Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial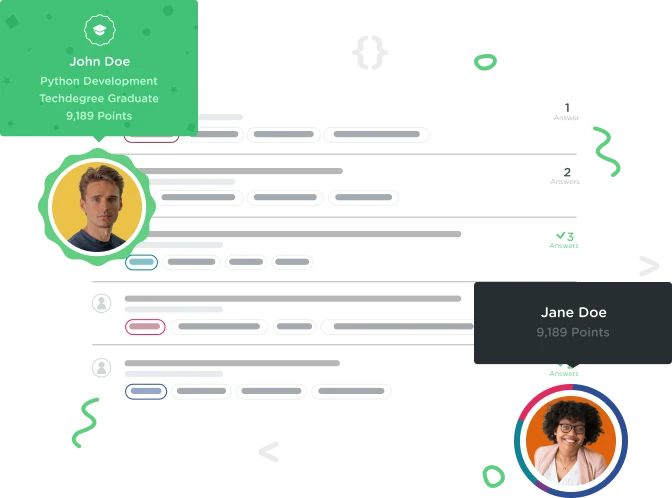

Harcharan Riyait
1,625 PointsMy Solution to the Conditional Challenge
After three hours I finally got it to work to a degree.
The part that has left me stumped is the final message that tells the player that if they scored 0 they receive no crown at all, so I decided to interpret the final requirement as "no message" :-P
The problem was that my starting score was 0 and I increment it by 1 when each question is answered correctly. My else statement states that if the score is 0 then alert("No crowns for you"). However, because the score starts at 0 the "no crowns for you" is displayed at the very end regardless of which crown score the user achieved.
Feedback welcome and appreciated.
Thanks
My script:
//Starting Score var score = 0;
//Questions var question1 = prompt("What is 1+1>"); var answer1 = parseInt(question1); if (answer1 === 2) { score++; } var question2 = prompt("What is 2+2>"); var answer2 = parseInt(question2); if (answer2 === 4) { score++; } var question3 = prompt("What is 3+3>"); var answer3 = parseInt(question3); if (answer3 === 6) { score++; } var question4 = prompt("What is 4+4>"); var answer4 = parseInt(question4); if (answer4 === 8) { score++; } var question5 = prompt("What is 5+5>"); var answer5 = parseInt(question5); if (answer5 === 10) { score++; } //Score Result var scoreMessage = ("Your score is " +score); alert("Score: " + score + " out of 5 questions answered correctly."); //Crown Result if(score === 5) { alert("Congratulations! You earned a Gold Crown!"); } else if (score === 3 || score === 4) { alert("Congratulations! You earned a Silver Crown!"); } else if (score === 1 || score === 2) { alert("Congratulations! You earned a Bronze Crown!"); }
3 Answers
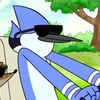
Zachary Fine
10,110 Points///You should define a score variable
var score = 0;
//Any then all the way at the bottom add an else statement if(score === 0)
var question1 = prompt("What is 1+1");
var answer1 = parseInt(question1);
if (answer1 === 2) {
score++;
}
var question2 = prompt("What is 2+2");
var answer2 = parseInt(question2);
if (answer2 === 4) {
score++;
}
var question3 = prompt("What is 3+3");
var answer3 = parseInt(question3);
if (answer3 === 6) {
score++;
}
var question4 = prompt("What is 4+4");
var answer4 = parseInt(question4);
if (answer4 === 8) {
score++;
}
var question5 = prompt("What is 5+5>");
var answer5 = parseInt(question5);
if (answer5 === 10) {
score++;
}
//Score Result
var scoreMessage = ("Your score is " +score);
alert("Score: " + score + " out of 5 questions answered correctly.");
//Crown Result
if(score === 5) {
alert("Congratulations! You earned a Gold Crown!");
} else if (score === 3 || score === 4) {
alert("Congratulations! You earned a Silver Crown!");
} else if (score === 1 || score === 2) {
alert("Congratulations! You earned a Bronze Crown!");
}

Harcharan Riyait
1,625 PointsSorry Zachary, I do have a score variable at the start of the code, I missed it out when pasting the code into the post (it was late).
I tried using an else variable if the score is 0, but the "0 Crowns" message pops up after the correct crown reward. TI understand this is happening because the score starts at 0 and the code waits for all the previous operations to run.

Harcharan Riyait
1,625 PointsOK. I was trying to end the conditional argument by using "else", but I just used "else if" and now it works. The 0 crowns message only pops up if no points are scored. Thanks Zachary for suggesting the "else if"
BTW: How do you get your code to present in the post like that? Mine just goes in like it's been minified?
Code:
//Starting Score var score = 0;
//Questions var question1 = prompt("What is 1+1>"); var answer1 = parseInt(question1); if (answer1 === 2) { score++; } var question2 = prompt("What is 2+2>"); var answer2 = parseInt(question2); if (answer2 === 4) { score++; } var question3 = prompt("What is 3+3>"); var answer3 = parseInt(question3); if (answer3 === 6) { score++; } var question4 = prompt("What is 4+4>"); var answer4 = parseInt(question4); if (answer4 === 8) { score++; } var question5 = prompt("What is 5+5>"); var answer5 = parseInt(question5); if (answer5 === 10) { score++; } //Score Result var scoreMessage = ("Your score is " +score); alert("Score: " + score + " out of 5 questions answered correctly."); //Crown Result if(score === 5) { alert("Congratulations! You earned a Gold Crown!"); } else if (score === 3 || score === 4) { alert("Congratulations! You earned a Silver Crown!"); } else if (score === 1 || score === 2) { alert("Congratulations! You earned a Bronze Crown!"); } else if (score===0){ alert("You didn't earn any Crowns!"); }