Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial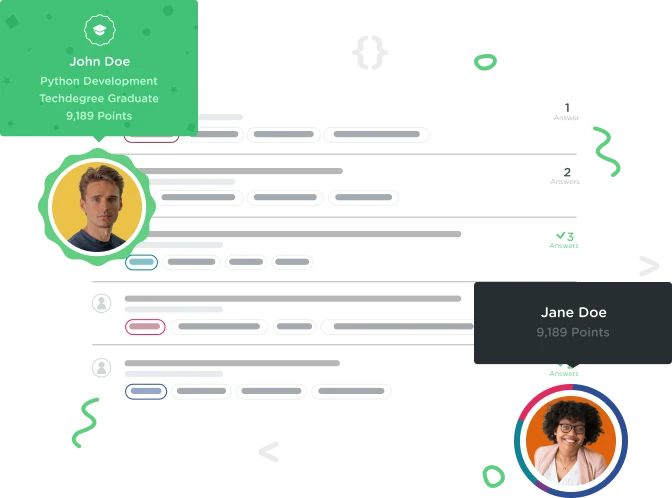

Fidel Severino
3,514 PointsMy solution to the Conditional Challenge Quiz. Looking for feedback.
Hello, I just finished the challenge and have seen the instructor's solution. I see where his code is an improvement over mine, but I am still looking for feedback regarding code legibility or basically anything that can be pointed out.
For example, I knew while writing my code, that I could use the .toUpperCase()
method inside of the condition for the if statements. However, I felt doing it the way I did it would increase readability.
/*
The Conditional Challenge Quiz
1. Ask at least five questions.
2. Keep track of the number of
questions the user answered correctly.
3. Provide a final message after the quiz letting the
user know the number of questions he/she got right.
4. Rank the player:
5 out of 5 = Gold
3-4 out of 5 = Silver
1-2 out of 5 = Bronze
0 out of 5 = No prize
*/
// ask the user 5 questions
var question1 = prompt('What is Spiderman\'s real full name?'),
question2 = prompt('What insect gave Spiderman his powers?'),
question3 = prompt('Who is Spiderman secretly in love with?'),
question4 = prompt('What Spiderman archnemesis has a black suit similar to Spiderman\'s?'),
question5 = prompt('What does Peter Parker work as for the Daily Bugle?');
// set all answers to uppercase
question1 = question1.toUpperCase();
question2 = question2.toUpperCase();
question3 = question3.toUpperCase();
question4 = question4.toUpperCase();
question5 = question5.toUpperCase();
// variable to keep score
var score = 0;
// when an answer is correct increase SCORE by 1
if (question1 === 'PETER PARKER') {
score += 1;
} else {
alert('Wrong answer! Better luck on the next question.');
}
if (question2 === 'RADIOACTIVE SPIDER') {
score += 1;
} else {
alert('Wrong answer! OOPS!');
}
if (question3 === 'MARY JANE') {
score += 1;
} else {
alert('Wrong answer! Really???');
}
if (question4 === 'VENOM') {
score += 1;
} else {
alert('Wrong answer! C\'mon! You can do better than that...');
}
if (question5 === 'PHOTOGRAPHER') {
score += 1;
} else {
alert('Wrong answer! I don\'t want to hear it!');
}
// test to see what prize to give depending on the number of correct answers
if (score === 5) {
document.write('<p>Amazing! You got all 5 questions correct. For that, you get a GOLD medal.</p>');
} else if (score === 4) {
document.write('<p>Congratulations! You got 4 out of 5! You scored the SILVER medal.</p>');
} else if (score === 3) {
document.write('<p>Not bad! You got 3 out of 5! You scored the SILVER medal.</p>');
} else if (score === 2) {
document.write('<p>Um, OK... You only got 2 out of 5! You\'re lucky to be getting a BRONZE medal.</p>');
} else if (score === 1) {
document.write('<p>Abismal performance. You got 1 out of 5! I don\'t even know why but you still get a BRONZE medal.</p>');
} else if (score === 0) {
document.write('<p> You got none of the questions right. You get NO PRIZE.</p>');
}
2 Answers
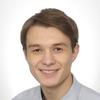
Sergey Podgornyy
20,660 PointsThe best way to perform this task is create an array with questions and answers, and then iterate through this array:
var questions = [
{
question: 'How many states are in the United States?',
answer: 50
},
{
question: 'How many continents are there?',
answer: 7
},
{
question: 'How many legs does an insect have?',
answer: 6
}
];
var correctAnswers = 0,
response;
function print(message) {
document.write(message);
}
for (var i = 0; i < questions.length; i += 1) {
response = parseInt(prompt(questions[i].question));
if (response === questions[i].answer) {
correctAnswers++;
}
}
html = "You got " + correctAnswers + " question(s) right."
print(html);

Mohamed Ali
10,010 Pointshow about this
var playerRank = 0;
var questions = 5;
var questionsLeft = '[' + questions + 'questionsLeft]';
var qestion1 = prompt('what is 1+1 ?' + questionsLeft);
if (parseInt(qestion1) === 2) {
playerRank +=1;
}
questions -= 1
questionsLeft = '[' + questions + 'questionsLeft]';
var qestion2 = prompt('how many hours in a day ?' + questionsLeft);
if (parseInt(qestion2) === 24) {
playerRank +=1;
}
questions -= 1
questionsLeft = '[' + questions + 'questionsLeft]';
var qestion3 = prompt('who founded facebook ?' + questionsLeft);
if (qestion3.toUpperCase() === 'MARK') {
playerRank +=1;
}
questions -= 1
questionsLeft = '[' + questions + 'questionsLeft]';
var qestion4 = prompt('who is the presedent of America ?' + questionsLeft);
if (qestion4.toUpperCase() === 'OBAMA') {
playerRank +=1;
}
questions -= 1
questionsLeft = '[' + questions + 'questionsLeft]';
var qestion5 = prompt('What is the name of your teacher javascript ?' + questionsLeft);
if (qestion5.toUpperCase() === 'DAVA') {
playerRank +=1;
}
alert('Are you ready to see your answer');
if (playerRank === 5) {
document.write('<p><strong> You earned a gold crown!</strong></p>');
} else if (playerRank >=3 ) {
document.write('<p><strong> You earned a silver crown!</strong></p>');
} else if (playerRank >=1) {
document.write('<p><strong> You earned a bronze crown!</strong></p>');
} else {
document.write('<p><strong> No crown for you. you need to study </strong></p>');
}
Sergey Podgornyy
20,660 PointsSergey Podgornyy
20,660 PointsIn your code you can simplify it adding .toUpperCase direct to your question variables:
var question1 = prompt('What is Spiderman\'s real full name?').toUpperCase()
And you need to alert message after prompt question:
For this you need to create function. Or iterate questions as I wrote you above.
At the end, you can print message using switch-case:
Fidel Severino
3,514 PointsFidel Severino
3,514 PointsAt this point in the Front-End track he hasn't introduced arrays, so while that code might be a better answer, it is one I couldn't code at this point in my learning.
Thanks for the answer though! Will review it once I learn more about arrays and objects.