selects the cancel button.
If the User types something in the prompt that is not found in the record and not the string 'quit', then a message will print that '"User input" was not found.'
*/
function askQuestion(){ // Ask a question
searchKey = prompt('Enter a name to search the data base. Type "quit" to quit the search');
}
function lookUp ( someInput ) { // Push any results into a new array called searchResults
for (var i = 0 ; i < students.length; i += 1){
var student = students[i];
if (someInput.toLowerCase() === (student.name).toLowerCase()){
searchResults.push(student)
}
}
}
function checkSearch (someArray) { // Were there any results in the array? True or False?
if (someArray.length > 0){
resultsFound = true;
}
}
function recordPresenter(someArray) {
var html = '';
for ( i = 0; i < someArray.length; i += 1 ) {
var record = someArray[i];
html += '<h2>' + record.name + '</h2>';
html += '<p>' + record.track + '<p>';
html += '<p>' + record.achievements + '<p>';
html += '<p>' + record.points + '<p>';
print(html);
}
}
// loop it and run it!
while (true) {
resetToStart();
askQuestion();
// use conditional here to quit the loop if we type 'quit' or if we select the cancel button
if (searchKey === null || searchKey.toLowerCase() === 'quit') {
break;
}
lookUp(searchKey);
checkSearch(searchResults);
//--------------------------
// User input logic
if (resultsFound === true) {
recordPresenter(searchResults);
} else if (resultsFound === false) {
print('No records found');
}
}
Brian Lane
7,250 PointsBrian Lane
7,250 PointsGreat work, John. Reading your solution really helped me work through the extra credit for myself. Thanks!