Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial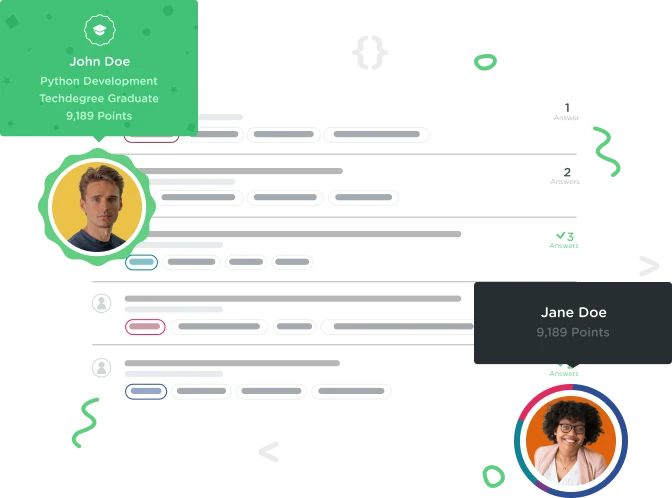

Daniel McElroy
3,277 PointsMy solution to a challenge was very different from the instructor's solution. What is the disadvantage in my version?
In the challenge, you write a quiz with 5 questions and tally the number correct. The instructor wrote the program in a very straight forward fashion with a series of if/else if/else conditions.
I approached mine in a very linear fashion. What is the disadvantage to the way I did it? I understand his way, I just don't know enough about the big picture yet to know why my way is inferior. It would help if someone could explain this to me. Thank you!
var question1 = prompt('What does the dog say?');
var question2 = prompt('What does the cat say?');
var question3 = prompt('What does the cow say?');
var question4 = prompt('What does the sheep say?');
var question5 = prompt('What does the horse say?');
var question1Correct = 'WOOF';
var question2Correct = 'MEOW';
var question3Correct = 'MOO';
var question4Correct = 'BAH';
var question5Correct = 'NEIGH';
var totalPoints = 0;
var question1Point = 0;
var question2Point = 0;
var question3Point = 0;
var question4Point = 0;
var question5Point = 0;
if (question1.toUpperCase() === question1Correct) {question1Point = 1;}
if (question2.toUpperCase() === question2Correct) {question2Point = 1;}
if (question3.toUpperCase() === question3Correct) {question3Point = 1;}
if (question4.toUpperCase() === question4Correct) {question4Point = 1;}
if (question5.toUpperCase() === question5Correct) {question5Point = 1;}
totalPoints = question1Point + question2Point + question3Point + question4Point + question5Point;
if (totalPoints === 0) {document.write("Sorry! You didn't get any correct. Dummy.");
} else if (totalPoints === 1) {document.write("Ouch, you only got 1 correct! Try again.");
} else if (totalPoints === 2) {document.write("Just 2 correct? Are you even trying?");
} else if (totalPoints === 3) {document.write("You got 3 correct, I guess you can have bronze.");
} else if (totalPoints === 4) {document.write("You got 4 correct, silver it is.");
} else {document.write("Hey! You got all 5 correct! You get gold!"); }
3 Answers
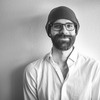
Joseph Fraley
Treehouse Guest TeacherCongratulations on passing the challenge! That's totally worth some cred up front. It definitely takes time basking in JavaScript to appreciate different advantages of one approach over another. In fact, that's one of the hardest, and most rewarding things about JavaScript: there are many, many ways to accomplish the same results.
In general, these are some good-making features of any solution:
- It's easier for a human to understand what the code does when they read it.
- It's shorter (as long as (1) is respected).
- It produces the desired result faster.
- It requires less memory.
There are many other things to strive for when writing good code, but these are probably the most pressing. Up for debate, but the above conditions are probably also in order of importance. The number one most important thing about your code (besides making it produce the effect you want) is that it is clear how it works to a HUMAN reader. Usually that will be YOU in the future, trying to decipher what you did in the past.
Employing these criteria, here are some reasons to prefer the instructor's code over the code you wrote.
- The instructor's is easier to read. For example:
Your code:
var totalPoints = 0;
var question1Point = 0;
var question2Point = 0;
var question3Point = 0;
var question4Point = 0;
var question5Point = 0;
What are all of these variables for? Their names are not clearly legible, they have numbers in the middle, there are a whole bunch of them in a row, they all have the same value. What are they all for? Compare that to the instructor's code:
// quiz begins, no answers correct
var correct = 0;
// ask questions
var answer1 = prompt("Name a programming language that's also a gem");
if ( answer1.toUpperCase() === 'RUBY' ) {
correct += 1;
}
var answer2 = prompt("Name a programming language that's also a snake");
if ( answer2.toUpperCase() === 'PYTHON' ) {
correct += 1;
}
Ok, here we see that there's one variable saving the number of correct answers, and it gets bigger for each correct answer. Crystal clear!
- The instructor's is shorter, without sacrificing legibility. For example:
Your code:
var totalPoints = 0;
var question1Point = 0;
var question2Point = 0;
var question3Point = 0;
var question4Point = 0;
var question5Point = 0;
Don't all of these variables really just describe how many points you have? Why not just have one master points variable that gets bigger when certain conditions are met? Compare that again:
// quiz begins, no answers correct
var correct = 0;
6 lines > 2 lines.
The instructor's code requires less memory. In this case it's not noticeable at all, because the script is so small. But every one of these variable assignments requires a chunk of RAM for its own safekeeping. If you see
var
, that's eating memory. In general, it's good to use less memory if some alternative is equally legible, and performs as well.The instructor's code is faster. It's a big topic about why, but to begin with: his code is short, so there's less code to run, so it's faster. That's not a universal truth, but it's a good rule of thumb for your beginning scripts.
Sometimes programmers refer to "DRY" code. It's an acronym that stands for "Don't Repeat Yourself". DRY code typically respects the four conditions of good code that I described above. You can see that your solution - while it works - isn't very DRY. There are lots of variables being created, when you could get by with many fewer.
The solution you've written is also not easily updated. Suppose you wanted to add other questions to the series. Your solution requires that you add new variables to the code for all of the possible answers and scores. That would be annoying, and it's not easily scalable (it doesn't get infinitely bigger easily). The instructor's code is much easier to append new questions to. It requires fewer changes in the code for any one change in the series. That means less work for you later. That's better.
Does that answer your question? If so, please up-vote my answer as "Best Answer"! It helps me reach my career path goals quickly. If not, feel free to write more!
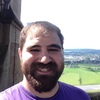
Jamie Perlmutter
10,955 PointsHonestly? It is stream lined, and the code is easy to follow, either way works (I assume your code works) and it is honestly great the it was so different. Everyone codes differently, sure some things come out the same, however for the most part it's all about what you have to do with the code. Your code has each part broken into different section, questions, then points then adding the points, then the out put. His code adds points as it goes and checks it at the end. Your code is good would be easy to figure out what's wrong if there was a problem, because your code is all broken up. His code is short gets to the point. Honestly it's not better or worse, it's just different. If it works and there is no way of breaking the program when it's run, that's what matters. It all depends on what you're doing and what make sense. Does that make sense? There are certain ideas of keeping your code simple so it's not too complicated in case there is a problem or someone else has to work on it. That being said I think the point of the challenges is to allow people to develop their own style of coding. There isn't a right or wrong answer only if it works or doesn't work.

Daniel McElroy
3,277 PointsSure. Thank you for your response. Since I am a novice, I didn't know if I'd get 3 or more courses in deeper and suddenly 'my way' of code writing won't work because new concepts are introduced that make it a bad or impossible approach. If someone with a lot more experience says "I do it this way.", I get a little concerned that it is radically different from my approach.
My version did work, I just wanted to make sure that it wasn't against 'best practices' or that there wasn't a very specific reason he did his that way. Thank you again.
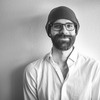
Joseph Fraley
Treehouse Guest TeacherAlso, for future reference, it's much easier for people to help decipher your code in the forums if you use this nifty trick:
You can include blocks of code in a comment by typing
```nameOfCodeLanguage
{block of code}
```
For example:
```javascript
var example = "isn't this easy to read?";
console.log(example); // => "isn't this easy to read?"
```
Looks like this:
var example = "isn't this easy to read?";
console.log(example); // => "isn't this easy to read?"

Daniel McElroy
3,277 PointsThanks, I've edited the original.
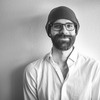
Joseph Fraley
Treehouse Guest TeacherWay to go Daniel! Be consistent about your studies and you'll be well on your way!
Daniel McElroy
3,277 PointsDaniel McElroy
3,277 PointsVery constructive criticism. These points make sense to me. About DRY, he did mention that; he said it would be engaged at a later point. This is exactly why I wanted to get some feedback now, so I don't run into problems later.
I will keep your list of priorities in mind. Thank you for taking the time to respond to me.