Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial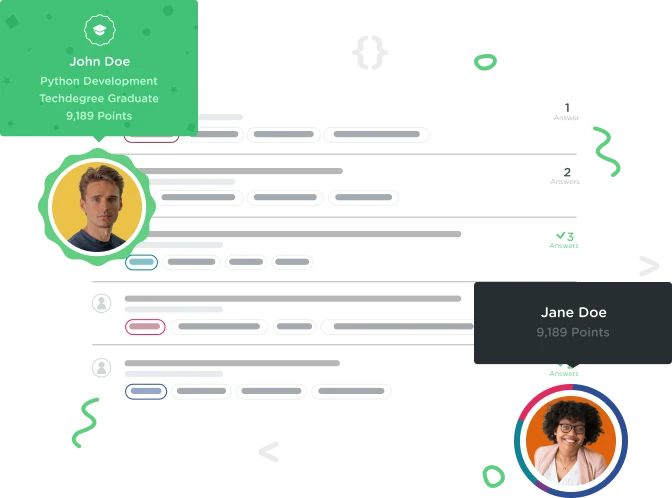
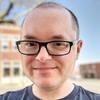
Daniel Beehn
2,991 PointsMy Solution To Dave's Bonus Challenge
...and I've added comments, for those who may be stuck. I think I've optimized this code pretty well, but if anybody has suggestions for how it can be made better, I'm all ears!
// Declare variables.
var htmlStr;
var studentCheck;
var studentQuery;
// Declare function for printing text to "ouput" DIV.
function printHTML(htmlString) {
var htmlOutput = document.getElementById('output');
htmlOutput.innerHTML = htmlString;
}
// And now, the meat of this script...set up an infinite do-while loop.
do {
// Start our "htmlStr" variable with a clean slate, so that each search
// output overwrites the previous one.
htmlStr = " ";
// Set the "studentCheck" variable to "false". This variable is used later
// to trigger our "student not found" message.
studentCheck = false;
// Displays our search prompt and stores the results in the "studentQuery"
// variable.
studentQuery = prompt("Search student records: type a name, or the word 'quit' to exit.");
// Creates an if condition--if the user types "quit", we break out of the
// loop. It's important this is included at the beginning of our do-while
// loop, as if it is missing, the program will otherwise return a "student
// not found error.
if (studentQuery.toLowerCase() === 'quit') {
break;
}
// Creates a for loop that iterates through our array of student objects and
// checks to see if any match the value entered. If so, the "studentCheck"
// variable is set to "true", and that student's info is added to the "htmlStr"
// variable and printed to the "output" DIV.
for (var i = 0; i < students.length; i += 1) {
if (studentQuery === students[i].name) {
studentCheck = true;
htmlStr += "<h2>Student: " + students[i]['name'] + "</h2>";
htmlStr += "<p>Track: " + students[i]['track'] + "</p>";
htmlStr += "<p>Points: " + students[i]['points'] + "</p>";
htmlStr += "<p>Achievements: " + students[i]['achievements'] + "</p>";
printHTML(htmlStr);
}
}
// One final conditional statement-- we check to see if we have iterated through
// our entire array. If we have AND no matches have been found, we add the
// "student not found" message to the "htmlStr" variable and print it to the
// "output" DIV.
if (i >= students.length && studentCheck === false) {
htmlStr = "<h2>That student does not exist.</h2>";
printHTML(htmlStr);
}
// The end of our do-while loop. We set the condition to "true" to make it infinite.
} while (true);
2 Answers
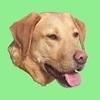
alastair cooper
30,617 PointsVery good.
1 small point.
the statement -- html = ' ' --- at the beginning of your loop is redundant. Instead you could just change the first line of your concatenation steps to read = instead of +=
Sorry if that seems picky, but it's the only point I could find to raise.
Well done on the challenge, and keep going with JavaScript.
Cheers
Alastair
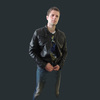
Radu BKR
Full Stack JavaScript Techdegree Student 7,170 PointsHello, I have one question: in you final conditional statement, why are you checking to see if "i >= students.length" ? Isn't one condition enough ? ( ! studentCheck / studentCheck === false ). best of luck, Radu