Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial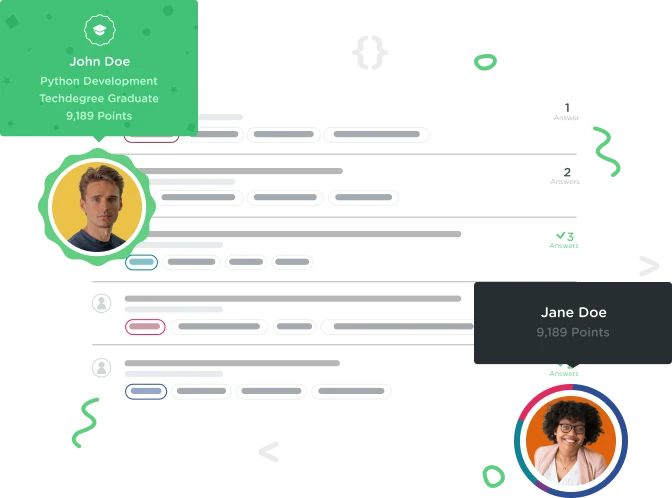
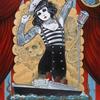
Saira Bottemuller
Courses Plus Student 1,749 PointsMy Star Trek Trivia game!
Here's what I came up with for the challenge. It seems to work just fine, although I've only been testing in Chrome. If I were to take it further I'd have to 1) switch to noncopyrighted material and 2) test functionality for multiple platforms... but here's my solution for the challenge. Constructive criticism and tips on how to be a better/more skilled coder are always welcome! (Some of the code is fairly messy, I think. I'd love some advice on better ways to format within JavaScript - how does one incorporate CSS into JS, at what point should a novice JSer go ahead and start learning JQuery---I've heard that can be helpful for formatting?---, etc).
Also, my apologies, I scoured the Markdown Cheatsheet and resource page, and couldn't find a way to make WordWrap turn on for code within my Forum posting.
<!DOCTYPE html>
<html>
<body>
<script type="text/javascript">
/*
The Ultimate Quiz Challenge
Challenge is from Team Treehouse;
Other than the challenge criteria issued by Team Treehouse, this program is all-original, and written by Saira Bottemuller on 03.26.15
This program is a Star Trek-themed quiz which asks the user a series of five questions about color, some easy, some a little tougher. The fifth question has a built-in failsafe so the user shouldn't be able to fail the test and earn a ZERO score ---- because Question 5 is built to accept any string, and since any user input comes in as a string, they will automatically earn at least ONE point in this quiz, even if they don't get anything else right, or enter a nonsensical answer for #5. IF YOU FIND a way to fail this test, please do let me know, sabottemuller@outlook.com , as I would love to play around with the program some more and see if I can get it to be totally failproof.
After #5 there is a bonus question, and it's about Star Trek, so I have written the program to shame them mercilessly if they get this one wrong - because it's easy, and there's just no room for Non-Trekkies in my world. :D
Once question 6 has been answered, a final score will be given, and prizes (or shame) duly awarded.
TO DO
============================================================
need to write a snippet of code that will:
---add an ALERT before 6th (Spock) question:
Now entering the BONUS ROUND - If you get this last question correct, you get 100 points! If you get it wrong......you automatically fail this quiz, and at life in general. But you might still get a performance-based consolation prize, so that's good right? Ready? Heeeere we go...:
---add a final score message to the player before the doling out of prizes and/or shame
---assign prizes appropriately:
0 correct = FAIL, no prize. might need glasses or to see a doctor. Just kidding, this test was built to be No-Fail.
1-2 correct = bronze crown
3-4 correct = silver crown
5 correct = gold crown
6 correct = platinum and diamond crown sprinkled with pixie dust, and a bonus prize
Bonus Prize = replica 1968 Star Trek : The Original Series phaser weapon....yeah not really, but it would be way cool right?! Howbout 100 bonus points instead? WOO!
*/
// Begin with an alert, to greet the user and give some info about the quiz:
alert("Hello! Welcome to my Star Trek: The Original Series Quiz! \n \t ************************************************** \n It may be of note that ALL PRIZES ARE IMAGINARY AND YOU WILL NOT ACTUALLY BE RECEIVING ANYTHING EXCEPT THE CHANCE TO IMPRESS MYSELF AND OTHERS WITH YOUR EXPERTISE. This is a casual game intended for fun and private, non-profit use only, and is in no way promisory, or affiliated with Star Trek, Gene Roddenberry, or any other applicable Trek-related party - company or individual.");
/*
===============================================================
The Alert segment has been tested and debugged. It works!
===============================================================
*/
//Defining the answer-counter variable as zero to start with:
var answersCorrect = 0
// Question 1:
var answer01 = prompt("1. What color is known as the shirt of doom, or kiss of death? Your answer choices are: Red, Yellow, Black, White, Gray, Green, Brown, Blue, Pink, or Neon-Clear.");
// Testing user's answer for correctness, and giving a score:
if ( answer01.toLowerCase() === "red" ) {
answer01 === true;
answersCorrect += 1;
alert("That's right! Okay kid, good job: you've gotten " + answersCorrect + " right so far - let's see what else you've got!");
} else {
answer01 === false;
alert("Sorry, that's not what we were looking for - but keep trying so you can improve your score! So far, you've gotten " + answersCorrect + " right.");
}
/*
===============================================================
The Question 1 segment has been tested and debugged. It works!
===============================================================
*/
// Question 2
var answer02= prompt("2. What color shirt (in various styles) does Captain James T. Kirk usually wear? Your answer choices are: Red, Yellow, Black, White, Gray, Green, Brown, Blue, Pink, or Neon-Clear.");
// Testing user's answer for correctness, and giving a score:
if ( answer02.toLowerCase() === "green" ) {
answer02 === true;
answersCorrect += 1;
alert("That's right! Good job, you might be a Trekster after all! You've gotten " + answersCorrect + " right so far.");
} else {
answer02 === false;
alert("Sorry, that's ...incorrect - but keep trying! Let's see if you can't improve on that score. So far, you've gotten " + answersCorrect + " right.");
}
/*
===============================================================
The Question 2 segment has been tested and debugged. It works!
===============================================================
*/
// Question 3
var answer03= prompt("3. And now a question of science and logic - dare you enter the realm of Mr. Spock? Okay....What 'color' is actually the combination of all colors in the visible spectrum, scientifically speaking? Your answer choices are: Red, Yellow, Black, White, Gray, Green, Brown, Blue, Pink, or Neon-Clear.");
// Testing user's answer for correctness, and giving a score:
if ( answer03.toLowerCase() === "white" ) {
answer03 === true;
answersCorrect += 1;
alert("Spock approves! Good job, you've gotten " + answersCorrect + " right so far!");
} else {
answer02 === false;
alert("I find fault with your logic, but keep trying - there may yet be hope for you. So far, you've gotten " + answersCorrect + " right.");
}
/*
===============================================================
The Question 3 segment has been tested and debugged. It works!
===============================================================
*/
// Question 4
var answer04= prompt("4. What color mainly comprises Uhura's uniform? Your answer choices are: Red, Yellow, Black, White, Gray, Green, Brown, Blue, Pink, or Neon-Clear.");
// Testing user's answer for correctness, and giving a score:
if ( answer04.toLowerCase() === "red" ) {
answer04 === true;
answersCorrect += 1;
alert("That's right, Nichelle looked lovely in red! Good job, you've gotten " + answersCorrect + " right so far!");
} else {
answer02 === false;
alert("Sorry, that's not what we were looking for - her trademark uniform was definitely red, but keep trying so you can improve your score! So far, you've gotten " + answersCorrect + " right.");
}
/*
===============================================================
The Question 4 segment has been tested and debugged. It works!
===============================================================
*/
// Question 5
var answer05= prompt("5. What color Federation uniform would YOU wear? Name any color you like, even if it's not on the list.");
// Testing user's answer for correctness, and giving a score:
if ( typeof answer05 === "string" ) {
answer05 === true;
answersCorrect += 1;
alert("That's pretty cool, a lot of other people have mentioned " + answer05 + " too! Good job, you've gotten " + answersCorrect + " right so far, including this one - because " + answer05 + " is a great choice!");
}
/*
===============================================================
The Question 5 segment has been tested and debugged. It works!
===============================================================
*/
//Declaring the prizeAwarded variable:
var prizeAwarded = alert("You won a prize! Let's see what's behind Door #3, Bob!");
/*
===============================================================
The prizeAwarded variable declaration segment has been tested and debugged. It works!
===============================================================
*/
// Handing out of the awards!
if (answersCorrect === 1 || answersCorrect === 2) {
prizeAwarded = "Well, at least you got " +answersCorrect + " right, so that's worth a prize. You may not have a LOT of Star Trek knowledge, but some is better than none. Please enjoy this bronze crown for your efforts. Keep it, sell it, melt it down and make something new - it's yours. Good job, and best of luck in the bonus round!";
alert(prizeAwarded);
} else if (answersCorrect === 3 || answersCorrect === 4) {
prizeAwarded = "So it looks like you got " + answersCorrect + " of those right, that's pretty decent! How about a silver crown for your efforts? Keep it and admire its sparkles, sell it, melt it down and make something new - it's yours. Good job! Best of luck in the Bonus Round.";
alert(prizeAwarded);
} else if (answersCorrect === 5) {
prizeAwarded = "WHOA! You got ALL of the answers correct?!!? That's DEFINITELY worth a prize. You have earned my respect and personal admiration...as well as a gold crown for being awesome! Keep it and admire its sparkles, sell it, since it's worth its weight in...well...gold... or melt it down and make something new - the gold crown is all yours. Excellent job! :) Best of luck in the bonus round!";
alert(prizeAwarded);
} else {
prizeAwarded = "Well, I THOUGHT I built this program to be failproof, but clearly you are a sneaky little ninja....and found a way to FAIL! Please email me at sabottemuller@outlook.com and let me know how you were able to get this alert! The more detail, the better, and I am perfectly open to polite and helpful critiques and advice - I am a novice, after all. Thanks for playing!";
alert(prizeAwarded);
}
/*
===============================================================
The handing out of the awards segment has been tested and debugged. It works!
===============================================================
*/
// The Bonus Question
alert("WOW! Okay, so you've gotten " + answersCorrect + " correct, out of five. Let's see if you can dominate in the bonus round! For the Grand Prize, answer the following question correctly:");
var bonusAnswer = prompt(" ---BONUS QUESTION:--- \n What was Scotty's role on the Enterprise during Season 1? \n ---SPELLING COUNTS,--- \n so pay attention! \n ------YOUR CHOICES ARE:------ \n Chief Security Officer \n Communications Officer \n Chief Engineering Officer \n First Officer \n Yeoman \n Helmsman \n Transporter Chief \n Science Officer \n Chief Operations Officer");
// Testing user's answer for correctness, and giving a score:
if ( bonusAnswer.toLowerCase() === "chief engineering officer" ) {
answersCorrect += 1;
alert("WHAT?!?! You got it RIGHT?!?! I am seriously impressed. Good job, Trekkie! Your final score over all was " + answersCorrect + " out of 6, and in addition to your regular prize, you will be taking home then grand prize... \n \n A REPLICA 1968 Star Trek: The Original Series Phaser weapon! Okay, not really, but don't you wish? Me too.");
} else {
alert("........OH well.. Not everybody can be amazing and know even the most *BASIC* of Star Trek trivia I guess........get to watching some Original Series, come back and try again! Better luck next time. Your final score wasn't TOO bad, at least you got " + answersCorrect + " right out of 6.");
}
</script>
</body>
</html>
3 Answers
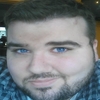
Marcus Parsons
15,719 PointsHey Saira,
First off, I love the wording you use in your little trivia game that incorporates pieces of Star Trek dialogue! Nicely done!
You do have some issues with your code, though, Saira. For example, you kept using this "===" operator in your statements, when I think you meant to set the answer to false. You have to use the single = sign to assign a value to a variable. === (or ==) are for comparisons only. Although, there is no reason to set them to false because you aren't using them again. And you kept referencing answer02 for some reason too lol.
//This:
answer04 === true;
//Should be:
answer04 = true;
//etc. for each answer
Otherwise, like I said, pretty awesome trivia game, Saira! Awesomesauce! :)
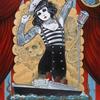
Saira Bottemuller
Courses Plus Student 1,749 PointsHaha oh my goodness THANK YOU! :D I figured there were some problems in that messy little code.
So when I need to set an answer to false, I don't need to use the === then, just the = to assign the variable a value of false, since you're GIVING it a value, not comparing it to anything. Okay, that's good and I'm glad you taught me that! Thank you :)
And as for answer02.... yeah I've got no excuse. That was lazy proofreading on my part lol :D
Thanks again for your help and upbeat feedback, I really appreciate it!
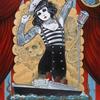
Saira Bottemuller
Courses Plus Student 1,749 PointsOut of curiousity, did you run it? Did it work for you, and did you find a way to make the "failsafe" I built into Question 5 work? I THINK I built it so the player can't achieve a zero score, but I'm curious if there's a way around it....
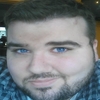
Marcus Parsons
15,719 PointsI totally did without trying to cheat and got 3 right including the freebie! haha
You did good with your question 5 if-statement but there is still one more sneaky thing that a user can do.....are you ready...are you SURE you're ready? Well, okay then...but seriously are you ready? Lol! They can hit the cancel button which returns a null
value. Since null
is not of type string, it will bypass the if-statement and not give the points.
To get around that just add an OR conditional to the if-statement:
if ( typeof answer05 === "string" || answer05 === null) {
answersCorrect += 1;
alert("That's pretty cool, a lot of other people have mentioned " + answer05 + " too! Good job, you've gotten " + answersCorrect + " right so far, including this one - because " + answer05 + " is a great choice!");
}
And then BAM! you got it! =]
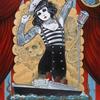
Saira Bottemuller
Courses Plus Student 1,749 PointsWOO! That is awesome. I knew there had to be a way around it, I'm glad you found it. I'll remember that for sure. Way good job on getting three right, you're Aces in my book. :D
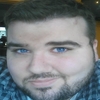
Marcus Parsons
15,719 PointsYes!!! You can't see it, but I totally just did the whole ending scene of The Breakfast Club!
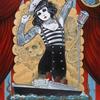
Saira Bottemuller
Courses Plus Student 1,749 PointsHAHAHAHA that's too funny, that movie is in my dvd player at home right now >_<
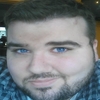
Marcus Parsons
15,719 PointsI'm peanut butter and jealous right now lol! I can watch it on Netflix, though haha I'm due for a rewatch here soon!