Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial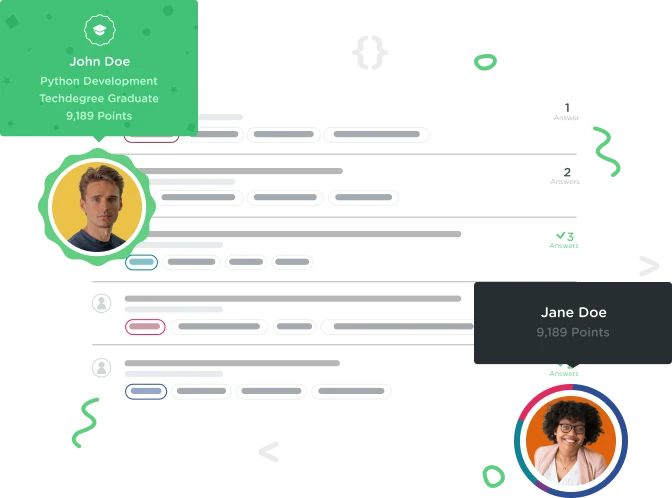

Joe Hartman
20,881 PointsMy solution to the quiz exercise (feedback requested)
Here's a link to my solution for the challenge. Any feedback would be welcome.
5 Answers
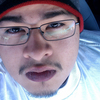
Chyno Deluxe
16,936 PointsYour code works but I would suggest doing a couple things.
Try to D.R.Y(Don't Repeat Yourself) up your code by using an array to hold the questions AND answers.
var quesAns = [
[question, answer],
[question, answer]
];
for(var i = 0; i < quesAns.length; i++){
//ASK QUESTIONS
var ask = prompt(quesAns[i][0]);
//DO MORE STUFF
}
Second, I would suggest changing the answers to all uppercase or lowercase and making sure you change the users input to which ever case you chose so that no matter how they answer a question (i.e Dream, DREAM, dream) that there answers still be considered correct.
I hope this help. Keep Coding!

Joe Hartman
20,881 PointsOK, thanks. Trying this again https://w.trhou.se/zcyzpjwctc

Joe Hartman
20,881 PointsThank you. As the lesson was on conditionals that was what I used but a looping structure would be less code. It didn't occur to me to use changing case in the test, which would have provided more flexibility for the user. I was thinking it would be obvious from the questions how to spell the response. I need to make a note to myself to avoid assumptions like this as much as possible.
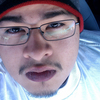
Chyno Deluxe
16,936 PointsHaha yes we always have to keep simple in mind when writing code. Users somehow always find a way to break something haha so we have to try our best to test every possibility.
If you decide and try to update your code and need some help, feel free to ask.

Gunhoo Yoon
5,027 PointsAt this stage there's not much things to tackle with (You haven't learned function declaration, loop, and many.... yet).
You will get a chance to use them to this same question in near future.
So I'll just post how I would wrote using what you've learned, except for 1~2 aspects.
// <- this is syntax for commenting, this code will not run.
//From now I'll communicate with comment.
//Questions
//This is just subjective thing about organizing code,
//you don't have to care for change made to this.
//Also, I used only one var to group questions. This is okay but
//generally not welcome in TreeHouse.
var question1 = prompt("What year did Columbus arrive in the Western Hemisphere?"),
question2 = prompt("How many moons does Mars have?"),
question3 = prompt("Fill in the blank: ____ Sagan, author of Cosmos."),
question4 = prompt("Fill in the blank: Lake _____ is one of the Great Lakes."),
question5 = prompt("Fill in the blank: Martin Luther King said \"I had a ______\".");
//Score counter
var score = 0;
//Grading
//Changed grader to ignore case-sensitivity to give some forgiveness.
if (parseInt(question1, 10) === 1492) { //parseInt(n, 10) makes sure it returns decimal.
score += 1;
}
if (parseInt(question2, 10) === 2) {
score += 1;
}
if (question3.toLowerCase() === "carl") { //toLowerCase() transforms text to lowercase.
score += 1;
}
//You can either apply toLowerCase() to all case or
//just make this an exception and save it as variable.
//Also, I'd like to separate each comparison in groups of 1 or 2.
var answer = question4.toLowerCase();
if (answer === "michigan" ||
answer === "erie" ||
answer === "superior" ||
answer === "huron" ||
answer === "ontario") {
score += 1;
}
if (question5.toLowerCase() === "dream") {
score += 1;
}
//General feedback template
//Extracted common part of feedback to save up few typing and for future maintainability.
var feedback = "<p>Congratulations, you answered " + score + " questions correctly.";
//Give feedback
//4 indent space is preferred but I like to use 2.
if (score === 5) {
document.write(feedback + 'You have earned a gold crown!</p>');
} else if (score >= 3) { //Changed this from score == 4 || score == 3
document.write(feedback + 'You have earned a silver crown!</p>');
} else if (score >= 1) { //Changed this from score == 2 || score == 1
document.write(feedback + 'You have earned a bronze crown!</p>');
} else {
document.write("<p>You answered all questions incorrectly. Please go sit in the corner.</p>");
}

Joe Hartman
20,881 PointsThanks for the tip in the final code block. That does shorten it up.

Gunhoo Yoon
5,027 PointsYeah I found most valuable lesson from this exercise is to realize that I hate typing.
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 PointsThe link you provided is a direct link, which will not work for anyone but you. You can take a snapshot of your workspace by following the instructions below.
Workspaces Snapshot