Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial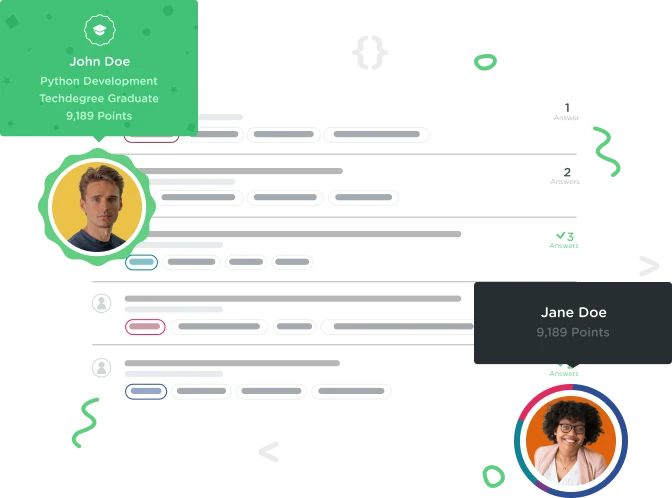

youssef moustahib
2,303 PointsNeed Help! I don't know whats wrong with my code..
?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(arg):
for word in arg.keys():
return len(arg)
def num_courses(arg):
count = 0
for word in arg.values():
count += 1
return count
1 Answer

Krishna Pratap Chouhan
15,203 PointsWe have to return the total number of courses for all the teachers. So sum up all the different courses that are being taught.
Lets see what you are doing:
def num_courses(arg):
count = 0
for word in arg.values():
count += 1
return count
here in your code "word" would be a list of courses so summing up all the list would tell you the number of teaches not the courses. You have to get the courses from each list.
Also, you are returning count inside the for loop so after the first iteration the function will return '1' no matter what.
Finally, there might be repetition in the courses. So two teachers might teach the same course(not sure if its definitely true but better if we cover this case as well).
See the code below for more details. . . .
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(arg):
for word in arg.keys():
return len(arg)
#The function should return the total number of courses for all of the teachers.
def num_courses(arg):
count = 0
courses_list = []
for name in arg.keys():
for course in arg[name]:
if course not in courses_list:
courses_list.append(course);
return len(courses_list);
youssef moustahib
2,303 Pointsyoussef moustahib
2,303 PointsThat one wasn't too bad, right? Let's try something a bit more challenging. Create a new function named num_courses that will receive the same dictionary as its only argument. The function should return the total number of courses for all of the teachers.