Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial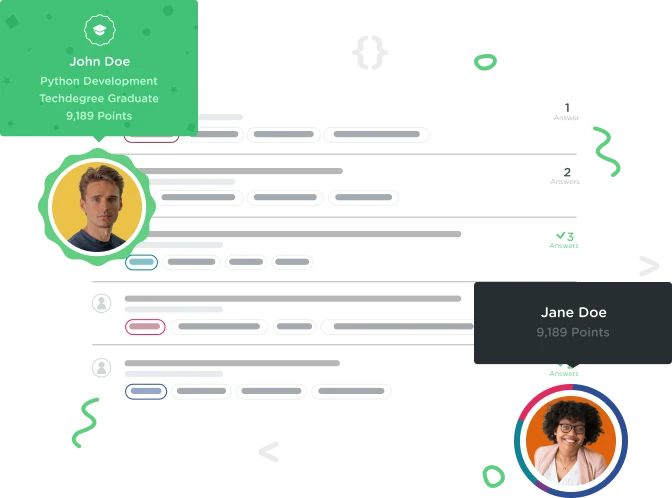
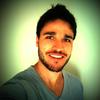
Marcio Mello
7,861 PointsMy solution - why .push not working. Could fix only with .concat
Whenever i try replacing .concat with .push it gives me an error saying: Push method does not exist. Why?
My code is as follows:
// VARIABLES
var answer;
var correctAnswers = [];
var wrongAnswers = [];
var questions = [
["How many paws a dog has?", "4"],
["What is the name of our planet?", "earth"],
["Who is de president of the United States?", "obama"]
];
// FUNCTIONS
function print(message) {
document.write(message);
}
function createHtmlList( list ) {
var htmlSnipet = "<ol>"
for ( i = 0; i < list.length; i++ ) {
htmlSnipet += "<li>" + list[i] + "</li>";
}
htmlSnipet += "</ol>";
return htmlSnipet;
}
// IMPLEMENTATION
for ( i = 0; i < questions.length; i++ ) {
answer = prompt(questions[i][0]);
if ( answer.toLowerCase() === questions[i][1] ) {
correctAnswers = correctAnswers.concat(questions[i][0]);
} else {
wrongAnswers = wrongAnswers.concat(questions[i][0]);
}
}
print("<h2>You have got " + correctAnswers.length + " out of 3 correct answers!</h2>")
print("<h3>Correct Answers</h3>");
print(createHtmlList(correctAnswers));
print("<h3>Wrong Answers</h3>");
print(createHtmlList(wrongAnswers));
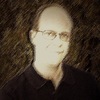
Jason Anders
Treehouse Moderator 145,860 PointsMOD note: added javascript
declaration after the back ticks to make code more readable.
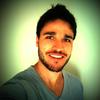
Marcio Mello
7,861 PointsThanks. I was having a hard time trying to make it readable. Guess I did not have to add the extras return key.
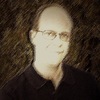
Jason Anders
Treehouse Moderator 145,860 PointsYou did everything right, you just missed adding the language immediately after the the opening back ticks (three ticks ... no space ... programming language). Other than that, the formatting was correct.
:)
2 Answers
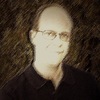
Jason Anders
Treehouse Moderator 145,860 PointsHi Marcio,
When you are using the push method, you don't want to reassign the value back into the variable. The way you have it set up, the compiler is trying to take a value and reassign back into the variable instead of appending it to your array.
When you have correctAnswers = correctAnswers.concat(questions[i][0]);
JavaScript is interpreting this as you wanting the value of what is after the = sign to be assigned as the new value to the variable (array) correctAnswers. That is why push was being interpreted as a function and not a method.
Instead, you just want the method to be run on the Array name by itself:
for ( i = 0; i < questions.length; i++ ) {
answer = prompt(questions[i][0]);
if ( answer.toLowerCase() === questions[i][1] ) {
correctAnswers.push(questions[i][0]); //deleted correctAnswers = and changed to push
} else {
wrongAnswers.push(questions[i][0]); //deleted wrongAnswers = and changed to push
}
}
Hope this helps and makes sense. Keep Coding! :)
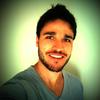
Marcio Mello
7,861 PointsOh! Right! Thank you very much Jason! ;)
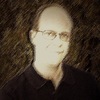
Jason Anders
Treehouse Moderator 145,860 PointsYou're welcome!
Chad Donohue
5,657 PointsChad Donohue
5,657 PointsTry surrounding your code with three(3) back ticks (`), before and after your code.
This would help others to view your code and understand the problem.