Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial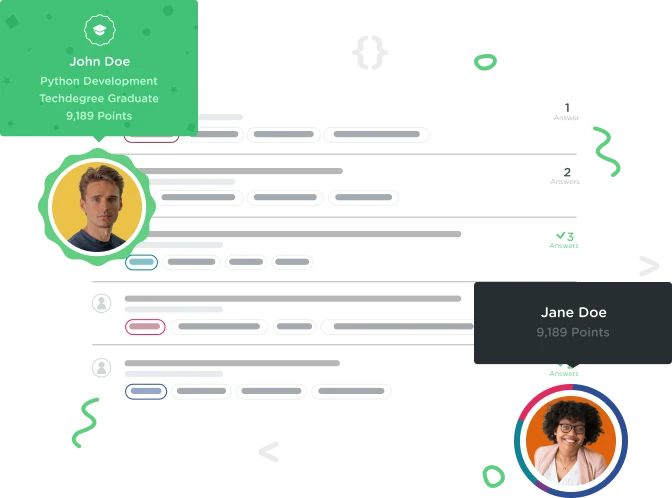
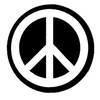
john larson
16,594 Pointsneed help cleaning up this code using a function
I got this code cleaned up quite a bit with this:
function print(output){
document.getElementById("display").innerHTML = output;
}
var one = prompt("How do you feel? " + questStr());
var two = prompt("and whats that like for you? " + questStr());
var three = prompt("bibity boo bot ...oh well " + questStr());
var message = "<p>If ever there was a "
+ one + ", then never ever could I "
+ two + ", on the other hand I never wanted to "
+ three + "</p>";
function questStr(){
var questions = 3;
var questionsLeft = "[ " + questions + " question(s) left]";
questions -= 1;
return questionsLeft;
}
It kinda works, but the counter for questions doesn't go down as it should. So I tried this:
function questStr() {
var questions = 3;
var questionsLeft = "[ " + questions + " question(s) left]";
function minus1() {
questions -=1;
return questions;
}
minus1();
return questionsLeft;
}
questStr();
I think it needs a callback or a closure...which is what I was trying to do, but I didn't get it to work. Insight is appreciated. Thank you.
2 Answers

Jae Min Kweon
4,695 PointsAs Joel said above, if you are trying to get the number of question to go down with each iteration you will need to set var questions =3 at global scope and then use it as an argument to your function. If you put it into the local scope for the function, then every time you call a function it will be reset to 3.
This would be my solution to the goal.
var questions = 3; //set the question counter outside the function to use as an argument.
function questStr (inputInt) {
return "[" + inputInt + " question(s) left]" //no need to declare a variable to use return and this is what you want to print out
}
The questStr() function that you are using in the top can be changed to questStr(questions --). What this will do is that it will execute the questStr() function using the set questions variable and then subsequently decrease the value of the questions variable by 1. In other words:
var questions = 3;
questStr(question --); //should return "[3 question(s) left]"
console.log(question) //should give 2 as a result
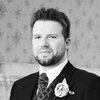
Joel Bardsley
31,249 PointsThe count isn't going down because you're setting var questions = 3 every time the questStr() function is called. In this case, the questions variable should be declared outside the function.
john larson
16,594 Pointsjohn larson
16,594 PointsJoel and Jae, thanks for helping. DRY coding has been my biggest downfall so far.