Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial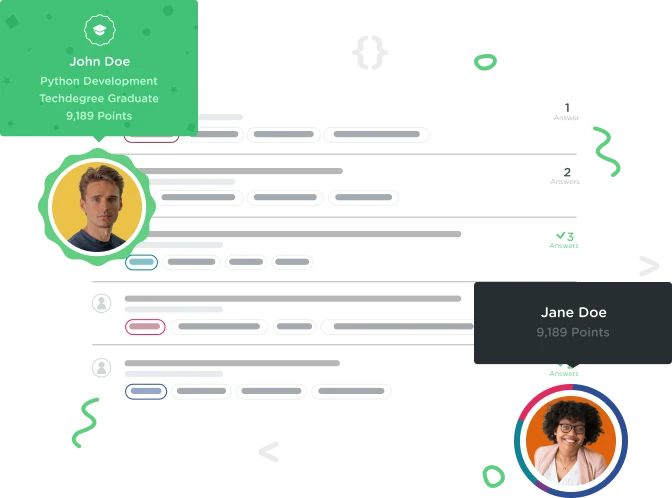
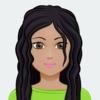
Jeeya M
16,839 PointsNeed Help in getting SwipeRefreshLayout working properly with Weather app
I really like how modern day Android apps (Gmail, Facebook, G+, etc.) have the functionality to swipe down to refresh the screen and data. I implemented that functionality as a challenge to the Weather app I created as part of Android Track. It works but for one major glitch. I have included links to my code on Github below.
What I did:
So here is what I did, I removed the progress and bar and Refresh icon from the top of the weather app's MainActivity
and replaced it with SwipeRefresh
functionality. I added a SwipeRefreshLayout
and associated code
<android.support.v4.widget.SwipeRefreshLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/swipeToRefresh"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:id="@+id/topRL4MainActivity"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/bg_gradient_blue"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:gravity="center"
tools:context=".ui.MainActivity">
onRefresh() method
Following is the code snippet from the onCreate()
method of the MainActivity.java
where we set OnRefreshListener
and handle the OnRefresh()
method.
mSwipeRefreshLayout = (SwipeRefreshLayout)findViewById(R.id.swipeToRefresh);
mSwipeRefreshLayout.setColorSchemeResources(R.color.Red,R.color.Orange,R.color.Blue,R.color.Green);
mSwipeRefreshLayout.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() {
@Override
public void onRefresh() {
Log.d(TAG,"************** SWIPE REFRESH EVENT TRIGGERED!!!!!");
getForecast();
});
What works and what does NOT work:
If I start the swipe from the upper or top part of my main screen and end my swipe about 30% of the top of the screen, the swipe event is NOT triggered and code does not go to my
onRefresh
method.But if I start the swipe from the middle of the screen and drag it down, it works perfectly and swipe event is triggered. And code reaches the onRefreshListener method and my call to reload the new JSON data from forecast.io is made successfully and screen is refreshed
Also, if I start the swipe from the top of the screen but end the swipe beyond middle of the screen, it also works and JSON call is made and screen is refreshed but the animation of swipe progress does not show properly.
Code
My whole working project is on GitHub at --> https://github.com/maanmehta/TreeHsBeginAndro_StormyWeather
activity_main.xml --> https://github.com/maanmehta/TreeHsBeginAndro_StormyWeather/blob/master/app/src/main/res/layout/activity_main.xml
MainActivity.java --> https://github.com/maanmehta/TreeHsBeginAndro_StormyWeather/blob/master/app/src/main/java/mun/treehsbeginandro_stormy/ui/MainActivity.java
I will highly appreciate if someone could check my code and if possible check out my project in latest Android Studio and get it to work and let me know what else I need to add to get this work. I have spent numerous hours on the net searching the problem and have tried lots of things but to no avail and hence I am asking here.
2 Answers
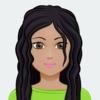
Jeeya M
16,839 PointsI had asked this question before but by mistake put it under ListAdapter topic, so no one answered. I have now solved the problem and I will post that below.
More findings
Also, I found out that it had nothing to do with swiping fro the middle of the screen or top of the screen. What I noticed was that in the middle of the screen I had a TextField
with huge font displaying temperature and when I started swipe from the TextField
, it worked perfectly. But it had nothing to do with TextField
but the fact that I had set an OnClickListener
to that TextField
. So, to verify, I added OnClickListener
to an image field and now if I swiped started on top of that image, swipe worked fine along with its animated circular progress animation.
Bug with SwipeRefreshLayout
So I think it is either a bug in SwipeRefreshLayout
where the swipe down functionality.
Therefore, to fix this bug, I set an OnClickListener
to the top most RelativeLayout
which was a child element to SwipeRefreshLayout and kept its onClick()
method just empty. This fixed the bug and now the screen could be dragged down from anywhere. Here is the code snippet from the onCreate
method of the MainActivity.java
// Bug fix for Swipe Refresh - Adding onClickListener to RelativeLayout inside the SwipeRefresh
mRL = (RelativeLayout) findViewById(R.id.topRL4MainActivity);
mRL.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Just empty method - do nothing
}
});
So, I am not sure if my solution is a workaround and if there is a better solution or is this a limitation with SwipeRefreshLayout
or that it has something to do with touch / click of the screen.
If someone else shed more light on this, I will appreciate it, though it is working as desired and I can now swipe down from anywhere on the screen and animated circular progress spinner spins fine as well and event is triggered every time.
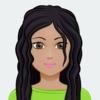
Jeeya M
16,839 PointsHi Ben Jakuben
Thanks for your reply. Yes, I would like you to read my post and my answer to it. In my answer I have explained that I have a workaround but wanted you to put your 2 cents if there is a better way than what I am doing or is this a constraint or bug in Android's SwipeRefreshLayout
Jeeya M
16,839 PointsJeeya M
16,839 PointsHi Ben Deitch , Ben Jakuben
Can you guys please review my this post and also my workaround/fix and comment. I will really appreciate it.
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherHey Tegh - are you still having trouble here? As you can probably tell, I'm trying to play catch-up on old Forum notifications!