Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial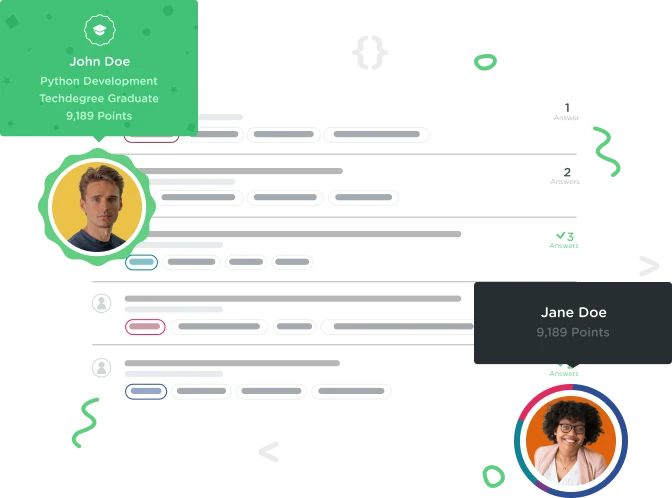

Jesse Ferguson
1,419 Pointsneed help again
have no idea what I am doing wrong
import random
start = 5
while start != 0:
start -= 1
random.randint(1, 99)
def even_odd(num):
if num % 2 == 1:
print('{} is even') .format(start)
else:
print('{} is odd').format(start)
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
3 Answers
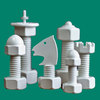
Steven Parker
230,995 PointsHere's a couple of hints:
- Don't modify the provided even_odd function.
- But you can use it in your code to test the chosen random number.
I'll bet you can get it now without a spoiler.

Joe Beltramo
Courses Plus Student 22,191 PointsYou almost got it.
The challenge is wanting you to use the function even_odd
in the while
loop without needing to modify it.
So, your while loop is a good start but instead of calling the random.randint(1, 99)
without a use for it, let's first place it into a variable that we can use at the first line of the loop
# You can use any name you want, I chose a variable named `rand`
rand = random.randint(1, 99)
Now you can use this new variable (rand) and pass it into the even_odd
function in an if statement
if even_odd(rand):
Inside the if statement you want to print out that the random number is even since the result of the if statement would need to be true to return an even number and false if the number is odd. In the code you provided you have almost done this. You are going to want to place the .format()
directly against the string inside the parenthesis of the print method
print('{} is even'.format(rand))
Then create an else statement and do the same thing above, with the exception of it saying odd instead of even.
Lastly, you will want to place your while loop below to even_odd
function as it must first be declared
At the end you should have something like this:
import random
start = 5
def even_odd(num):
# there is some stuff here that you didn't write or modify
while start != 0:
rand = random.randint(1, 99)
if even_odd(rand):
print('{} is even'.format(rand))
else:
print('{} is odd'.format(rand))
start -= 1
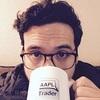
Nicholas Gaerlan
9,501 PointsThe program itself still runs top to bottom and outside to in (scope). So your main issue is that your while loop executes before "even_odd" is defined. You don't want to mess with the block of code within even_odd(), that's already done. It takes a number and returns a boolean. so... import random, initialize your start variable to five, leave even_odd alone, then BELOW all of that, write your while loop. It will look something like this. You have to imagine the flow of how this is executed from top to bottom. First you import random, then you have a variable, then you create a function, then you begin the while loop which has it's own flow... it makes a random number, then you start an if statement... that if/else loops, then finishes so then start -= 1 is called and the while loop finishes and re-evaluates until it is false. Hope that makes sense.
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
rnum = random.randint(1,99)
if even_odd(rnum):
print("{} is even".format(rnum))
else:
print("{} is odd".format(rnum))
start -= 1
Jesse Ferguson
1,419 PointsJesse Ferguson
1,419 PointsThanks