Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial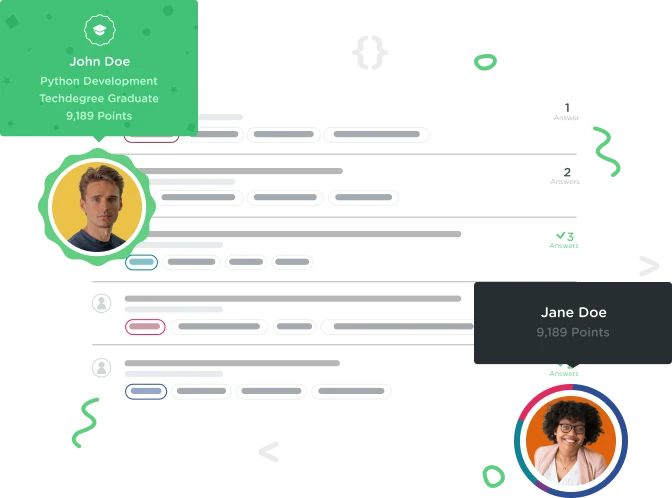

Francis Wanyonyi
6,768 PointsNeed help with question involving list methods, list formatting and exceptions.
Hi, My code for this question produces an error yet when I run it in my python environment, it works. I feel it could be a formatting problem rather than a code problem but I await advice from others. Please help. Thanks
def disemvowel(word):
final_word = list(word)
for letter in word:
try:
final_word.remove('a')
except ValueError:
pass
try:
final_word.remove('a'.upper())
except ValueError:
pass
try:
final_word.remove('e')
except ValueError:
pass
try:
final_word.remove('e'.upper())
except ValueError:
pass
try:
final_word.remove('i')
except ValueError:
pass
try:
final_word.remove('i'.upper())
except ValueError:
pass
try:
final_word.remove('o')
except ValueError:
pass
try:
final_word.remove('o'.upper())
except ValueError:
pass
try:
final_word.remove('u')
except ValueError:
pass
try:
final_word.remove('u'.upper())
except ValueError:
pass
return final_word
3 Answers

Charlie Gallentine
12,092 PointsThe challenge wants you to return a word with the vowels missing. There is nothing wrong with your code and it does return the correct letters, however, it returns them as a list of consonants. If you change your return statement to join the list into a single string, you will pass the challenge:
def disemvowel(word):
final_word = list(word)
for letter in word:
try:
final_word.remove('a')
except ValueError:
pass
try:
final_word.remove('a'.upper())
except ValueError:
pass
try:
final_word.remove('e')
except ValueError:
pass
try:
final_word.remove('e'.upper())
except ValueError:
pass
try:
final_word.remove('i')
except ValueError:
pass
try:
final_word.remove('i'.upper())
except ValueError:
pass
try:
final_word.remove('o')
except ValueError:
pass
try:
final_word.remove('o'.upper())
except ValueError:
pass
try:
final_word.remove('u')
except ValueError:
pass
try:
final_word.remove('u'.upper())
except ValueError:
pass
return "".join(final_word)

Francis Wanyonyi
6,768 PointsHaha, It worked. Thanks Charlie, I completely forgot to turn it back to a string.

Charlie Gallentine
12,092 PointsNo problem :) Happy coding!

Francis Wanyonyi
6,768 PointsHi Anyone, If you ever land here, here is the far more elegant code for the same challenge:
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u"]
final_word = list(word)
for letter in word:
if letter.lower() in vowels:
final_word.remove(letter)
return "".join(final_word)
courtesy of @andren