Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial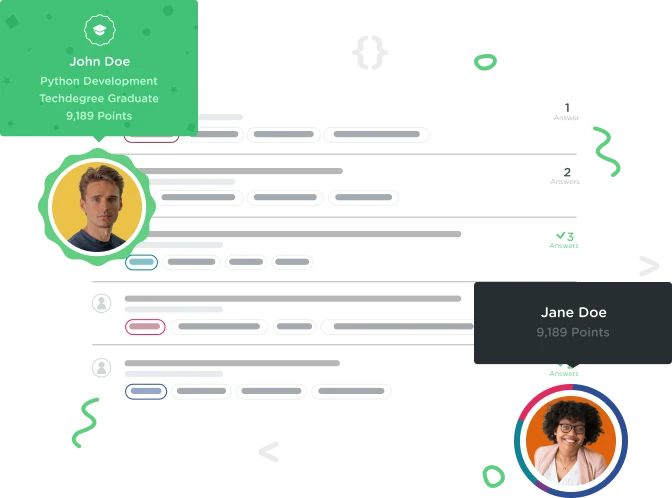
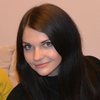
Alisa Trunczik
3,659 PointsMy solution (without .push method)
Hello, everyone!
I've tried to add a third item to existing 2-dimens. array we had. Here is my solution:
var quizData = [
["Which planet is nearest the sun?", "MERCURY"],
["What is the singular of Scampi ?", "SCAMPO"],
["What is bottled a lot in the French town Vichy?", "WATER"]
];
var question;
var answersCorrect = 0;
var userAnswer;
var answer;
var html;
function print(message) {
document.write(message);
}
for (var i = 0; i < quizData.length; i += 1) {
question = quizData[i][0];
answer = quizData[i][1];
userAnswer = prompt(question);
quizData[i][2] = userAnswer.toUpperCase() === answer;
}
print("<h1> You got these question(s) CORRECT:</h1>");
for (var j = 0; j < quizData.length; j += 1) {
if (quizData[j][2]) {
answersCorrect += 1;
print("<h4>" + quizData[j][0] + "</h4>");
print("<h2>The correct answer is: "+ quizData[j][1] + "</h2>");
}
}
print("<h1> You got these question(s) WRONG:</h1>");
for (var j = 0; j < quizData.length; j += 1) {
if (!quizData[j][2]) {
print("<h4>" + quizData[j][0] + "</h4>");
print("<h2>The correct answer was: "+ quizData[j][1] + "</h2>");
}
}
print("<h1> You got " + answersCorrect + " question(s) correct!</h1>");
I also started to refactor this code by adding a function to print results to make it shorter.
Is my solution good? What's better to use?
Thanks!
1 Answer

Gunhoo Yoon
5,027 PointsGreat job Alisa it's a great mind-set to have to make your version of code and trying to enhance it.
Right now your code has little place for refactoring, based on what you've learned. Once you start to learn more you will find different approach to solve this problem.
Here is something to consider.
Removing html variable which is unused.
Adding comments to your own code.
Here is something you can challenge yourself.
Summarizing your code into human language.
Finding different approach that will do same or similar thing.
Try to write shorter code with minimal loop. (This is easily done as you learn more)
Keep improving this code by implementing what you will learn in the future.
I will add my version of your code when I get some time.
Keep up the good work!
Alisa Trunczik
3,659 PointsAlisa Trunczik
3,659 PointsWow, Gunhoo Yoon, thank you so much for useful tipps and approaches, I will try them all and learn more:)
Sometimes I think it's just so hard for me to be creative with refactoring, but I guess it comes with practice.
Gunhoo Yoon
5,027 PointsGunhoo Yoon
5,027 PointsHere's my version and new vocabulary for you which you will need to create your own HTML element or existing HTML element.
You only need these two vocabulary to understand it.
document.createElement('tagName')
Creates HTML element.node.appendChild(element)
append child element to node. Node refers to html element.This code seems needlessly long because of all the interaction with HTML elements(about 25% + space for comment). But what it tries to do is build a structured content rather than rendering content on every iteration. No one does this sort of madness but this is basic way of JavaScript interacting with HTML element.