Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial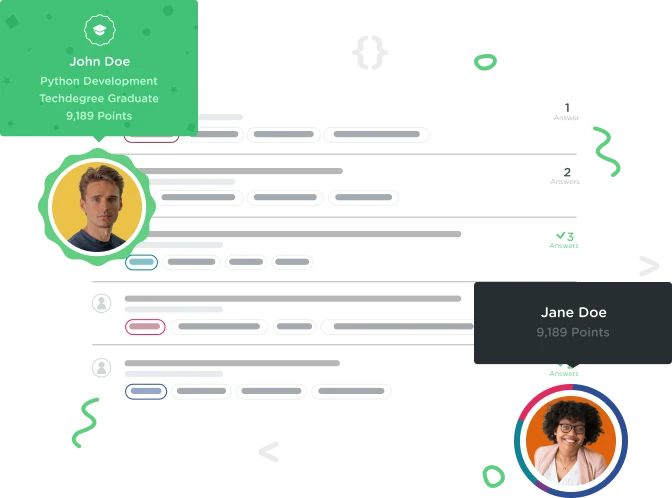

Blake Kazmierzak
2,491 PointsMy submission works, why is it being rejected?
My function has worked as expected with every example that I've thrown at it, why isn't it being accepted upon submission?
Instructions:
Let's write some functions to explore set math a bit more. We're going to be using this COURSES dict in all of the examples. Don't change it, though! So, first, write a function named covers that accepts a single parameter, a set of topics. Have the function return a list of courses from COURSES where the supplied set and the course's value (also a set) overlap. For example, covers({"Python"}) would return ["Python Basics"].
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(topics):
return [i[0] for i in COURSES.items() if topics == i[-1] & topics]
2 Answers
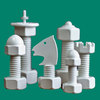
Steven Parker
231,275 PointsYou have a good idea for a compact solution, but there's an extra comparison constraining the results to exact subsets instead of any case of overlap. Remove that extra comparison and you'll have it:
def covers(topics):
return [i[0] for i in COURSES.items() if i[-1] & topics]
You can also simplify it a bit and eliminate the indexing by unpacking the tuples:
return [c for c,t in COURSES.items() if t & topics]

Robin Goyal
4,582 PointsIf you're still stuck on this, this is what I figured out after trying my hand at it. It's not exclusively looking for courses that meet all of the topics. So if your topics set was {"Python", "functions"}
, it shouldn't only return ["Python Basics"]. It should return ["Python Basics", "Python Basics", "Ruby Basics"]. Since "Python" and "functions" both appear in "Python Basics" course, you get the two entries of "Python Basics" in the list and "functions" only appears in the "Ruby Basics" course so you get only one entry of "Ruby Basics". It took me a while to get to this conclusion since the wording for this challenge and the example provided doesn't give enough info in my opinion.

Blake Kazmierzak
2,491 PointsOkay, thanks so much for the reply.
Blake Kazmierzak
2,491 PointsBlake Kazmierzak
2,491 PointsWow, thanks!