Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial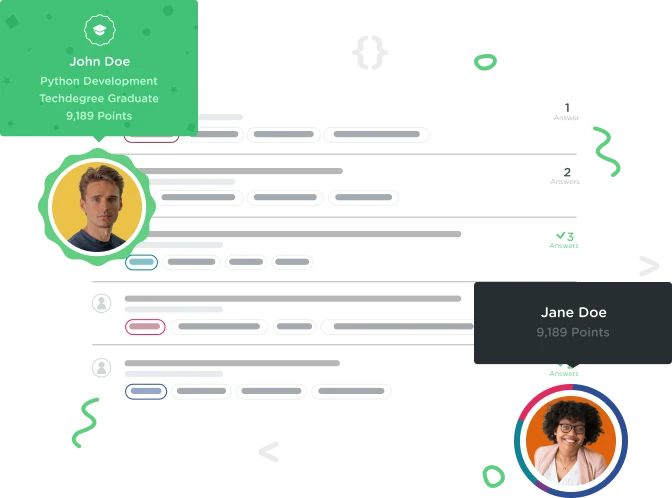

Andrew Chung
Courses Plus Student 4,094 PointsMy solution w/ extra credit (feedback/suggestions)
I added another student named Trish to the students array and decided to keep all of the JS code for this challenge in the students.js file. Would really appreciate any feedback and suggestions for improvement. Thanks all!
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
},
{
name: 'Trish',
track: 'Learn WordPress',
achievements: '2',
points: '195'
}
];
function print(message) {
var wrapper = document.getElementById('output');
wrapper.innerHTML = message;
}
function siteSearch() {
while (true) {
var searchQuery = prompt('Search for students by name. Type "quit" at anytime to exit the program.');
if (searchQuery === 'quit') {
break;
}
else {
findStudent(searchQuery);
}
}
}
function findStudent(name) {
/*
Loop through students array and compare name parameter against each student object's name value.
Matched students are placed in the foundStudents array, which is sent as an argument
to the createStudentProfiles function.
*/
foundStudents = [];
for (var i = 0; i < students.length; i++) {
student = students[i];
if (name === student.name) {
foundStudents.push(student);
}
}
if (foundStudents.length > 0) {
createStudentProfiles(foundStudents);
}
else {
alert(name + ' is not in our student directory.');
}
}
function createStudentProfiles(foundStudents) {
var studentProfilesHTML = '';
for (var i = 0; i < foundStudents.length; i ++) {
student = foundStudents[i];
var profileHeading = '<h2>Student: ' + student.name + '</h2>';
var profileBody = '<p>Track: ' + student.track + '</p>' +
'<p>Points: ' + student.points + '</p>' +
'<p>Achievements: ' + student.achievements + '</p>';
studentProfilesHTML += profileHeading + profileBody;
}
print(studentProfilesHTML);
}
//Begin program.
siteSearch();
5 Answers
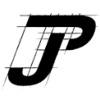
Jeff Pierce
18,077 PointsHi Andrew,
Your code is very clean and easy to follow. I have one suggestion:
// Test for the cancel button if (searchQuery == null || searchQuery === 'quit') {

Andrew Chung
Courses Plus Student 4,094 PointsThanks for your feedback and suggestion Jeff! It makes sense that clicking cancel would return a null value, but it didn't quite click until I read your comment.
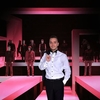
Joe Sleiman
5,921 Pointssorry how can i put my solution as this way... i tried and didn't match

Ary de Oliveira
28,298 Pointslet test your code

Ary de Oliveira
28,298 PointsCool i like...