Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial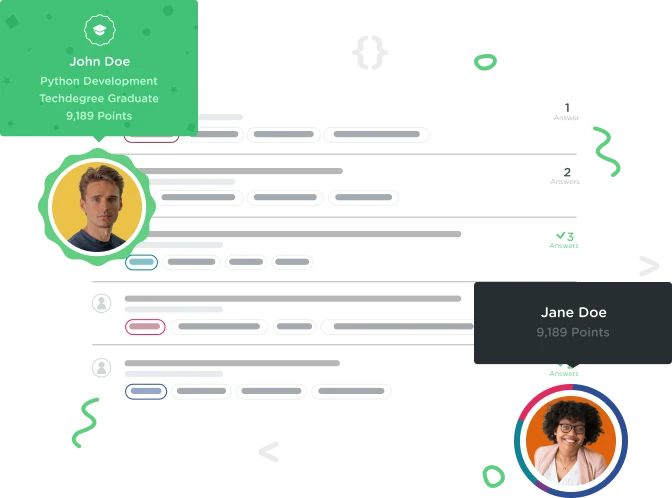
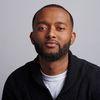
yahya hussein
3,697 PointsMy Solution(feedback please. is it too WET for what we have learned so far?)
My code works as far as I know. I was wondering if I could make it more dry or if it was good enough for what we have learned so far?
var correctAnswer = "You got the answer right";
var wrongAnswer = "Sorry that was the wrong answer";
var questionsRemaining = 5;
var points = 0;
var questionOne = prompt("How many states are in the US");
if (parseInt(questionOne) === 51){
points += 20;
questionsRemaining -= 1;
alert(correctAnswer + " You have " + questionsRemaining + " questions remaining");
}else{
alert(wrongAnswer + " You have " + questionsRemaining + " questions remaining");
}
var questionTwo = prompt("When did Michael Jackson die?");
if (parseInt(questionTwo) === 2009){
points += 20;
questionsRemaining -= 1;
alert(correctAnswer + " You have " + questionsRemaining + " questions remaining");
}else{
alert(wrongAnswer + " You have " + questionsRemaining + " questions remaining");
}
var questionThree = prompt("How many rings does Michael Jordan have?");
if (parseInt(questionThree) === 6){
points += 20;
questionsRemaining -= 1;
alert(correctAnswer + " You have " + questionsRemaining + " questions remaining");
}else{
alert(wrongAnswer + " You have " + questionsRemaining + " questions remaining");
}
var questionFour = prompt("Who is the best player in the NBA?");
if (questionFour.toUpperCase() === "LEBRON JAMES" || questionFour.toUpperCase === "STEPHEN CURRY"){
points += 20;
questionsRemaining -= 1;
alert(correctAnswer + " You have " + questionsRemaining + " questions remaining");
}else{
alert(wrongAnswer + " You have " + questionsRemaining + " questions remaining");
}
var questionFive = prompt("Where is the best place to learn the basics of coding?")
if (questionFive.toUpperCase() === "TEAM TREEHOUSE"){
points += 20;
alert(correctAnswer);
}else{
alert(wrongAnswer);
}
alert("You got " + points + "% correct");
// Medals Earned
var goldMedal = "You earned the gold medal";
var silverMedal = "You earned the silver medal";
var bronzeMedal = "You earned the silver medal";
if(points > 90) {
alert(goldMedal);
}else if(points > 70){
alert(silverMedal);
}else if (points > 50){
alert(bronzeMedal);
}else {
alert("Sorry you didn't win anything, maybe next time");
}
1 Answer
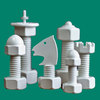
Steven Parker
231,236 PointsThis is perhaps a bit verbose, but probably just fine for a simplified case like this (except you probably want to reduce the count of questions remaining whether the answer is right or not). But if you were going to have a large number of questions, it would be helpful to put the logic into a function you could call, something like this:
function doquestion(question, answer) {
var message = "";
var response = prompt(question);
if (response.toUpperCase() === answer) {
points += 20;
message = correctAnswer;
} else {
message = wrongAnswer;
}
if (--questionsRemaining > 0) {
message += " You have " + questionsRemaining + " questions remaining";
}
alert(message);
}
And then the test body would be much more compact, like this:
doquestion("How many states are in the US", "50");
doquestion("What year did Michael Jackson die?", "2009");
doquestion("How many rings does Michael Jordan have?", "6");
doquestion("Where is the best place to learn the basics of coding?", "TEAM TREEHOUSE");
doquestion("What is simplified code called?", "DRY");
Now this example doesn't handle cases of more than one right answer, but the function could be expanded if needed to be able to accomodate that also.
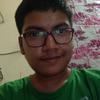
Abirbhav Goswami
15,450 PointsHe hasn't learnt about functions in this course yet. So the code he wrote is fine.
Jacob Cordeiro
2,796 PointsJacob Cordeiro
2,796 PointsThe US has 50 states, not 51.