Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial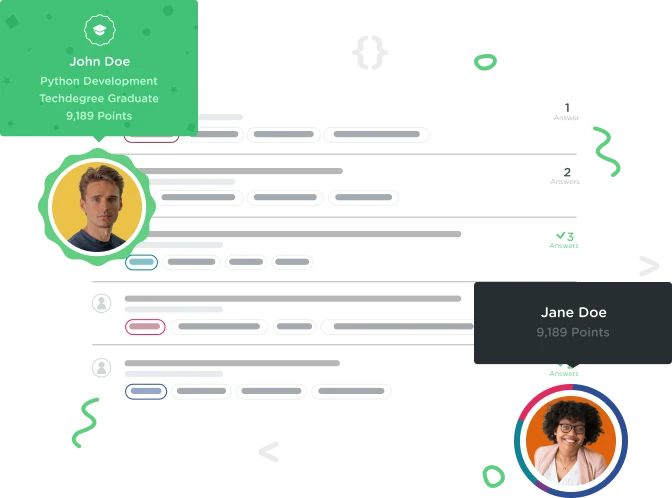

davidtodd2
5,590 PointsMy solution to the last things he says in the video
Idk, seems about as efficient as I can make it with what's been presented so far and without wracking my brain for hours.
//Declaring the variables and functions at the beginning
var student;
var quit = true;
var search = '';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
//The main, useful function I made to iterate
//through the array and its objects.
function query (students, search) {
/*declaring the variable in the function
but before the for loop makes it so that
it is blank when you search for another name
but doesn't erase if there are multiple people
with the same name, like in what he said towards
the end of the video.. Funny enough I got that
part right by coincidence.
*/
var message = '';
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search.toUpperCase() === student.name.toUpperCase()) {
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
}
}
//If message is blank then it means no student
//was found, so this informs the user of that.
if (message === '' && search !== null && search.toUpperCase() !== 'QUIT') {
message = "Sorry, no student by that name was found.";
}
return message
}
/*The do while loop just performs the
search prompt while making sure to have a
way to exit the loop and also prints the results of the search.
*/
do {
search = prompt("What is the student's name?");
if ( search === null || search.toUpperCase() === 'QUIT') {
quit = false;
}
print(query(students, search));
} while ( quit === true );

John Alexander
2,617 PointsWhy do you declare two arguments students and search for the function?
'''function query (students, search)'''
David Laycock
2,988 PointsDavid Laycock
2,988 PointsWow, that was incredibly simple. Here I am trying to create complicated secondary functions to make it work when all I had to do was change the scope of a variable (or clear the variable after the while loop but before the for loop). Great solution.