Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial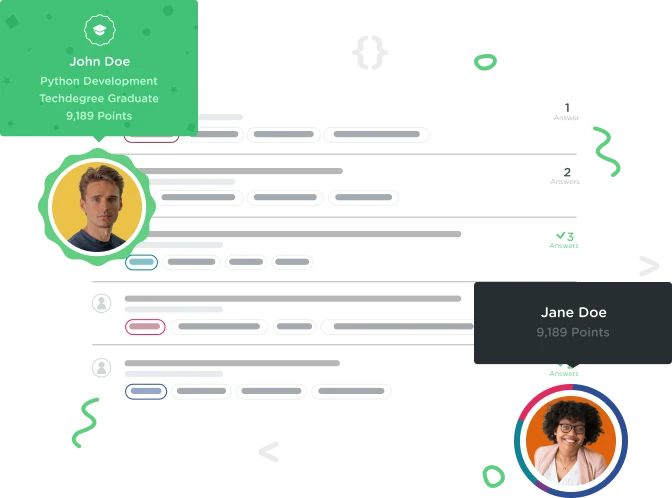
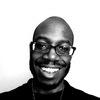
Micah Dunson
34,368 PointsNeed Help with Arrays
I need to create a FOR or WHILE loop to run thru the following:
var temperatures = [100,90,99,80,70,65,30,10];
This is my FOR loop, however it is incorrect and I don't know what I'm doing wrong:
for ( var i = 0, i > temperatures.length; i += 1 ) { console.log(temperatures[i]); }
var temperatures = [100,90,99,80,70,65,30,10];
for ( var i = 0, i > temperatures.length; i += 1 ) {
console.log(temperatures[i]);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
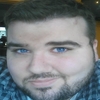
Marcus Parsons
15,719 PointsHi Micah,
Your error is that you have a comma inside of your for loop right after creating the variable i
and this should be a semicolon. Also, you need to use the < and not > because i is starting at 0 and 0 can't be greater than the length of the array meaning that your for loop would never execute.
var temperatures = [100,90,99,80,70,65,30,10];
//change comma to a semicolon
//also change > to <
for ( var i = 0; i < temperatures.length; i += 1 ) {
console.log(temperatures[i]);
}
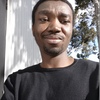
Andrews Gyamfi
Courses Plus Student 15,658 PointsHi Micah,
There are two issues with your for loop:
Firstly, you used the wrong punctuation just after your variable declaration inside the loop. Instead of a semi-colon you used a comma.
Secondly, your logic seem a bit flawed because you are increasing your counter variable (var i in this case) each time around the loop yet your condition states that the loop should run whilst var i is greater than the length of the temperatures array which would always be true (because you are increasing it from 0 to infinity). This would cause an infinite loop untill your computer runs out of memory:
var temperatures = [100,90,99,80,70,65,30,10];
for ( var i = 0, i > temperatures.length; i += 1 ) {
console.log(temperatures[i]);
}
Change your code to that shown below and it should work fine:
var temperatures = [100,90,99,80,70,65,30,10];
for ( var i = 0; i < temperatures.length; i += 1 ) {
console.log(temperatures[i]);
}
Cheers!
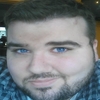
Marcus Parsons
15,719 PointsAndrews,
Your solution is right on the money. But just to address the logic behind why the for loop doesn't execute: the for loop would never execute because the condition is false and i
is of course not greater than the length of the array.
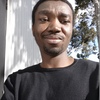
Andrews Gyamfi
Courses Plus Student 15,658 PointsHi Marcus,
That's perfectly right. The loop never gets executed as the first iteration would fail the conditional test. I was actually in a bit of a rush whilst replying to the question from my daytime workplace - haha! I call it "sneak coding". Thanks for picking that up though.
Cheers & happy coding!
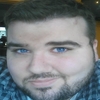
Marcus Parsons
15,719 PointsCheers and happy coding to you, as well! :)
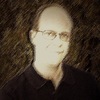
Jason Anders
Treehouse Moderator 145,860 PointsSimple fix... You have i+=1 in your 'for' condition. Change it to i++ and you'll be good to go. :)
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsWow... guess it's time to call it a night. LOL... I didn't catch that... and realize now that i+=1 IS right (just a different way of i++) ... haha.
Need to limit the amount of hours I stare at the computer screen, apparently! :/ Thanks Marcus.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsJason, you're trying buddy, and that's what counts! Keep on helping out and being an awesome student! :)
Micah Dunson
34,368 PointsMicah Dunson
34,368 PointsThank you I appreciate it. It's always the little thing lol
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsYou're very welcome, Micah! I know what you mean haha Happy Coding!