Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial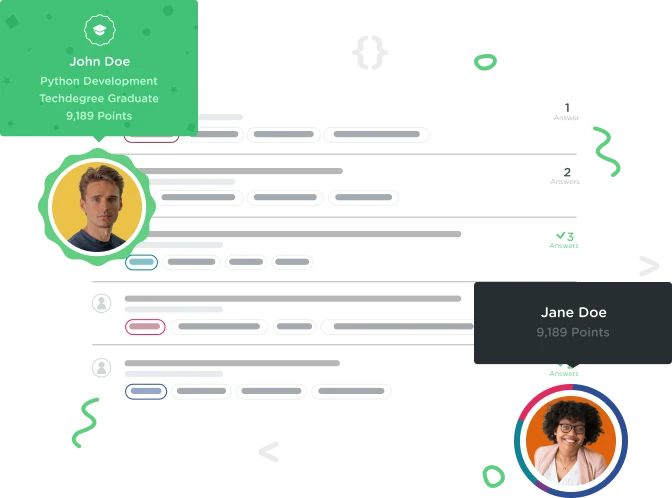
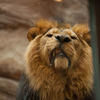
shawn007
15,408 PointsMy solution to the challenge
var message = '';
var student;
var search;
var registor = [];
//To print message to output div.
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
//Bring the registor into html format and stor it into report.
function getStudentReport()
{
var report = '';
for (var m = 0; m < registor.length; m += 1)
{
console.log(search);
report += '<h2>Student: ' + registor[m].name + '</h2>';
report += '<p>Track: ' + registor[m].track + '</p>';
report += '<p>Points: ' + registor[m].points + '</p>';
report += '<p>Achievements: ' + registor[m].achievements + '</p>';
}
return report;
}
// compare will check if any student have the same name.
function compare() {
for (var i = 0; i < students.length; i += 1) {
studentFirst = students[i];
studentFirstName = students[i].name;
studentLower = students[i].name.toLowerCase();
for (var n = 0; n < students.length; n += 1) {
student2 = students[n];
student2Name = students[n].name;
if ((studentFirstName === search || studentLower === search) && studentFirst.ID === student2.ID) {
registor.push(studentFirst);
console.log(registor);
}
}
}
return registor;
console.log(registor)
}
while (true) {
registor = [];
search = prompt('Search student records: Type the name or type Quit to get out.');
compare();
if (search === null || search.toLowerCase() === 'quit') {
break;
} else {
message = '<h2>Not a student</h2>';
print(message);
}
for (var p = 0; p < students.length; p += 1) {
student = students[p];
if (student.name === search || student.name.toLowerCase() === search) {
console.log(search);
message = getStudentReport();
print(message);
}
}
}
7 Answers
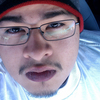
Chyno Deluxe
16,936 PointsOk. so here's how I got it to work.
Original
while (true) {
registor = [];
search = prompt('Search student records: Type the name or type Quit to get out.');
compare();
if (search === null || search.toLowerCase() === 'quit') {
break;
} else {
message = '<h2>Not a student</h2>';
print(message);
}
for (var p = 0; p < students.length; p += 1) {
student = students[p];
if (student.name === search || student.name.toLowerCase() === search) {
console.log(search);
message = getStudentReport();
print(message);
}
}
}
I first started by removing your OR within the conditional if statement., Then I added a .toLowerCase to your initial prompt. and Voila.
Updated JS
while (true) {
registor = [];
search = prompt('Search student records: Type the name or type Quit to get out.').toLowerCase();
compare();
if (search === null || search.toLowerCase() === 'quit') {
break;
} else {
message = '<h2>Not a student</h2>';
print(message);
}
for (var p = 0; p < students.length; p += 1) {
student = students[p];
if (student.name.toLowerCase() === search) {
console.log(search);
message = getStudentReport();
print(message);
}
}
}
any input will be sent to lower case no matter how it is entered and therefore Jody, jOdy and jody will all pull the students records.
I hope this helps.
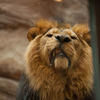
shawn007
15,408 PointsThanks much. So simple.
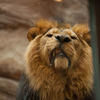
shawn007
15,408 PointsI noticed an error since .toLowerCase() is added to the alert. "Uncaught TypeError: Cannot read property 'toLowerCase' of null" when the prompt is canceled or exited out. Not much of a problem, I think. Can't see the error unless going into inspect element. At least it works.
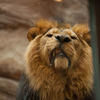
shawn007
15,408 PointsThank you. How did you fix that?
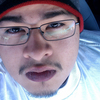
Chyno Deluxe
16,936 PointsPosting code on the forums
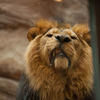
shawn007
15,408 PointsThis is the best I can do so far. I am able to print as many students with the same name. Also if someone were to spell in all lower case it will still output the message. The only thing that will not work right now if someone input JOdy instead of Jody or jody for example. Any ideas on getting irregular case spelling to work?
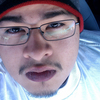
Chyno Deluxe
16,936 PointsCan you post a snapshot of your entire workspace?
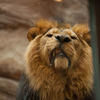
shawn007
15,408 PointsNot sure how to do that?
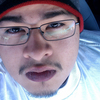
Chyno Deluxe
16,936 PointsTaking a Snapshot of your Workspaces
- Launch any of your workspaces.
- Select the snapshot menu in the upper right corner.
- Click "Take Snapshot"
- Visit the link and share it with someone.
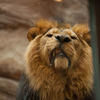
shawn007
15,408 PointsI'm using visual studios for the time being. Trying to get a handle of it while I'm studying. Any idea on how to do the same thing?
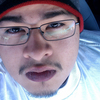
Chyno Deluxe
16,936 PointsYou can post the code on an online code editor like JSFiddle.
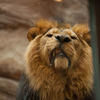
shawn007
15,408 PointsHere is a link on how I got the challenge to work.
https://jsfiddle.net/x4mzen1a/embedded/result/
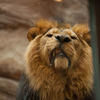
shawn007
15,408 PointsHere shows the code after testing. https://jsfiddle.net/x4mzen1a/
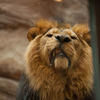
shawn007
15,408 PointsThanks for the info Chyno!

henrihouthoff
33,595 PointsMy solution:
var message;
var student;
var search;
var studentNames = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
studentNames.push(student.name.toLowerCase());
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
message = '';
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name.toLocaleLowerCase() === search ) {
message += getStudentReport ( student );
}
}
print(message);
if (studentNames.indexOf(search.toLowerCase()) === -1) {
print('<p>No student named "' + search + '" was found.</p>');
}
}
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 PointsFixed Code Presentation